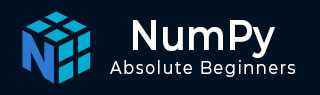
- NumPy Tutorial
- NumPy - Home
- NumPy - Introduction
- NumPy - Environment
- NumPy Arrays
- NumPy - Ndarray Object
- NumPy - Data Types
- NumPy Creating and Manipulating Arrays
- NumPy - Array Creation Routines
- NumPy - Array Manipulation
- NumPy - Array from Existing Data
- NumPy - Array From Numerical Ranges
- NumPy - Iterating Over Array
- NumPy - Reshaping Arrays
- NumPy - Concatenating Arrays
- NumPy - Stacking Arrays
- NumPy - Splitting Arrays
- NumPy - Flattening Arrays
- NumPy - Transposing Arrays
- NumPy Indexing & Slicing
- NumPy - Indexing & Slicing
- NumPy - Indexing
- NumPy - Slicing
- NumPy - Advanced Indexing
- NumPy - Fancy Indexing
- NumPy - Field Access
- NumPy - Slicing with Boolean Arrays
- NumPy Array Attributes & Operations
- NumPy - Array Attributes
- NumPy - Array Shape
- NumPy - Array Size
- NumPy - Array Strides
- NumPy - Array Itemsize
- NumPy - Broadcasting
- NumPy - Arithmetic Operations
- NumPy - Array Addition
- NumPy - Array Subtraction
- NumPy - Array Multiplication
- NumPy - Array Division
- NumPy Advanced Array Operations
- NumPy - Swapping Axes of Arrays
- NumPy - Byte Swapping
- NumPy - Copies & Views
- NumPy - Element-wise Array Comparisons
- NumPy - Filtering Arrays
- NumPy - Joining Arrays
- NumPy - Sort, Search & Counting Functions
- NumPy - Searching Arrays
- NumPy - Union of Arrays
- NumPy - Finding Unique Rows
- NumPy - Creating Datetime Arrays
- NumPy - Binary Operators
- NumPy - String Functions
- NumPy - Matrix Library
- NumPy - Linear Algebra
- NumPy - Matplotlib
- NumPy - Histogram Using Matplotlib
- NumPy Sorting and Advanced Manipulation
- NumPy - Sorting Arrays
- NumPy - Sorting along an axis
- NumPy - Sorting with Fancy Indexing
- NumPy - Structured Arrays
- NumPy - Creating Structured Arrays
- NumPy - Manipulating Structured Arrays
- NumPy - Record Arrays
- Numpy - Loading Arrays
- Numpy - Saving Arrays
- NumPy - Append Values to an Array
- NumPy - Swap Columns of Array
- NumPy - Insert Axes to an Array
- NumPy Handling Missing Data
- NumPy - Handling Missing Data
- NumPy - Identifying Missing Values
- NumPy - Removing Missing Data
- NumPy - Imputing Missing Data
- NumPy Performance Optimization
- NumPy - Performance Optimization with Arrays
- NumPy - Vectorization with Arrays
- NumPy - Memory Layout of Arrays
- Numpy Linear Algebra
- NumPy - Linear Algebra
- NumPy - Matrix Library
- NumPy - Matrix Addition
- NumPy - Matrix Subtraction
- NumPy - Matrix Multiplication
- NumPy - Element-wise Matrix Operations
- NumPy - Dot Product
- NumPy - Matrix Inversion
- NumPy - Determinant Calculation
- NumPy - Eigenvalues
- NumPy - Eigenvectors
- NumPy - Singular Value Decomposition
- NumPy - Solving Linear Equations
- NumPy - Matrix Norms
- NumPy Element-wise Matrix Operations
- NumPy - Sum
- NumPy - Mean
- NumPy - Median
- NumPy - Min
- NumPy - Max
- NumPy Set Operations
- NumPy - Unique Elements
- NumPy - Intersection
- NumPy - Union
- NumPy - Difference
- NumPy Random Number Generation
- NumPy - Random Generator
- NumPy - Permutations & Shuffling
- NumPy - Uniform distribution
- NumPy - Normal distribution
- NumPy - Binomial distribution
- NumPy - Poisson distribution
- NumPy - Exponential distribution
- NumPy - Rayleigh Distribution
- NumPy - Logistic Distribution
- NumPy - Pareto Distribution
- NumPy - Visualize Distributions With Sea born
- NumPy - Matplotlib
- NumPy - Multinomial Distribution
- NumPy - Chi Square Distribution
- NumPy - Zipf Distribution
- NumPy File Input & Output
- NumPy - I/O with NumPy
- NumPy - Reading Data from Files
- NumPy - Writing Data to Files
- NumPy - File Formats Supported
- NumPy Mathematical Functions
- NumPy - Mathematical Functions
- NumPy - Trigonometric functions
- NumPy - Exponential Functions
- NumPy - Logarithmic Functions
- NumPy - Hyperbolic functions
- NumPy - Rounding functions
- NumPy Fourier Transforms
- NumPy - Discrete Fourier Transform (DFT)
- NumPy - Fast Fourier Transform (FFT)
- NumPy - Inverse Fourier Transform
- NumPy - Fourier Series and Transforms
- NumPy - Signal Processing Applications
- NumPy - Convolution
- NumPy Polynomials
- NumPy - Polynomial Representation
- NumPy - Polynomial Operations
- NumPy - Finding Roots of Polynomials
- NumPy - Evaluating Polynomials
- NumPy Statistics
- NumPy - Statistical Functions
- NumPy - Descriptive Statistics
- NumPy Datetime
- NumPy - Basics of Date and Time
- NumPy - Representing Date & Time
- NumPy - Date & Time Arithmetic
- NumPy - Indexing with Datetime
- NumPy - Time Zone Handling
- NumPy - Time Series Analysis
- NumPy - Working with Time Deltas
- NumPy - Handling Leap Seconds
- NumPy - Vectorized Operations with Datetimes
- NumPy ufunc
- NumPy - ufunc Introduction
- NumPy - Creating Universal Functions (ufunc)
- NumPy - Arithmetic Universal Function (ufunc)
- NumPy - Rounding Decimal ufunc
- NumPy - Logarithmic Universal Function (ufunc)
- NumPy - Summation Universal Function (ufunc)
- NumPy - Product Universal Function (ufunc)
- NumPy - Difference Universal Function (ufunc)
- NumPy - Finding LCM with ufunc
- NumPy - ufunc Finding GCD
- NumPy - ufunc Trigonometric
- NumPy - Hyperbolic ufunc
- NumPy - Set Operations ufunc
- NumPy Useful Resources
- NumPy Compiler
- NumPy - Quick Guide
- NumPy - Cheatsheet
- NumPy - Useful Resources
- NumPy - Discussion
NumPy - Matrix Subtraction
What is Matrix Subtraction?
Matrix subtraction is an operation where two matrices of the same size are subtracted element-wise. In matrix subtraction, each element of one matrix is subtracted by the corresponding element of the other matrix.
Just like matrix addition, for matrix subtraction to be valid, both matrices must have the same dimensions (the same number of rows and columns).
If you have two matrices, say A and B, of the same size, then their difference C is defined as:
C = A - B
Where,
Cij = Aij - Bij
In simple terms, the element in the ith row and jth column of matrix C is the result of subtracting the corresponding elements in matrices A and B.
Example of Matrix Subtraction
Consider the following two matrices:
A = [[5, 8], [7, 10]] B = [[2, 4], [3, 6]]
The difference C = A - B will be calculated as:
C = [[5-2, 8-4], [7-3, 10-6]] = [[3, 4], [4, 4]]
So, the result of subtracting matrix B from matrix A gives us matrix C:
C = [[3, 4], [4, 4]]
Matrix Subtraction in NumPy
In NumPy, matrix subtraction is done using the - operator or using the numpy.subtract() function. NumPy handles matrix operations like subtraction element-wise, which makes mathematical computations fast and easy.
Following are the key points to remember while performing matrix subtraction −
- Matrix Dimensions: For matrix subtraction to be valid, both matrices must have the same dimensions (same number of rows and columns).
- Element-wise Operations: NumPy automatically handles element-wise operations, making it easy to subtract matrices using the - operator or the numpy.subtract() function.
- Flexible Arrays: NumPy arrays are flexible and can handle matrices of different sizes as long as they are compatible in dimensions.
Creating Matrices in NumPy
Before performing matrix subtraction, let us first create two matrices in NumPy using the np.array() function. These matrices should have the same dimensions for subtraction to work as shown below −
import numpy as np # Creating two 2x2 matrices A = np.array([[5, 8], [7, 10]]) B = np.array([[2, 4], [3, 6]]) # Print the matrices print("Matrix A:") print(A) print("\nMatrix B:") print(B)
Following is the output obtained −
Matrix A: [[ 5 8] [ 7 10]] Matrix B: [[2 4] [3 6]]
Matrix Subtraction Using the - Operator
The simplest way to subtract two matrices in NumPy is by using the - operator. This operator automatically performs element-wise subtraction of the two matrices.
Example
In the following example, we are subtracting two matrices "A" and "B" using the "-" operator −
import numpy as np # Creating two 2x2 matrices A = np.array([[5, 8], [7, 10]]) B = np.array([[2, 4], [3, 6]]) # Subtracting two matrices using the - operator C = A - B # Print the result print("Matrix C (A - B):") print(C)
The output obtained is as shown below −
Matrix C (A - B): [[3 4] [4 4]]
Using the numpy.subtract() Function
Alternatively, you can subtract matrices using the numpy.subtract() function. This function works the same way as the - operator. It takes two matrices (or arrays) as inputs and returns their difference.
Example
In this example, we are subtracting two matrices "A" and "B" using the "numpy.subtract()" function −
import numpy as np # Creating two 2x2 matrices A = np.array([[5, 8], [7, 10]]) B = np.array([[2, 4], [3, 6]]) # Subtracting two matrices using numpy.subtract() function C = np.subtract(A, B) # Print the result print("Matrix C (A - B using numpy.subtract()):") print(C)
We get the output as shown below −
Matrix C (A - B using numpy.subtract()): [[3 4] [4 4]]
Broadcasting in Matrix Subtraction
Matrix subtraction, like matrix addition, also relies on the concept of broadcasting in NumPy. Broadcasting allows NumPy to perform element-wise operations on arrays of different shapes.
However, for matrix subtraction, both matrices must have the same shape. Broadcasting is not applicable here if the matrices don't match in size.
Example
To give you a sense of how broadcasting works (though not directly applicable to matrix subtraction), here's an example of subtracting a scalar from a matrix −
import numpy as np # Create a 2x2 matrix A = np.array([[10, 12], [15, 18]]) # Subtract a scalar from the matrix using broadcasting B = A - 5 # Print the result print("Matrix A - 5:") print(B)
The result produced is as follows −
Matrix A - 5: [[ 5 7] [10 13]]
Error Handling in Matrix Subtraction
If you try to subtract matrices with different shapes, NumPy will raise an error. It is important to ensure the matrices have the same dimensions before attempting subtraction.
Example
Following is an example of mismatch dimension in NumPy while performing matrix subtraction −
import numpy as np # Create two matrices with different shapes A = np.array([[1, 2], [3, 4]]) B = np.array([[5, 6, 7]]) # Try to subtract them (this will raise an error) C = A - B print(C)
After executing the above code, we get the following output −
Traceback (most recent call last): File "/home/cg/root/6734345c5507a/main.py", line 8, in <module> C = A - B ValueError: operands could not be broadcast together with shapes (2,2) (1,3)
Applications of Matrix Subtraction
- Image Processing: In image processing, matrices represent images as pixel values. Matrix subtraction is used to modify images, such as subtracting certain values to enhance or reduce brightness.
- Data Analysis: In data science, matrices are used to represent datasets. Subtracting matrices can be useful for removing unwanted noise or for normalizing datasets.
- Scientific Computing: In physics and engineering, matrix subtraction is often used to calculate differences between matrices representing different variables or states of a system.
- Computer Graphics: In computer graphics, matrix subtraction helps in transformations and adjustments of shapes or objects in 3D space.