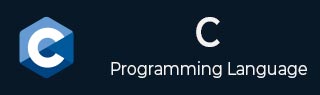
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Pointer to an Array in C
An array name is a constant pointer to the first element of the array. Therefore, in this declaration,
int balance[5];
balance is a pointer to &balance[0], which is the address of the first element of the array.
Example
In this code, we have a pointer ptr that points to the address of the first element of an integer array called balance.
#include <stdio.h> int main(){ int *ptr; int balance[5] = {1, 2, 3, 4, 5}; ptr = balance; printf("Pointer 'ptr' points to the address: %d", ptr); printf("\nAddress of the first element: %d", balance); printf("\nAddress of the first element: %d", &balance[0]); return 0; }
Output
In all the three cases, you get the same output −
Pointer 'ptr' points to the address: 647772240 Address of the first element: 647772240 Address of the first element: 647772240
If you fetch the value stored at the address that ptr points to, that is *ptr, then it will return 1.
Array Names as Constant Pointers
It is legal to use array names as constant pointers and vice versa. Therefore, *(balance + 4) is a legitimate way of accessing the data at balance[4].
Once you store the address of the first element in "ptr", you can access the array elements using *ptr, *(ptr + 1), *(ptr + 2), and so on.
Example
The following example demonstrates all the concepts discussed above −
#include <stdio.h> int main(){ /* an array with 5 elements */ double balance[5] = {1000.0, 2.0, 3.4, 17.0, 50.0}; double *ptr; int i; ptr = balance; /* output each array element's value */ printf("Array values using pointer: \n"); for(i = 0; i < 5; i++){ printf("*(ptr + %d): %f\n", i, *(ptr + i)); } printf("\nArray values using balance as address:\n"); for(i = 0; i < 5; i++){ printf("*(balance + %d): %f\n", i, *(balance + i)); } return 0; }
Output
When you run this code, it will produce the following output −
Array values using pointer: *(ptr + 0): 1000.000000 *(ptr + 1): 2.000000 *(ptr + 2): 3.400000 *(ptr + 3): 17.000000 *(ptr + 4): 50.000000 Array values using balance as address: *(balance + 0): 1000.000000 *(balance + 1): 2.000000 *(balance + 2): 3.400000 *(balance + 3): 17.000000 *(balance + 4): 50.000000
In the above example, ptr is a pointer that can store the address of a variable of double type. Once we have the address in ptr, *ptr will give us the value available at the address stored in ptr.
To Continue Learning Please Login