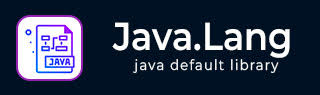
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java StackTraceElement getFileName() Method
Description
The Java StackTraceElement getFileName() method returns the name of the source file containing the execution point represented by this stack trace element.
Declaration
Following is the declaration for Java StackTraceElement getFileName() method
public String getFileName()
Parameters
NA
Return Value
This method returns the name of the file containing the execution point represented by this stack trace element, or null if this information is unavailable.
Exception
NA
Example: Getting Current Thread File Name using StackTraceElement
The following example shows the usage of Java StackTraceElement getFileName() method. In this program, we've defined a function named function2() which gets the file name from StackTraceElement of current thread. Another function named function1() is used to instantiate the StackTraceElementDemo class and call the function2() method. In main method, we've called the function1() method and result is printed.
package com.tutorialspoint; public class StackTraceElementDemo { // main method public static void main(String[] args) { // call function1() to invoke function2() function1(); } // function1() is to invoke function2() // using StackTraceElementDemo object public static void function1() { new StackTraceElementDemo().function2(); } // print the file name of current thread using StackTraceElement public void function2() { System.out.print("File name : "); System.out.print(Thread.currentThread().getStackTrace()[1]. getFileName()); } }
Output
Let us compile and run the above program, this will produce the following result −
File name : StackTraceElementDemo.java
Example: Getting File Name using ArithmeticException's StackTraceElement
The following example shows the usage of Java StackTraceElement getFileName() method. In main method, we've created three int variables where one variable is carrying zero value. In try-catch block, we've created a divide by zero scenario to raise an ArithmeticException. In catch block, we've handled the ArithmeticException, retrieved an array of StackTraceElement from it using getStackTrace() method and then using getFileName() method on first element of the array, the file name is printed.
package com.tutorialspoint; public class StackTraceElementDemo { public static void main(String[] args) { // variables to store int values int a = 0, b = 1, c; try { // being divide by zero, this line will throw an ArithmeticException c = b / a; }catch(ArithmeticException e) { // get the array of StackTraceElement StackTraceElement[] stackTraceElements = e.getStackTrace(); // print the file name System.out.println(stackTraceElements[0].getFileName()); } } }
Output
Let us compile and run the above program, this will produce the following result −
StackTraceElementDemo.java
Example: Getting File Name using Exception's StackTraceElement
The following example shows the usage of Java StackTraceElement getFileName() method. In main method, we've created three int variables where one variable is carrying zero value. In try-catch block, we've created a divide by zero scenario to raise an ArithmeticException. In catch block, we've handled the Exception, retrieved an array of StackTraceElement from it using getStackTrace() method and then using getFileName() method on first element of the array, the file name is printed.
package com.tutorialspoint; public class StackTraceElementDemo { public static void main(String[] args) { // variables to store int values int a = 0, b = 1, c; try { // being divide by zero, this line will throw an ArithmeticException c = b / a; }catch(Exception e) { // get the array of StackTraceElement StackTraceElement[] stackTraceElements = e.getStackTrace(); // print the file name System.out.println(stackTraceElements[0].getFileName()); } } }
Output
Let us compile and run the above program, this will produce the following result −
StackTraceElementDemo.java