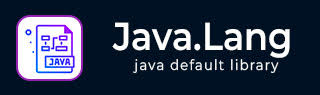
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java StackTraceElement getMethodName() Method
Description
The Java StackTraceElement getMethodName() method returns the name of the method containing the execution point represented by this stack trace element.
Declaration
Following is the declaration for java.lang.StackTraceElement.getMethodName() method
public String getMethodName()
Parameters
NA
Return Value
This method returns the name of the method containing the execution point represented by this stack trace element.
Exception
NA
Example: Getting Current Thread Method Name using StackTraceElement
The following example shows the usage of Java StackTraceElement getFileName() method. In this program, we've defined a function named function2() which gets the method name from StackTraceElement of current thread. Another function named function1() is used to instantiate the StackTraceElementDemo class and call the function2() method. In main method, we've called the function1() method and result is printed.
package com.tutorialspoint; public class StackTraceElementDemo { public static void main(String[] args) { function1(); } public static void function1() { new StackTraceElementDemo().function2(); } public void function2() { int i; System.out.println("method name : "); // print stack trace for( i = 1; i <= 3; i++ ) { System.out.println(Thread.currentThread().getStackTrace()[i]. getMethodName()); } } }
Output
Let us compile and run the above program, this will produce the following result −
method name : function2 function1 main
Example: Getting Method Name using ArithmeticException's StackTraceElement
The following example shows the usage of Java StackTraceElement getMethodName() method. In main method, we've created three int variables where one variable is carrying zero value. In try-catch block, we've created a divide by zero scenario to raise an ArithmeticException. In catch block, we've handled the ArithmeticException, retrieved an array of StackTraceElement from it using getStackTrace() method and then using getMethodName() method on first element of the array, the method name is printed.
package com.tutorialspoint; public class StackTraceElementDemo { public static void main(String[] args) { // variables to store int values int a = 0, b = 1, c; try { // being divide by zero, this line will throw an ArithmeticException c = b / a; }catch(ArithmeticException e) { // get the array of StackTraceElement StackTraceElement[] stackTraceElements = e.getStackTrace(); // print the line number System.out.println(stackTraceElements[0].getLineNumber()); } } }
Output
Let us compile and run the above program, this will produce the following result −
main
Example: Getting Line Number using Exception's StackTraceElement
The following example shows the usage of Java StackTraceElement getMethodName() method. In main method, we've created three int variables where one variable is carrying zero value. In try-catch block, we've created a divide by zero scenario to raise an ArithmeticException. In catch block, we've handled the Exception, retrieved an array of StackTraceElement from it using getStackTrace() method and then using getMethodName() method on first element of the array, the method name is printed.
package com.tutorialspoint; public class StackTraceElementDemo { public static void main(String[] args) { // variables to store int values int a = 0, b = 1, c; try { // being divide by zero, this line will throw an ArithmeticException c = b / a; }catch(Exception e) { // get the array of StackTraceElement StackTraceElement[] stackTraceElements = e.getStackTrace(); // print the method name System.out.println(stackTraceElements[0].getMethodName()); } } }
Output
Let us compile and run the above program, this will produce the following result −
main