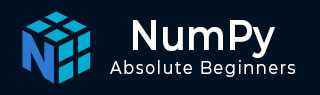
- NumPy Tutorial
- NumPy - Home
- NumPy - Introduction
- NumPy - Environment
- NumPy Arrays
- NumPy - Ndarray Object
- NumPy - Data Types
- NumPy Creating and Manipulating Arrays
- NumPy - Array Creation Routines
- NumPy - Array Manipulation
- NumPy - Array from Existing Data
- NumPy - Array From Numerical Ranges
- NumPy - Iterating Over Array
- NumPy - Reshaping Arrays
- NumPy - Concatenating Arrays
- NumPy - Stacking Arrays
- NumPy - Splitting Arrays
- NumPy - Flattening Arrays
- NumPy - Transposing Arrays
- NumPy Indexing & Slicing
- NumPy - Indexing & Slicing
- NumPy - Indexing
- NumPy - Slicing
- NumPy - Advanced Indexing
- NumPy - Fancy Indexing
- NumPy - Field Access
- NumPy - Slicing with Boolean Arrays
- NumPy Array Attributes & Operations
- NumPy - Array Attributes
- NumPy - Array Shape
- NumPy - Array Size
- NumPy - Array Strides
- NumPy - Array Itemsize
- NumPy - Broadcasting
- NumPy - Arithmetic Operations
- NumPy - Array Addition
- NumPy - Array Subtraction
- NumPy - Array Multiplication
- NumPy - Array Division
- NumPy Advanced Array Operations
- NumPy - Swapping Axes of Arrays
- NumPy - Byte Swapping
- NumPy - Copies & Views
- NumPy - Element-wise Array Comparisons
- NumPy - Filtering Arrays
- NumPy - Joining Arrays
- NumPy - Sort, Search & Counting Functions
- NumPy - Searching Arrays
- NumPy - Union of Arrays
- NumPy - Finding Unique Rows
- NumPy - Creating Datetime Arrays
- NumPy - Binary Operators
- NumPy - String Functions
- NumPy - Matrix Library
- NumPy - Linear Algebra
- NumPy - Matplotlib
- NumPy - Histogram Using Matplotlib
- NumPy Sorting and Advanced Manipulation
- NumPy - Sorting Arrays
- NumPy - Sorting along an axis
- NumPy - Sorting with Fancy Indexing
- NumPy - Structured Arrays
- NumPy - Creating Structured Arrays
- NumPy - Manipulating Structured Arrays
- NumPy - Record Arrays
- Numpy - Loading Arrays
- Numpy - Saving Arrays
- NumPy - Append Values to an Array
- NumPy - Swap Columns of Array
- NumPy - Insert Axes to an Array
- NumPy Handling Missing Data
- NumPy - Handling Missing Data
- NumPy - Identifying Missing Values
- NumPy - Removing Missing Data
- NumPy - Imputing Missing Data
- NumPy Performance Optimization
- NumPy - Performance Optimization with Arrays
- NumPy - Vectorization with Arrays
- NumPy - Memory Layout of Arrays
- Numpy Linear Algebra
- NumPy - Linear Algebra
- NumPy - Matrix Library
- NumPy - Matrix Addition
- NumPy - Matrix Subtraction
- NumPy - Matrix Multiplication
- NumPy - Element-wise Matrix Operations
- NumPy - Dot Product
- NumPy - Matrix Inversion
- NumPy - Determinant Calculation
- NumPy - Eigenvalues
- NumPy - Eigenvectors
- NumPy - Singular Value Decomposition
- NumPy - Solving Linear Equations
- NumPy - Matrix Norms
- NumPy Element-wise Matrix Operations
- NumPy - Sum
- NumPy - Mean
- NumPy - Median
- NumPy - Min
- NumPy - Max
- NumPy Set Operations
- NumPy - Unique Elements
- NumPy - Intersection
- NumPy - Union
- NumPy - Difference
- NumPy Random Number Generation
- NumPy - Random Generator
- NumPy - Permutations & Shuffling
- NumPy - Uniform distribution
- NumPy - Normal distribution
- NumPy - Binomial distribution
- NumPy - Poisson distribution
- NumPy - Exponential distribution
- NumPy - Rayleigh Distribution
- NumPy - Logistic Distribution
- NumPy - Pareto Distribution
- NumPy - Visualize Distributions With Sea born
- NumPy - Matplotlib
- NumPy - Multinomial Distribution
- NumPy - Chi Square Distribution
- NumPy - Zipf Distribution
- NumPy File Input & Output
- NumPy - I/O with NumPy
- NumPy - Reading Data from Files
- NumPy - Writing Data to Files
- NumPy - File Formats Supported
- NumPy Mathematical Functions
- NumPy - Mathematical Functions
- NumPy - Trigonometric functions
- NumPy - Exponential Functions
- NumPy - Logarithmic Functions
- NumPy - Hyperbolic functions
- NumPy - Rounding functions
- NumPy Fourier Transforms
- NumPy - Discrete Fourier Transform (DFT)
- NumPy - Fast Fourier Transform (FFT)
- NumPy - Inverse Fourier Transform
- NumPy - Fourier Series and Transforms
- NumPy - Signal Processing Applications
- NumPy - Convolution
- NumPy Polynomials
- NumPy - Polynomial Representation
- NumPy - Polynomial Operations
- NumPy - Finding Roots of Polynomials
- NumPy - Evaluating Polynomials
- NumPy Statistics
- NumPy - Statistical Functions
- NumPy - Descriptive Statistics
- NumPy Datetime
- NumPy - Basics of Date and Time
- NumPy - Representing Date & Time
- NumPy - Date & Time Arithmetic
- NumPy - Indexing with Datetime
- NumPy - Time Zone Handling
- NumPy - Time Series Analysis
- NumPy - Working with Time Deltas
- NumPy - Handling Leap Seconds
- NumPy - Vectorized Operations with Datetimes
- NumPy ufunc
- NumPy - ufunc Introduction
- NumPy - Creating Universal Functions (ufunc)
- NumPy - Arithmetic Universal Function (ufunc)
- NumPy - Rounding Decimal ufunc
- NumPy - Logarithmic Universal Function (ufunc)
- NumPy - Summation Universal Function (ufunc)
- NumPy - Product Universal Function (ufunc)
- NumPy - Difference Universal Function (ufunc)
- NumPy - Finding LCM with ufunc
- NumPy - ufunc Finding GCD
- NumPy - ufunc Trigonometric
- NumPy - Hyperbolic ufunc
- NumPy - Set Operations ufunc
- NumPy Useful Resources
- NumPy Compiler
- NumPy - Quick Guide
- NumPy - Cheatsheet
- NumPy - Useful Resources
- NumPy - Discussion
NumPy - Logarithmic Universal Function (ufunc)
Logarithmic Universal Function (ufunc)
A logarithmic universal function (ufunc) in NumPy is a function that applies the logarithm operation to each element in an array. This means it computes the logarithm of every individual value in the array, either using the natural logarithm (base e) or a different base such as base-2 logarithm or base-10 logarithm.
NumPy provides several logarithmic ufuncs, such as numpy.log(), numpy.log2(), numpy.log10().
NumPy Natural Logarithm
The numpy.log() function is used to compute the natural logarithm (base-e) of each element in an array. This function is commonly used in mathematical computations involving exponential growth or decay.
Example
In the following example, we use the numpy.log() function to calculate the natural logarithm of each element in an array −
import numpy as np # Define an array a = np.array([1, 2, 3, 4, 5]) # Compute natural logarithm result = np.log(a) print(result)
Following is the output obtained −
[0. 0.69314718 1.09861229 1.38629436 1.60943791]
NumPy Base-10 Logarithm
The numpy.log10() function is used to compute the base-10 logarithm of each element in an array. This function is useful in scientific fields such as chemistry and physics, where logarithmic scales are often used.
Example
In the following example, we use the numpy.log10() function to calculate the base-10 logarithm of each element in an array −
import numpy as np # Define an array a = np.array([1, 10, 100, 1000, 10000]) # Compute base-10 logarithm result = np.log10(a) print(result)
This will produce the following result −
[0. 1. 2. 3. 4.]
NumPy Base-2 Logarithm
The numpy.log2() function is used to compute the base-2 logarithm of each element in an array. This function is often used in computer science and information theory.
Example
In the following example, we use the numpy.log2() function to calculate the base-2 logarithm of each element in an array −
import numpy as np # Define an array a = np.array([1, 2, 4, 8, 16]) # Compute base-2 logarithm result = np.log2(a) print(result)
Following is the output of the above code −
[0. 1. 2. 3. 4.]
NumPy Logarithm with Any Base
While NumPy provides specific functions for base-e, base-10, and base-2 logarithms, you can compute logarithms with any base by using the numpy.log() function in combination with the change of base formula.
Example
In the following example, we calculate the base-3 logarithm of each element in an array using the change of base formula −
import numpy as np # Define an array a = np.array([1, 3, 9, 27, 81]) # Compute base-3 logarithm result = np.log(a) / np.log(3) print(result)
The result produced is as follows −
[0. 1. 2. 3. 4.]
NumPy Logarithm of 1 plus Input
The numpy.log1p() function is used to compute the natural logarithm of 1 plus the input array elements. This function provides more accurate results for small input values compared to directly using numpy.log(1 + x) function.
Example
In the following example, we use the numpy.log1p() function to calculate the natural logarithm of 1 plus each element in an array −
import numpy as np # Define an array a = np.array([0.1, 0.2, 0.3, 0.4, 0.5]) # Compute natural logarithm of 1 plus the input array elements result = np.log1p(a) print(result)
Following is the output obtained −
[0.09531018 0.18232156 0.26236426 0.33647224 0.40546511]