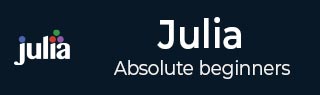
- Julia Tutorial
- Julia - Home
- Julia - Overview
- Julia - Environment Setup
- Julia - Basic Syntax
- Julia - Arrays
- Julia - Tuples
- Integers & Floating-Point Numbers
- Julia - Rational & Complex Numbers
- Julia - Basic Operators
- Basic Mathematical Functions
- Julia - Strings
- Julia - Functions
- Julia - Flow Control
- Julia - Dictionaries & Sets
- Julia - Date & Time
- Julia - Files I/O
- Julia - Metaprogramming
- Julia - Plotting
- Julia - Data Frames
- Working with Datasets
- Julia - Modules and Packages
- Working with Graphics
- Julia - Networking
- Julia - Databases
- Julia Useful Resources
- Julia - Quick Guide
- Julia - Useful Resources
- Julia - Discussion
Julia - Arrays
An Array is an ordered set of elements which are often specified with squared brackets having comma-separated items. We can create arrays that are −
Full or empty
Hold values of different types
Restricted to values of a specific type
In Julia, arrays are actually mutable type collections which are used for lists, vectors, tables, and matrices. That is why the values of arrays in Julia can be modified with the use of certain pre-defined keywords. With the help of push! command you can add new element in array. Similarly, with the help of splice! function you can add elements in an array at a specified index.
Creating Simple 1D Arrays
Following is the example showing how we can create a simple 1D array −
julia> arr = [1,2,3] 3-element Array{Int64,1}: 1 2 3
The above example shows that we have created a 1D array with 3 elements each of which is a 64-bit integer. This 1D array is bound to the variable arr.
Uninitialized array
We can also specify the type and the dimension of an array by using the below syntax −
Array{type}(dims)
Following is an example of uninitialized array −
julia> array = Array{Int64}(undef, 3) 3-element Array{Int64,1}: 0 0 0 julia> array = Array{Int64}(undef, 3, 3, 3) 3×3×3 Array{Int64,3}: [:, :, 1] = 8 372354944 328904752 3 331059280 162819664 32 339708912 1 [:, :, 2] = 331213072 3 331355760 1 328841776 331355984 -1 328841680 2 [:, :, 3] = 1 0 339709232 164231472 328841872 347296224 328841968 339709152 16842753
Here we placed the type in curly braces and the dimensions in parentheses. We use undef which means that particular array has not been initialized to any known value and thats why we got random numbers in the output.
Arrays of anything
Julia gives us the freedom to create arrays with elements of different types. Let us see the example below in which we are going to create array of an odd mixture — numbers, strings, functions, constants −
julia> [1, "TutorialsPoint.com", 5.5, tan, pi] 5-element Array{Any,1}: 1 "TutorialsPoint.com" 5.5 tan (generic function with 12 methods) π = 3.1415926535897...
Empty Arrays
Just like creating an array of specific type, we can also create empty arrays in Julia. The example is given below −
julia> A = Int64[] Int64[] julia> A = String[] String[]
Creating 2D arrays & matrices
Leave out the comma between elements and you will be getting 2D arrays in Julia. Below is the example given for single row, multi-column array −
julia> [1 2 3 4 5 6 7 8 9 10] 1×10 Array{Int64,2}: 1 2 3 4 5 6 7 8 9 10
Here, 1×10 is the first row of this array.
To add another row, just add a semicolon(;). Let us check the below example −
julia> [1 2 3 4 5 ; 6 7 8 9 10] 2×5 Array{Int64,2}: 1 2 3 4 5 6 7 8 9 10
Here, it becomes 2×5 array.
Creating arrays using range objects
We can create arrays using range objects in the following ways −
Collect() function
First useful function to create an array using range objects is collect(). With the help of colon(:) and collect() function, we can create an array using range objects as follows −
julia> collect(1:5) 5-element Array{Int64,1}: 1 2 3 4 5
We can also create arrays with floating point range objects −
julia> collect(1.5:5.5) 5-element Array{Float64,1}: 1.5 2.5 3.5 4.5 5.5
Let us see a three-piece version of a range object with the help of which you can specify a step size other than 1.
The syntax for the same is given below −start:step:stop.
Below is an example to build an array with elements that go from 0 to 50 in steps of 5 −
julia> collect(0:5:50) 11-element Array{Int64,1}: 0 5 10 15 20 25 30 35 40 45 50
ellipsis(…) or splat operator
Instead of using collect() function, we can also use splat operator or ellipsis(…) after the last element. Following is an example −
julia> [0:10...] 11-element Array{Int64,1}: 0 1 2 3 4 5 6 7 8 9 10
range() function
Range() is another useful function to create an array with range objects. It goes from start value to end value by taking a specific step value.
For example, let us see an example to go from 1 to 150 in exactly 15 steps −
julia> range(1, length=15, stop=150) 1.0:10.642857142857142:150.0 Or you can use range to take 10 steps from 1, stopping at or before 150: julia> range(1, stop=150, step=10) 1:10:141
We can use range() with collect() to build an array as follows −
julia> collect(range(1, length=15, stop=150)) 15-element Array{Float64,1}: 1.0 11.642857142857142 22.285714285714285 32.92857142857143 43.57142857142857 54.214285714285715 64.85714285714286 75.5 86.14285714285714 96.78571428571429 107.42857142857143 118.07142857142857 128.71428571428572 139.35714285714286 150.0
Creating arrays using comprehensions and generators
Another useful way to create an array is to use comprehensions. In this way, we can create array where each element can produce using a small computation. For example, we can create an array of 10 elements as follows −
julia> [n^2 for n in 1:10] 10-element Array{Int64,1}: 1 4 9 16 25 36 49 64 81 100
We can easily create a 2-D array also as follows −
julia> [n*m for n in 1:10, m in 1:10] 10×10 Array{Int64,2}: 1 2 3 4 5 6 7 8 9 10 2 4 6 8 10 12 14 16 18 20 3 6 9 12 15 18 21 24 27 30 4 8 12 16 20 24 28 32 36 40 5 10 15 20 25 30 35 40 45 50 6 12 18 24 30 36 42 48 54 60 7 14 21 28 35 42 49 56 63 70 8 16 24 32 40 48 56 64 72 80 9 18 27 36 45 54 63 72 81 90 10 20 30 40 50 60 70 80 90 100
Similar to comprehension, we can use generator expressions to create an array −
julia> collect(n^2 for n in 1:5) 5-element Array{Int64,1}: 1 4 9 16 25
Generator expressions do not build an array to first hold the values rather they generate the values when needed. Hence they are more useful than comprehensions.
Populating an Array
Following are the functions with the help of which you can create and fill arrays with specific contents −
zeros (m, n)
This function will create matrix of zeros with m number of rows and n number of columns. The example is given below −
julia> zeros(4,5) 4×5 Array{Float64,2}: 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0
We can also specify the type of zeros as follows −
julia> zeros(Int64,4,5) 4×5 Array{Int64,2}: 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
ones (m, n)
This function will create matrix of ones with m number of rows and n number of columns. The example is given below −
julia> ones(4,5) 4×5 Array{Float64,2}: 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0 1.0
rand (m, n)
As the name suggests, this function will create matrix of random numbers with m number of rows and n number of columns. The example is given below −
julia> rand(4,5) 4×5 Array{Float64,2}: 0.514061 0.888862 0.197132 0.721092 0.899983 0.503034 0.81519 0.061025 0.279143 0.204272 0.687983 0.883176 0.653474 0.659005 0.970319 0.20116 0.349378 0.470409 0.000273225 0.83694
randn(m, n)
As the name suggests, this function will create m*n matrix of normally distributed random numbers with mean=0 and standard deviation(SD)=1.
julia> randn(4,5) 4×5 Array{Float64,2}: -0.190909 -1.18673 2.17422 0.811674 1.32414 0.837096 -0.0326669 -2.03179 0.100863 0.409234 -1.24511 -0.917098 -0.995239 0.820814 1.60817 -1.00931 -0.804208 0.343079 0.0771786 0.361685
fill()
This function is used to fill an array with a specific value. More specifically, it will create an array of repeating duplicate value.
julia> fill(100,5) 5-element Array{Int64,1}: 100 100 100 100 100 julia> fill("tutorialspoint.com",3,3) 3×3 Array{String,2}: "tutorialspoint.com" "tutorialspoint.com" "tutorialspoint.com" "tutorialspoint.com" "tutorialspoint.com" "tutorialspoint.com" "tutorialspoint.com" "tutorialspoint.com" "tutorialspoint.com"
fill!()
It is similar to fill() function but the sign of exclamation (!) is an indication or warning that the content of an existing array is going to be changed. The example is given below:
julia> ABC = ones(5) 5-element Array{Float64,1}: 1.0 1.0 1.0 1.0 1.0 julia> fill!(ABC,100) 5-element Array{Float64,1}: 100.0 100.0 100.0 100.0 100.0 julia> ABC 5-element Array{Float64,1}: 100.0 100.0 100.0 100.0 100.0
Array Constructor
The function Array(), we have studied earlier, can build array of a specific type as follows −
julia> Array{Int64}(undef, 5) 5-element Array{Int64,1}: 294967297 8589934593 8589934594 8589934594 0
As we can see from the output that this array is uninitialized. The odd-looking numbers are memories’ old content.
Arrays of arrays
Following example demonstrates creating arrays of arrays −
julia> ABC = Array[[3,4],[5,6]] 2-element Array{Array,1}: [3, 4] [5, 6]
It can also be created with the help of Array constructor as follows −
julia> Array[1:5,6:10] 2-element Array{Array,1}: [1, 2, 3, 4, 5] [6, 7, 8, 9, 10]
Copying arrays
Suppose you have an array and want to create another array with similar dimensions, then you can use similar() function as follows −
julia> A = collect(1:5); Here we have hide the values with the help of semicolon(;) julia> B = similar(A) 5-element Array{Int64,1}: 164998448 234899984 383606096 164557488 396984416
Here the dimension of array A are copied but not values.
Matrix Operations
As we know that a two-dimensional (2D) array can be used as a matrix so all the functions that are available for working on arrays can also be used as matrices. The condition is that the dimensions and contents should permit. If you want to type a matrix, use spaces to make rows and semicolon(;) to separate the rows as follows −
julia> [2 3 ; 4 5] 2×2 Array{Int64,2}: 2 3 4 5
Following is an example to create an array of arrays (as we did earlier) by placing two arrays next to each other −
julia> Array[[3,4],[5,6]] 2-element Array{Array,1}: [3, 4] [5, 6]
Below we can see what happens when we omit the comma and place columns next to each other −
julia> [[3,4] [5,6]] 2×2 Array{Int64,2}: 3 5 4 6
Accessing the contents of arrays
In Julia, to access the contents/particular element of an array, you need to write the name of the array with the element number in square bracket.
Below is an example of 1-D array −
julia> arr = [5,10,15,20,25,30,35,40,45,50] 10-element Array{Int64,1}: 5 10 15 20 25 30 35 40 45 50 julia> arr[4] 20
In some programming languages, the last element of an array is referred to as -1. However, in Julia, it is referred to as end. You can find the last element of an array as follows −
julia> arr[end] 50
And the second last element as follows −
julia> arr[end-1] 45
To access more than one element at a time, we can also provide a bunch of index numbers as shown below −
julia> arr[[2,5,6]] 3-element Array{Int64,1}: 10 25 30
We can access array elements even by providing true and false −
julia> arr[[true, false, true, true,true, false, false, true, true, false]] 6-element Array{Int64,1}: 5 15 20 25 40 45
Now let us access the elements of 2-D.
julia> arr2 = [10 11 12; 13 14 15; 16 17 18] 3×3 Array{Int64,2}: 10 11 12 13 14 15 16 17 18 julia> arr2[1] 10
The below command will give 13 not 11 as we were expecting.
julia> arr2[2] 13
To access row1, column2 element, we need to use the command below −
julia> arr2[1,2] 11
Similarly, for row1 and column3 element, we have to use the below command −
julia> arr2[1,3] 12
We can also use getindex() function to obtain elements from a 2-D array −
julia> getindex(arr2,1,2) 11 julia> getindex(arr2,2,3) 15
Adding Elements
We can add elements to an array in Julia at the end, at the front and at the given index using push!(), pushfirst!() and splice!() functions respectively.
At the end
We can use push!() function to add an element at the end of an array. For example,
julia> push!(arr,55) 11-element Array{Int64,1}: 5 10 15 20 25 30 35 40 45 50 55
Remember we had 10 elements in array arr. Now push!() function added the element 55 at the end of this array.
The exclamation(!) sign represents that the function is going to change the array.
At the front
We can use pushfirst!() function to add an element at the front of an array. For example,
julia> pushfirst!(arr,0) 12-element Array{Int64,1}: 0 5 10 15 20 25 30 35 40 45 50 55
At a given index
We can use splice!() function to add an element into an array at a given index. For example,
julia> splice!(arr,2:5,2:6) 4-element Array{Int64,1}: 5 10 15 20 julia> arr 13-element Array{Int64,1}: 0 2 3 4 5 6 25 30 35 40 45 50 55
Removing Elements
We can remove elements at last position, first position and at the given index, from an array in Julia, using pop!(), popfirst!() and splice!() functions respectively.
Remove the last element
We can use pop!() function to remove the last element of an array. For example,
julia> pop!(arr) 55 julia> arr 12-element Array{Int64,1}: 0 2 3 4 5 6 25 30 35 40 45 50
Removing the first element
We can use popfirst!() function to remove the first element of an array. For example,
julia> popfirst!(arr) 0 julia> arr 11-element Array{Int64,1}: 2 3 4 5 6 25 30 35 40 45 50
Removing element at given position
We can use splice!() function to remove the element from a given position of an array. For example,
julia> splice!(arr,5) 6 julia> arr 10-element Array{Int64,1}: 2 3 4 5 25 30 35 40 45 50