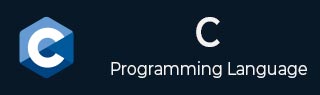
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Variable Length Arrays in C
A variable length array in C is also called a variable-sized or runtime-sized array. It is an array whose length is determined at the runtime rather than at the time of compiling the program. Its size depends on a value generated during the runtime of a program, usually received as an input from the user.
Usually, the array size is declared beforehand in the program as follows −
int arr[10];
The size of an array, once declared, remains fixed during the execution of a program and cannot be changed during its runtime. However, in case of a Variable Length Array (VLA), the compiler allocates the memory with automatic storage duration on the stack. The support for VLAs was added in the C99 standard.
Creating a Variable Length Array
The syntax for creating a variable length arrays is as follows −
void arr_init(int length){ int arr[length]; //code using arr; };
Example
The following example demonstrates how you can create a variable length array −
#include <stdio.h> int main(){ int i, j; int size; // variable to hold size of one-dimensional array printf("Enter the size of one-dimensional array: "); scanf("%d", &size); int arr[size]; for(i = 0; i < size; ++i){ printf("Enter a number: "); scanf("%d", &j); arr[i] = j; } for(i = 0; i < size; ++i) printf("a[%d]: %d\n", i, arr[i]); return 0; }
Output
When you run this code, it will ask you enter the size of the array. Notice that the length of the array is not fixed at the time of declaration. You define its size at runtime.
Enter the size of one-dimensional array: 5 Enter a number: 1 Enter a number: 5 Enter a number: 7 Enter a number: 8 Enter a number: 7 a[0]: 1 a[1]: 5 a[2]: 7 a[3]: 8 a[4]: 7
Two-dimensional Variable Length Arrays
We can also declare and use a two-dimensional variable length array.
Example 1
Take a look at the following example −
#include <stdio.h> int main(){ int i, j, x; int row, col; // number of rows & columns of two D array printf("Enter number of rows & columns of 2-D array:\n"); scanf("%d %d", &row, &col); int arr2D[row][col]; for(i = 0; i < row; ++i){ for(j = 0; j < col; ++j){ printf("Enter a number: "); scanf("%d", &x); arr2D[i][j] = x; } } for(i = 0; i < row; ++i){ printf("\n"); for(j = 0; j < col; ++j) printf("%d\t", arr2D[i][j]); } return 0; }
Output
Run the code and check its output −
Enter number of rows & columns of 2-D array: 2 3 Enter a number: 10 Enter a number: 20 Enter a number: 30 Enter a number: 40 Enter a number: 50 Enter a number: 60 10 20 30 40 50 60
Example 2
The following code declares a variable length one-dimensional array and populates it with incrementing numbers −
#include <stdio.h> int main(){ int n; printf("Enter the size of the array: \n"); scanf("%d", &n); int arr[n]; for(int i = 0; i < n; i++) arr[i] = i+1; printf("The array elements are: "); for(int i = 0; i < n; i++) printf("%d ", arr[i]); return 0; }
Output
Run the code and check its output −
Enter the size of the array: 5 The array elements are: 1 2 3 4 5 ....
Example 3
The following code fills the variable length array with randomly generated numbers using the functions srand() and rand() from the stdlib.h header file.
#include <stdio.h> #include <stdlib.h> #include <time.h> void oneDArray(int length, int a[length]); // function prototype void twoDArray(int row, int col, int a[row][col]); // function prototype int main(){ int i, j; // counter variable int size; // variable to hold size of one dimensional array int row, col; // number of rows & columns of two D array srand(time(NULL)); printf("Enter the size of one-dimensional array: "); scanf("%d", &size); printf("Enter the number of rows & columns of 2-D array:\n"); scanf("%d %d", &row, &col); // declaring arrays int arr[size]; // 2-D array int arr2D[row][col]; // one dimensional array for(i = 0; i < size; ++i){ arr[i] = rand() % 100 + 1; } // two dimensional array for(i = 0; i < row; ++i){ for(j = 0; j < col; ++j){ arr2D[i][j] = rand() % 100 + 1; } } // printing arrays printf("One-dimensional array:\n"); // oneDArray(size, arr); for(i = 0; i < size; ++i) printf("a[%d]: %d\n", i, arr[i]); printf("\nTwo-dimensional array:\n"); // twoDArray(row1, col1, arr2D); for(i = 0; i < row; ++i){ printf("\n"); for (j = 0; j < col; ++j) printf("%5d", arr2D[i][j]); } }
Output
Run the code and check its output −
Enter the size of one-dimensional array: 5 Enter the number of rows & columns of 2-D array: 4 4 One-dimensional array: a[0]: 95 a[1]: 93 a[2]: 4 a[3]: 52 a[4]: 68 Two-dimensional array: 92 19 79 23 56 21 44 98 8 22 89 54 93 1 63 38
Jagged Array
A jagged array is a collection of two or more arrays of similar data types of variable length. In C, the concept of jagged array is implemented with the help of pointers of arrays.
A jagged array is represented by the following figure −
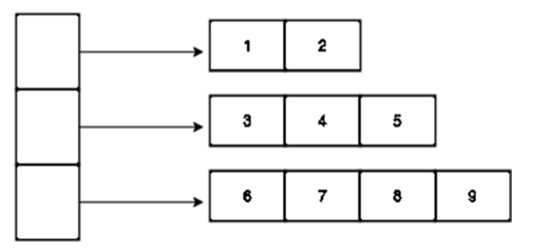
Example
In this program, we declare three one-dimensional arrays of different sizes and store their pointers in an array of pointers that acts as a jagged array.
#include <stdio.h> int main(){ int a[] = {1,2}; int b[] = {3,4,5}; int c[] = {6,7,8,9}; int l1 = sizeof(a)/sizeof(int), l2 = sizeof(b)/sizeof(int), l3 = sizeof(c)/sizeof(int); int *arr[] = {a,b,c}; int size[] = {l1, l2, l3}; int *ptr; int i, j, k = 0; for(i = 0; i < 3; i++){ ptr = arr[i]; for(j = 0; j < size[k]; j++){ printf("%d\t", *ptr); ptr++; } printf("\n"); k++; arr[i]++; } return 0; }
Output
When you run this code, it will produce the following output −
1 2 3 4 5 6 7 8 9
VLA is a fast and more straightforward option compared to heap allocation. VLAs are supported by most modern C compilers such as GCC, Clang etc. and are used by most compilers.
To Continue Learning Please Login