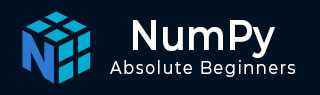
- NumPy Tutorial
- NumPy - Home
- NumPy - Introduction
- NumPy - Environment
- NumPy - Ndarray Object
- NumPy - Data Types
- NumPy - Array Attributes
- NumPy - Array Creation Routines
- NumPy - Array from Existing Data
- Array From Numerical Ranges
- NumPy - Indexing & Slicing
- NumPy - Advanced Indexing
- NumPy - Broadcasting
- NumPy - Iterating Over Array
- NumPy - Array Manipulation
- NumPy - Binary Operators
- NumPy - String Functions
- NumPy - Mathematical Functions
- NumPy - Arithmetic Operations
- NumPy - Statistical Functions
- Sort, Search & Counting Functions
- NumPy - Byte Swapping
- NumPy - Copies & Views
- NumPy - Matrix Library
- NumPy - Linear Algebra
- NumPy - Matplotlib
- NumPy - Histogram Using Matplotlib
- NumPy - I/O with NumPy
- NumPy Useful Resources
- NumPy Compiler
- NumPy - Quick Guide
- NumPy - Useful Resources
- NumPy - Discussion
numpy.char.strip()
This function returns a copy of array with elements stripped of the specified characters leading and/or trailing in it.
import numpy as np print np.char.strip('ashok arora','a') print np.char.strip(['arora','admin','java'],'a')
Here is its output −
shok aror ['ror' 'dmin' 'jav']
numpy_string_functions.htm
Advertisements