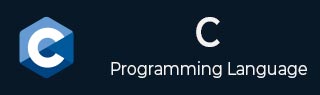
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Unions in C
A union is a special data type available in C that allows to store different data types in the same memory location. You can define a union with many members, but only one member can contain a value at any given time. Unions provide an efficient way of using the same memory location for multiple purpose.
All the members of a union share the same memory location. Therefore, if we need to use the same memory location for two or more members, then union is the best data type for that. The largest union member defines the size of the union.
Defining a Union
Union variables are created in same manner as structure variables. The keyword union is used to define unions in C language.
Here is the syntax of unions in C language −
union [union tag]{ member definition; member definition; ... member definition; } [one or more union variables];
The "union tag" is optional and each member definition is a normal variable definition, such as "int i;" or "float f;" or any other valid variable definition.
At the end of the union's definition, before the final semicolon, you can specify one or more union variables.
Difference between Structure and Union
Both structures and unions are composite data types in C programming. The most significant difference between a structure and a union is the way they store their data. A structure stores each member in separate memory locations, whereas a union stores all its members in the same memory location.
Here is a definition of union type called myunion −
union myunion{ int a; double b; char c; };
The definition of a union is similar to the definition of a structure. A definition of "struct type mystruct" with the same elements looks like this −
struct mystruct{ int a; double b; char c; };
The main difference between a struct and a union is the size of the variables. The compiler allocates the memory to a struct variable, to be able to store the values for all the elements. In mystruct, there are three elements − an int, a double, and char, requiring 13 bytes (4 + 8 + 1). Hence, sizeof(struct mystruct) returns 13.
On the other hand, for a union type variable, the compiler allocates a chunk of memory of the size enough to accommodate the element of the largest byte size. The myunion type has an int, a double and a char element. Out of the three elements, the size of the double variable is the largest, i.e., 8. Hence, sizeof(union myunion) returns 8.
Another point to take into consideration is that a union variable can hold the value of only one its elements. When you assign value to one element, the other elements are undefined. If you try to use the other elements, it will result in some garbage.
Example 1: Memory Occupied by a Union
In the code below, we define a union type called Data having three members i, f, and str. A variable of type Data can store an integer, a floating point number, or a string of characters. It means a single variable, i.e., the same memory location, can be used to store multiple types of data. You can use any built-in or user-defined data types inside a union, as per your requirement.
The memory occupied by a union will be large enough to hold the largest member of the union. For example, in the above example, Data will occupy 20 bytes of memory space because this is the maximum space which can be occupied by a character string.
The following example displays the total memory size occupied by the above union −
#include <stdio.h> #include <string.h> union Data{ int i; float f; char str[20]; }; int main(){ union Data data; printf("Memory occupied by Union Data: %d \n", sizeof(data)); return 0; }
Output
When you compile and execute the code, it will produce the following output −
Memory occupied by Union Data: 20
Example 2: Memory Occupied by a Structure
Now, let's create a structure with the same elements and check how much space it occupies in the memory.
#include <stdio.h> #include <string.h> struct Data{ int i; float f; char str[20]; }; int main(){ struct Data data; printf("Memory occupied by Struct Data: %d \n", sizeof(data)); return 0; }
Output
This stucture will occupy 28 bytes (4 + 4 + 20). Run the code and check its output −
Memory occupied by Struct Data: 28
Accessing the Union Members
To access any member of a union, we use the member access operator (.). The member access operator is coded as a period between the union variable name and the union member that we wish to access. You would use the keyword union to define variables of union type. The following example shows how to use unions in a program −
Example 1
#include <stdio.h> #include <string.h> union Data{ int i; float f; char str[20]; }; int main(){ union Data data; data.i = 10; data.f = 220.5; strcpy(data.str, "C Programming"); printf("data.i: %d \n", data.i); printf("data.f: %f \n", data.f); printf("data.str: %s \n", data.str); return 0; }
Output
When the above code is compiled and executed, it produces the following result −
data.i: 1917853763 data.f: 4122360580327794860452759994368.000000 data.str: C Programming
Here, we can see that the values of i and f (members of the union) show garbage values because the final value assigned to the variable has occupied the memory location and this is the reason that the value of str member is getting printed very well.
Example 2
Now let's look at the same example once again where we will use one variable at a time which is the main purpose of having unions −
#include <stdio.h> #include <string.h> union Data{ int i; float f; char str[20]; }; int main(){ union Data data; data.i = 10; printf("data.i: %d \n", data.i); data.f = 220.5; printf("data.f: %f \n", data.f); strcpy(data.str, "C Programming"); printf("data.str: %s \n", data.str); return 0; }
Output
When the above code is compiled and executed, it produces the following result −
data.i: 10 data.f: 220.500000 data.str: C Programming
Here, the values of all the Union members are getting printed very well because one member is being used at a time.
To Continue Learning Please Login