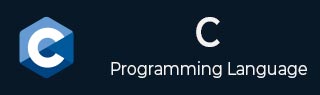
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
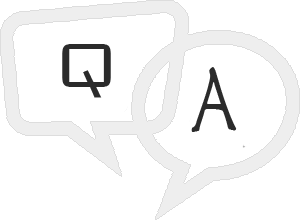
Q 1 - What is the output of the following code snippet?
#include<stdio.h> main() { int x = 5; if(x=5) { if(x=5) break; printf("Hello"); } printf("Hi"); }
Answer : A
Explanation
compile error, keyword break can appear only within loop/switch statement.
Q 2 - What is the output of the following program?
#include<stdio.h> main() { printf("\"); }
Answer : D
Explanation
Compile error, Format string of printf is not terminated.
Q 3 - Choose the application option for the following program?
#include<stdio.h> main() { int *p, **q; printf("%u\n", sizeof(p)); printf("%u\n", sizeof(q)); }
A - Both the printf() will print the same value
B - First printf() prints the value less than the second.
Answer : A
Explanation
Irrespective of any data type every type of pointer variable occupies same amount of memory.
Q 4 - What is the output of the following program?
#include<stdio.h> main() { char *s = "Hello, " "World!"; printf("%s", s); }
Answer : A
Explanation
Two immediate string constant are considered as single string constant.
Q 5 - What is the output of the following program?
#include<stdio.h> main() { struct student { int num = 10; }var; printf("%d", var.num); }
Answer : D
Explanation
Structure elements cannot be initialized
Q 6 - Choose the correct function which can return a reminder by dividing -10.0/3.0?
Answer : B
Explanation
In the C Programming Language, the fmod() function compute and returns the floating-point remainder when x is divided by y.
#include <math.h> #include <stdio.h> int main( void ) { double x = -10.0, y = 3.0, z; z = fmod( x, y ); printf( "The remainder of %.2f / %.2f is %f\n", w, x, z ); }
Q 7 - Which of the following operator can be used to access value at address stored in a pointer variable?
Answer : A
Explanation
Pointer operator,
* (Value Operator) = Gives Value stored at Particular address
& (Address Operator) = Gives Address of Variable
Q 8 - Which of the following variable cannot be used by switch-case statement?
Answer : C
Explanation
Switch Case only accepts integer values for case label, float values can’t be accepted in case of Switch Case.
#include<stdio.h> int main () { i = 1.8 switch ( i ) { case 1.6: printf ("Case 1.6"); break; case 1.7: printf ("Case 1.7"); break; case 1.8: printf ("Case 1.8"); break; default : printf ("Default Case "); } }
Q 9 - During preprocessing, the code “#include<stdio.h>” gets replaced by the contents of the file stdio.h.
B - During linking the code “#include<stdio.h>” replaces by stdio.h
C - During execution the code “#include<stdio.h>” replaces by stdio.h
D - During editing the code “#include<stdio.h>” replaces by stdio.h
Answer : A
Explanation
Preprocessing enlarges and boosts the C programming language by replacing preprocessing directive “#include<stdio.h>” with the content of the file stdio.h.
Q 10 - Which statement can print \n on the screen?
Answer : A
Explanation
Option A is the correct answer. In C programming language, "\n" is the escape sequence for printing a new line character. In printf("\\n"); statement, "\\" symbol will be printed as "\" and “n” will be known as a common symbol.
To Continue Learning Please Login