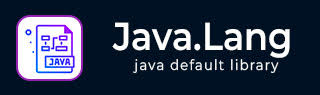
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java StringBuilder Class
StringBuilder Class Introduction
The Java StringBuilder class is mutable sequence of characters. This provides an API compatible with StringBuffer, but with no guarantee of synchronization. StringBuilder class can be used to replace StringBuffer where single threaded operations are in use. As StringBuffer has overhead of synchronization, StringBuilder acts as a faster alternative of StringBuffer. In case of multi-threaded environment, where we need to synchronized string manipulation operations, StringBuffer is recommanded, otherwise StringBuilder is to be used to improve performance.
StringBuilder Class Declaration
Following is the declaration for java.lang.StringBuilder class −
public final class StringBuilder extends Object implements Serializable, CharSequence
StringBuilder Class Constructors
Following is the list of constructors of the StringBuilder class.
Sr.No. | Constructor & Description |
---|---|
1 |
StringBuilder() This constructs a string builder with no characters in it and an initial capacity of 16 characters. |
2 |
StringBuilder(CharSequence seq) This constructs a string builder that contains the same characters as the specified CharSequence. |
3 |
StringBuilder(int capacity) This constructs a string builder with no characters in it and an initial capacity specified by the capacity argument. |
4 |
StringBuilder(String str) This constructs a string builder initialized to the contents of the specified string. |
StringBuilder Class methods
Following is the list of methods of the StringBuilder class. Each method is having multiple examples to demonstrate the functionality of the method.
Sr.No. | Method & Description |
---|---|
1 | StringBuilder append()
This method appends the given string argument to the sequence. |
2 | StringBuilder appendCodePoint(int codePoint)
This method appends the string representation of the codePoint argument to this sequence. |
3 | int capacity()
This method returns the current capacity. |
4 | char charAt(int index)
This method returns the char value in this sequence at the specified index. |
5 | int codePointAt(int index)
This method returns the character (Unicode code point) at the specified index. |
6 | int codePointBefore(int index)
This method returns the character (Unicode code point) before the specified index. |
7 | int codePointCount(int beginIndex, int endIndex)
This method returns the number of Unicode code points in the specified text range of this sequence. |
8 | StringBuilder delete(int start, int end)
This method removes the characters in a substring of this sequence. |
9 | StringBuilder deleteCharAt(int index)
This method removes the char at the specified position in this sequence. |
10 | void ensureCapacity(int minimumCapacity)
This method ensures that the capacity is at least equal to the specified minimum. |
11 | void getChars(int srcBegin, int srcEnd, char[] dst, int dstBegin)
Characters are copied from this sequence into the destination character array dst. |
12 | int indexOf(String str)
This method returns the index within this string of the first occurrence of the specified substring. |
13 | StringBuilder insert()
This method inserts the string representation of the given argument into this sequence. |
14 | int lastIndexOf(String str)
This method returns the index within this string of the rightmost occurrence of the specified substring. |
15 | int length()
This method returns the length (character count). |
16 | int offsetByCodePoints(int index, int codePointOffset)
This method returns the index within this sequence that is offset from the given index by codePointOffset code points. |
17 | StringBuilder replace(int start, int end, String str)
This method replaces the characters in a substring of this sequence with characters in the specified String. |
18 | StringBuilder reverse()
This method causes this character sequence to be replaced by the reverse of the sequence. |
19 | void setCharAt(int index, char ch)
Character at the specified index is set to ch. |
20 | void setLength(int newLength)
This method sets the length of the character sequence. |
21 | CharSequence subSequence(int start, int end)
This method returns a new character sequence that is a subsequence of this sequence. |
22 | String substring(int start)
This method returns a new String that contains a subsequence of characters currently contained in this character sequence. |
23 | String toString()
This method returns a string representing the data in this sequence. |
24 | void trimToSize()
This method attempts to reduce storage used for the character sequence. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.Object
java.lang.CharSequence
Example: Append a Boolean to the StringBuilder
The following example shows the usage of Java StringBuilder append(Boolean b) method. Here, we are instantiating a StringBuilder object "buff" with the string name "tuts". Then, we invoke the append() method using the "buff" object with a boolean argument "true". The return value will be the appended string name "tuts true". Similarly, we demonstrate another case using the string name "abcd" and a boolean argument "false".
package com.tutorialspoint; public class StringBuilderDemo { public static void main(String[] args) { StringBuilder stringBuilder = new StringBuilder("tuts "); System.out.println("builder = " + stringBuilder); // appends the boolean argument as string to the string stringBuilder stringBuilder.append(true); // print the string stringBuilder after appending System.out.println("After append = " + stringBuilder); stringBuilder = new StringBuilder("abcd "); System.out.println("stringBuilder = " + stringBuilder); // appends the boolean argument as string to the string stringBuilder stringBuilder.append(false); // print the string stringBuilder after appending System.out.println("After append = " + stringBuilder); } }
Output
Let us compile and run the above program, this will produce the following result −
stringBuilder = tuts After append = tuts true stringBuilder = abcd After append = abcd false