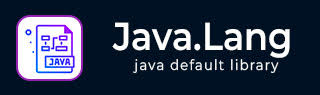
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java StackTraceElement Class
Introduction
The Java StackTraceElement class element represents a single stack frame. All stack frames except for the one at the top of the stack represent a method invocation. The frame at the top of the stack represents the execution point at which the stack trace was generated.
Class Declaration
Following is the declaration for java.lang.StackTraceElement class −
public final class StackTraceElement extends Object implements Serializable
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | StackTraceElement(String declaringClass, String methodName, String fileName, int lineNumber) This creates a stack trace element representing the specified execution point. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean equals(Object obj)
This method returns true if the specified object is another StackTraceElement instance representing the same execution point as this instance. |
2 | String getClassName()
This method returns the fully qualified name of the class containing the execution point represented by this stack trace element. |
3 | String getFileName()
This method returns the name of the source file containing the execution point represented by this stack trace element. |
4 | int getLineNumber()
This method returns the line number of the source line containing the execution point represented by this stack trace element. |
5 | String getMethodName()
This method returns the name of the method containing the execution point represented by this stack trace element. |
6 | int hashCode()
This method returns a hash code value for this stack trace element. |
7 | boolean isNativeMethod()
This method returns true if the method containing the execution point represented by this stack trace element is a native method. |
8 | String toString()
This method returns a string representation of this stack trace element. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.Object
Example: Getting Current Thread Class Name using StackTraceElement
The following example shows the usage of Java StackTraceElement getClassName() method. In this program, we've defined a function named function2() which gets the class name from StackTraceElement of current thread. Another function named function1() is used to instantiate the StackTraceElementDemo class and call the function2() method. In main method, we've called the function1() method and result is printed.
package com.tutorialspoint; public class StackTraceElementDemo { // main method public static void main(String[] args) { // call function1() to invoke function2() function1(); } // function1() is to invoke function2() // using StackTraceElementDemo object public static void function1() { new StackTraceElementDemo().function2(); } // print the class name of current thread using StackTraceElement public void function2() { System.out.print("class name : "); System.out.print(Thread.currentThread().getStackTrace()[1]. getClassName()); } }
Output
Let us compile and run the above program, this will produce the following result −
class name : com.tutorialspoint.StackTraceElementDemo