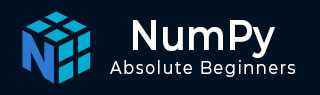
- NumPy Tutorial
- NumPy - Home
- NumPy - Introduction
- NumPy - Environment
- NumPy Arrays
- NumPy - Ndarray Object
- NumPy - Data Types
- NumPy Creating and Manipulating Arrays
- NumPy - Array Creation Routines
- NumPy - Array Manipulation
- NumPy - Array from Existing Data
- NumPy - Array From Numerical Ranges
- NumPy - Iterating Over Array
- NumPy - Reshaping Arrays
- NumPy - Concatenating Arrays
- NumPy - Stacking Arrays
- NumPy - Splitting Arrays
- NumPy - Flattening Arrays
- NumPy - Transposing Arrays
- NumPy Indexing & Slicing
- NumPy - Indexing & Slicing
- NumPy - Indexing
- NumPy - Slicing
- NumPy - Advanced Indexing
- NumPy - Fancy Indexing
- NumPy - Field Access
- NumPy - Slicing with Boolean Arrays
- NumPy Array Attributes & Operations
- NumPy - Array Attributes
- NumPy - Array Shape
- NumPy - Array Size
- NumPy - Array Strides
- NumPy - Array Itemsize
- NumPy - Broadcasting
- NumPy - Arithmetic Operations
- NumPy - Array Addition
- NumPy - Array Subtraction
- NumPy - Array Multiplication
- NumPy - Array Division
- NumPy Advanced Array Operations
- NumPy - Swapping Axes of Arrays
- NumPy - Byte Swapping
- NumPy - Copies & Views
- NumPy - Element-wise Array Comparisons
- NumPy - Filtering Arrays
- NumPy - Joining Arrays
- NumPy - Sort, Search & Counting Functions
- NumPy - Searching Arrays
- NumPy - Union of Arrays
- NumPy - Finding Unique Rows
- NumPy - Creating Datetime Arrays
- NumPy - Binary Operators
- NumPy - String Functions
- NumPy - Matrix Library
- NumPy - Linear Algebra
- NumPy - Matplotlib
- NumPy - Histogram Using Matplotlib
- NumPy Sorting and Advanced Manipulation
- NumPy - Sorting Arrays
- NumPy - Sorting along an axis
- NumPy - Sorting with Fancy Indexing
- NumPy - Structured Arrays
- NumPy - Creating Structured Arrays
- NumPy - Manipulating Structured Arrays
- NumPy - Record Arrays
- Numpy - Loading Arrays
- Numpy - Saving Arrays
- NumPy - Append Values to an Array
- NumPy - Swap Columns of Array
- NumPy - Insert Axes to an Array
- NumPy Handling Missing Data
- NumPy - Handling Missing Data
- NumPy - Identifying Missing Values
- NumPy - Removing Missing Data
- NumPy - Imputing Missing Data
- NumPy Performance Optimization
- NumPy - Performance Optimization with Arrays
- NumPy - Vectorization with Arrays
- NumPy - Memory Layout of Arrays
- Numpy Linear Algebra
- NumPy - Linear Algebra
- NumPy - Matrix Library
- NumPy - Matrix Addition
- NumPy - Matrix Subtraction
- NumPy - Matrix Multiplication
- NumPy - Element-wise Matrix Operations
- NumPy - Dot Product
- NumPy - Matrix Inversion
- NumPy - Determinant Calculation
- NumPy - Eigenvalues
- NumPy - Eigenvectors
- NumPy - Singular Value Decomposition
- NumPy - Solving Linear Equations
- NumPy - Matrix Norms
- NumPy Element-wise Matrix Operations
- NumPy - Sum
- NumPy - Mean
- NumPy - Median
- NumPy - Min
- NumPy - Max
- NumPy Set Operations
- NumPy - Unique Elements
- NumPy - Intersection
- NumPy - Union
- NumPy - Difference
- NumPy Random Number Generation
- NumPy - Random Generator
- NumPy - Permutations & Shuffling
- NumPy - Uniform distribution
- NumPy - Normal distribution
- NumPy - Binomial distribution
- NumPy - Poisson distribution
- NumPy - Exponential distribution
- NumPy - Rayleigh Distribution
- NumPy - Logistic Distribution
- NumPy - Pareto Distribution
- NumPy - Visualize Distributions With Sea born
- NumPy - Matplotlib
- NumPy - Multinomial Distribution
- NumPy - Chi Square Distribution
- NumPy - Zipf Distribution
- NumPy File Input & Output
- NumPy - I/O with NumPy
- NumPy - Reading Data from Files
- NumPy - Writing Data to Files
- NumPy - File Formats Supported
- NumPy Mathematical Functions
- NumPy - Mathematical Functions
- NumPy - Trigonometric functions
- NumPy - Exponential Functions
- NumPy - Logarithmic Functions
- NumPy - Hyperbolic functions
- NumPy - Rounding functions
- NumPy Fourier Transforms
- NumPy - Discrete Fourier Transform (DFT)
- NumPy - Fast Fourier Transform (FFT)
- NumPy - Inverse Fourier Transform
- NumPy - Fourier Series and Transforms
- NumPy - Signal Processing Applications
- NumPy - Convolution
- NumPy Polynomials
- NumPy - Polynomial Representation
- NumPy - Polynomial Operations
- NumPy - Finding Roots of Polynomials
- NumPy - Evaluating Polynomials
- NumPy Statistics
- NumPy - Statistical Functions
- NumPy - Descriptive Statistics
- NumPy Datetime
- NumPy - Basics of Date and Time
- NumPy - Representing Date & Time
- NumPy - Date & Time Arithmetic
- NumPy - Indexing with Datetime
- NumPy - Time Zone Handling
- NumPy - Time Series Analysis
- NumPy - Working with Time Deltas
- NumPy - Handling Leap Seconds
- NumPy - Vectorized Operations with Datetimes
- NumPy ufunc
- NumPy - ufunc Introduction
- NumPy - Creating Universal Functions (ufunc)
- NumPy - Arithmetic Universal Function (ufunc)
- NumPy - Rounding Decimal ufunc
- NumPy - Logarithmic Universal Function (ufunc)
- NumPy - Summation Universal Function (ufunc)
- NumPy - Product Universal Function (ufunc)
- NumPy - Difference Universal Function (ufunc)
- NumPy - Finding LCM with ufunc
- NumPy - ufunc Finding GCD
- NumPy - ufunc Trigonometric
- NumPy - Hyperbolic ufunc
- NumPy - Set Operations ufunc
- NumPy Useful Resources
- NumPy Compiler
- NumPy - Quick Guide
- NumPy - Cheatsheet
- NumPy - Useful Resources
- NumPy - Discussion
NumPy - Field access
NumPy Field Access
Field access in NumPy refers to retrieving or modifying specific elements within a structured array based on their field names. It allows you to work with individual attributes or properties of each record in the array.
Structured arrays in NumPy enable you to define arrays with records that contain multiple fields, each with its own data type. Fields in a structured array can be accessed individually, allowing for manipulation of data.
Accessing Individual Fields by Name
Structured arrays in NumPy allow you to assign names to different fields within each element. This naming convention makes it easy to access specific fields directly using those names.
In NumPy, when working with structured arrays, accessing individual fields allows you to interact with specific components or attributes of each element in the array. This is important when dealing with arrays that contain multiple types of data.
Example
In the following example, we are accessing the 'name' field of a structured array to extract and retrieve all the names from the array −
import numpy as np # Define a structured array with fields 'name' and 'age' dtype = [('name', 'U10'), ('age', 'i4')] data = [('Alice', 25), ('Bob', 30)] structured_array = np.array(data, dtype=dtype) # Access the 'name' field names = structured_array['name'] print("Names:", names)
Following is the output obtained −
Names: ['Alice' 'Bob']
Field Access in Multi-dimensional Arrays
To access specific fields in a multi-dimensional structured array, you can use indexing techniques similar to those used in 1D and 2D arrays but applied across multiple dimensions.
A multi-dimensional structured array is an array where each element is itself a structured array, and these elements are organized in multiple dimensions (e.g., 2D, 3D arrays). Each element in the array can have multiple fields, similar to a table where each row is a record with several attributes.
Example
In the example below, we are accessing the 'name' field from the first layer of a 3D structured array −
import numpy as np # Define a 3D structured array dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [[[('Alice', 25, 5.5), ('Bob', 30, 6.0)], [('Charlie', 35, 5.8), ('David', 40, 6.2)]], [[('Eve', 28, 5.7), ('Frank', 33, 6.1)], [('Grace', 29, 5.6), ('Hank', 32, 6.3)]]] structured_array_3d = np.array(data, dtype=dtype) # Access the 'name' field from the first layer names_layer_0 = structured_array_3d[0]['name'] print("Names in the first layer:\n", names_layer_0)
Following is the output of the above code −
Names in the first layer: [['Alice' 'Bob'] ['Charlie' 'David']]
Accessing Fields in Specific Slices
Accessing fields in specific slices means retrieving values from particular subsets or ranges of data within a structured array.
When you slice a single dimension of a structured array, you can then access specific fields from the resulting slice. To access fields from slices involving multiple dimensions, you need to apply slicing across dimensions and then select fields from the resulting sub-array.
Example: Slicing 1D and Accessing Fields
In the following example, we are slicing a structured array to obtain a subset of rows, specifically rows 1 and 2. After slicing, we access and retrieve the 'name' and 'age' fields from this subset −
import numpy as np # Define a structured array with fields 'name' and 'age' dtype = [('name', 'U10'), ('age', 'i4')] data = [('Alice', 25), ('Bob', 30), ('Charlie', 35), ('David', 40)] structured_array = np.array(data, dtype=dtype) # Slice the array to get a subset of rows sliced_array = structured_array[1:3] # Gets rows 1 and 2 # Access the 'name' field from the sliced array names = sliced_array['name'] # Access the 'age' field from the sliced array ages = sliced_array['age'] print("Sliced names:", names) print("Sliced ages:", ages)
The output obtained is as shown below −
Sliced names: ['Bob' 'Charlie'] Sliced ages: [30 35]
Example: Slicing 2D and Accessing Fields
Here, we are slicing a 2D structured array to extract a subset of rows and columns. We then access and retrieve the 'name' and 'age' fields from this sliced portion of the array −
import numpy as np # Define a 2D array with structured data dtype = [('name', 'U10'), ('age', 'i4')] data = [[('Alice', 25), ('Bob', 30)], [('Charlie', 35), ('David', 40)]] structured_array = np.array(data, dtype=dtype).view(np.recarray) # Slice the array to get a subset of rows and columns sliced_array = structured_array[0:2, 0:2] # Gets all rows and columns # Access the 'name' field from the sliced array names = sliced_array['name'] # Access the 'age' field from the sliced array ages = sliced_array['age'] print("Sliced names:", names) print("Sliced ages:", ages)
After executing the above code, we get the following output −
Sliced names: [['Alice' 'Bob'] ['Charlie' 'David']] Sliced ages: [[25 30] [35 40]]
Accessing Multiple Fields Simultaneously
Accessing multiple fields simultaneously means retrieving data from more than one field in a structured array at the same time, allowing you to work with a subset of fields together.
To access multiple fields simultaneously in NumPy, you can use the following ways −
- Accessing with a List of Field Names: You can specify multiple field names in a list to get a structured array containing only those fields.
- Using Field Indexing with Structured Arrays: If you need to access fields by their indices, you can select them using their positions.
Example
In the example below, we are accessing different fields of a structured array by specifying field names or indices, and printing the results. We retrieve specific fields such as 'name' and 'age', as well as all fields simultaneously −
import numpy as np # Define a structured array with fields 'name', 'age', and 'height' dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [('Alice', 25, 5.5), ('Bob', 30, 6.0), ('Charlie', 35, 5.8)] structured_array = np.array(data, dtype=dtype) # 1. Accessing multiple fields with a list of field names selected_fields = structured_array[['name', 'age']] print("Selected fields (name and age):") print(selected_fields) # 2. Accessing fields by index names = structured_array['name'] ages = structured_array['age'] heights = structured_array['height'] print("\nNames:", names) print("Ages:", ages) print("Heights:", heights) # Accessing all fields simultaneously all_fields = structured_array[['name', 'age', 'height']] print("\nAll fields:") print(all_fields)
The result produced is as follows −
Selected fields (name and age): [('Alice', 25) ('Bob', 30) ('Charlie', 35)] Names: ['Alice' 'Bob' 'Charlie'] Ages: [25 30 35] Heights: [5.5 6. 5.8] All fields: [('Alice', 25, 5.5) ('Bob', 30, 6. ) ('Charlie', 35, 5.8)]
Combining Field Access with Boolean Indexing
Combining field access with Boolean indexing means retrieving specific fields from a structured array based on a condition or filter applied to the array.
Boolean indexing allows you to select elements of an array that satisfy a given condition. By applying a boolean mask (an array of boolean values) to a structured array, you can filter the array based on conditions applied to one or more fields.
Example
In the following example, we are using a boolean mask to filter a structured array based on the 'age' field. We then select and print the 'name' and 'height' fields of the entries where 'age' is greater than 30 −
import numpy as np # Define a structured array dtype = [('name', 'U10'), ('age', 'i4'), ('height', 'f4')] data = [('Alice', 25, 5.5), ('Bob', 30, 6.0), ('Charlie', 35, 5.8), ('David', 40, 6.2)] structured_array = np.array(data, dtype=dtype) # Create a boolean mask for filtering based on 'age' mask = structured_array['age'] > 30 # Apply boolean indexing and select 'name' and 'height' fields filtered_fields = structured_array[mask][['name', 'height']] print("Filtered Fields (name and height) where age > 30:\n", filtered_fields)
We get the output as shown below −
Filtered Fields (name and height) where age > 30:[('Charlie', 5.8) ('David', 6.2)]