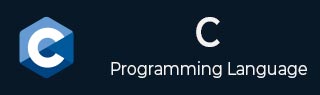
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Return a Pointer from a Function in C
In C programming, a function can be defined to have more than one argument, but it can return only one expression to the calling function.
A function can return a single value that may be any type of variable, either of a primary type (such as int, float, char, etc.), a pointer to a variable of primary or user−defined type, or a pointer to any variables.
Read this chapter to learn the different ways in which a function in a C program returns a pointer.
Return a Static Array from a Function in C
If a function has a local variable or a local array, then returning a pointer of the local variable is not acceptable because it points to a variable that no longer exists. Note that a local variable ceases to exist as soon as the scope of the function is over.
Example 1
The following example shows how you can use a static array inside the called function (arrfunction) and return its pointer back to the main() function.
#include <stdio.h> #include <math.h> float * arrfunction(int); int main(){ int x = 100, i; float *arr = arrfunction(x); printf("Square of %d: %f\n", x, *arr); printf("Cube of %d: %f\n", x, arr[1]); printf("Square root of %d: %f\n", x, arr[2]); return 0; } float *arrfunction(int x){ static float arr[3]; arr[0] = pow(x,2); arr[1] = pow(x, 3); arr[2] = pow(x, 0.5); return arr; }
Output
When you run this code, it will produce the following output −
Square of 100: 10000.000000 Cube of 100: 1000000.000000 Square root of 100: 10.000000
Example 2
Now consider the following function which will generate 10 random numbers. They are stored in a static array and return their pointer to the main() function. The array is then traversed in the main() function as follows −
#include <stdio.h> #include <time.h> #include <stdlib.h> /* function to generate and return random numbers */ int *getRandom() { static int r[10]; srand((unsigned)time(NULL)); /* set the seed */ for(int i = 0; i < 10; ++i){ r[i] = rand(); } return r; } int main(){ int *p; /* a pointer to an int */ p = getRandom(); for(int i = 0; i < 10; i++) { printf("*(p + %d): %d\n", i, *(p + i)); } return 0; }
Output
Run the code and check its output −
*(p + 0): 776161014 *(p + 1): 80783871 *(p + 2): 135562290 *(p + 3): 697080154 *(p + 4): 2064569097 *(p + 5): 1933176747 *(p + 6): 653917193 *(p + 7): 2142653666 *(p + 8): 1257588074 *(p + 9): 1257936184
Return a String from a Function in C
Using the same approach, you can pass and return a string to a function. A string in C is an array of char type. In the following example, we pass a string with a pointer, manipulate it inside the function, and return it to the main() function.
Example
Inside the called function, we use the malloc() function to allocate the memory. The passed string is concatenated with the local string before returning.
#include <stdio.h> #include <string.h> #include <stdlib.h> char *hellomsg(char *); int main(){ char *name = "TutorialsPoint"; char *arr = hellomsg(name); printf("%s\n", arr); return 0; } char *hellomsg(char *x){ char *arr = (char *)malloc(50*sizeof(char)); strcpy(arr, "Hello "); strcat(arr, x); return arr; }
Output
Run the code and check its output −
Hello TutorialsPoint
Return a Struct Pointer from a Function in C
The following example shows how you can return the pointer to a variable of struct type.
Here, the area() function has two call−by−value arguments. The main() function reads the length and breadth from the user and passes them to the area() function, which populates a struct variable and passes its reference (pointer) back to the main() function.
Example
Take a look at the program −
#include <stdio.h> #include <string.h> struct rectangle{ float len, brd; double area; }; struct rectangle * area(float x, float y); int main(){ struct rectangle *r; float x, y; x = 10.5, y = 20.5; r = area(x, y); printf("Length: %f \nBreadth: %f \nArea: %lf\n", r->len, r->brd, r->area); return 0; } struct rectangle * area(float x, float y){ double area = (double)(x*y); static struct rectangle r; r.len = x; r.brd = y; r.area = area; return &r; }
Output
When you run this code, it will produce the following output −
Length: 10.500000 Breadth: 20.500000 Area: 215.250000
To Continue Learning Please Login