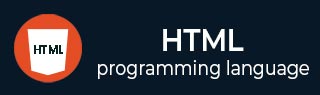
- HTML Tutorial
- HTML - Home
- HTML - History and Evolution
- HTML - Overview
- HTML - Editors
- HTML - Basic Tags
- HTML - Elements
- HTML - Attributes
- HTML - Formatting
- HTML - Headings
- HTML - Paragraphs
- HTML - Quotations
- HTML - Comments
- HTML - Phrase Tags
- HTML - Meta Tags
- HTML - Style Sheet
- HTML - CSS Classes
- HTML - CSS IDs
- HTML - Images
- HTML - Image Map
- HTML Tables
- HTML - Tables
- HTML - Headers & Caption
- HTML - Table Styling
- HTML - Table Colgroup
- HTML - Nested Tables
- HTML Lists
- HTML - Lists
- HTML - Unordered Lists
- HTML - Ordered Lists
- HTML - Definition Lists
- HTML Links
- HTML - Text Links
- HTML - Image Links
- HTML - Email Links
- HTML - Iframes
- HTML - Blocks
- HTML Backgrounds
- HTML - Backgrounds
- HTML Colors
- HTML - Colors
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML Forms
- HTML - Forms
- HTML - Form Attributes
- HTML - Form Control
- HTML - Input Attributes
- HTML Media
- HTML - Video Element
- HTML - Audio Element
- HTML - Embed Multimedia
- HTML Header
- HTML - Head Element
- HTML - Adding Favicon
- HTML - Javascript
- HTML Layouts
- HTML - Layouts
- HTML - Layout Elements
- HTML - Layout using CSS
- HTML - Responsiveness
- HTML - Symbols
- HTML - Emojis
- HTML - Style Guide
- HTML Graphics
- HTML - SVG
- HTML - Canvas
- HTML APIs
- HTML - Geolocation API
- HTML - Drag & Drop API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web Storage
- HTML - Server Sent Events
- HTML Miscellaneous
- HTML - MathML
- HTML - Microdata
- HTML - IndexedDB
- HTML - Web Messaging
- HTML - Web CORS
- HTML - Web RTC
- HTML Demo
- HTML - Audio Player
- HTML - Video Player
- HTML - Web slide Desk
- HTML Tools
- HTML - Velocity Draw
- HTML - QR Code
- HTML - Modernizer
- HTML - Validation
- HTML - Color Code Builder
- HTML References
- HTML - Tags Reference
- HTML - Attributes Reference
- HTML - Events Reference
- HTML - Fonts Reference
- HTML - ASCII Codes
- ASCII Table Lookup
- HTML - Color Names
- HTML - Entities
- MIME Media Types
- HTML - URL Encoding
- Language ISO Codes
- HTML - Character Encodings
- HTML - Deprecated Tags
- HTML Resources
- HTML - Quick Guide
- HTML - Useful Resources
- HTML - Color Code Builder
- HTML - Online Editor
HTML - CSS IDs
HTML "id" is an attribute used to uniquely identify an element within a web page. It serves as a label for that element and enables JavaScript and CSS to target it specifically. This identification helps in applying custom styles, making interactive features, and navigating within the webpage with precision. "id" values must be unique within the document.
Defining ID Attribute
In HTML and CSS, you can define an "id" attribute to uniquely identify an element and apply specific styles or JavaScript interactions. To define an "id" in HTML, simply add it to an element using the following format −
Syntax
<element id="your_id_name">Content</element>
Example
<p id="my-paragraph">This is a paragraph with an ID.</p>
In CSS, you can target this ID by using a hash symbol (#) followed by the ID name to apply styles −
Syntax
#your_id_name { property: value; }
For the previous HTML example −
#my-paragraph { color: blue; font-size: 16px; }
This allows you to uniquely style or interact with specific elements on your web page.
ID attribute in HTML
IDs are unique identifiers in HTML, used to select specific elements. They are valuable for styling and JavaScript manipulation. Below are two methods, each with an example of using IDs.
Using IDs for Styling (CSS)
In this method, we use IDs to style specific HTML elements using CSS. We define a unique ID, "special-text," and apply styles like color and font-weight to the element with that ID. It ensures that only the element with the specified ID receives these styles.
<!DOCTYPE html> <html> <head> <style> #special-text { color: blue; font-weight: bold; } </style> </head> <body> <p>This is <span id="special-text">special</span> text. </p> <p>Regular text.</p> </body> </html>
In this example, we apply CSS styles to the element with the ID "special-text."
Using IDs for JavaScript Interaction
In the below program, an ID called "special-text" is used to enable JavaScript interaction with a specific HTML element. The JavaScript function "changeText" is defined to change the content of the element when clicked.
<!DOCTYPE html> <html> <head> <script> function changeText() { var element = document.getElementById("special-text"); element.innerHTML = "new text"; } </script> </head> <body> <p>This is <span id="special-text" onclick="changeText()">special</span> text. </p> <p>Regular text.</p> </body> </html>
ID Attribute Rules in HTML
Make sure that the ID attribute follows the below rules or not −
In the ID attribute at least one character should be there, the starting letter should be a character (a-z) or (A-Z), and the rest of the letters of any type can written even special characters.
The ID attribute does not contain any spaces.
Within the document every ID must be unique.
Example
The following example shows the values of the ID attribute −
<div id = "#123"> .... </div> <tag id = "#%ADF@"> .... </tag> <h1 id = " _H"> .... </h1> <div id = "@"> .... </tag> <tag id = " {}"> .... </tag>
Before understanding whether these values for the ID attribute are valid or not, try to understand the class attribute which is explained in the class chapter, and the difference between class and ID attribute, it helps in understanding about ID attribute in detail.
Valid and Invalid ID attributes
Certain ID Attributes are valid in HTML 5, but not in Cascading Style Sheets. In such cases, it is recommended to go with simple output rather than styled output because certain values that we use for ID may be invalid in CSS.
The following example demonstrates the use of simple ID attributes using CSS −
Example
<!DOCTYPE html> <html> <head> <title>Simple Id Attributes</title> <style> #@TP { color: #070770; text-align: center; font-size: 30px; font-weight: bold; } #@TP1 { text-align: center; font-size: 25px; } </style> </head> <body> <div id="@TP">TutorialsPoint Website</div> <div id="@TP1"> Html5 Tutorials, CSS Tutorials, JavaScript Tutorials </div> </body> </html>
When we execute the above code, two div elements will be displayed, one with ID attribute (TutorialsPoint Website), and the other one with other ID attribute (Html5 Tutorials, CSS Tutorials, JavaScript Tutorials).
Now we will change the value IDs in the above example. ID’s values are valid in HTML 5 and in CSS. Therefore, we can get a styled output because of valid ID’s. Following is the example which demonstrate the functioning of valid ID’s −
<!DOCTYPE html> <html> <head> <title>Simple Id Attributes</title> <style> #TP1 { color: #070770; text-align: center; font-size: 30px; font-weight: bold; } #TP2 { text-align: center; font-size: 25px; } </style> </head> <body> <div id="TP1">TutorialsPoint Website</div> <div id="TP2">Html5 Tutorials, CSS Tutorials, JavaScript Tutorials</div> </body> </html>
When we execute the above program, two div elements with ID attributes are displayed.
Consider another example which demonstrates "Invalid ID’s not supported in JavaScript" notion.
<!DOCTYPE html> <html> <body> <h1 id="1TP">TutorialsPoint WEBSITE Content</h1> <button onclick="Value()"> Display </button> <style> #.1TP { color: blue; } </style> <script> function Value() { document.getElementById("1TP").innerHTML = "TutorialsPoint"; } </script> </body> </html>
Consider another example which demonstrates Valid ID’s that are supported by JavaScript and HTML.
<!DOCTYPE html> <html> <body> <h1 id="TP">TutorialsPoint WEBSITE Content</h1> <button onclick="displayResult()"> Display </button> <style> #TP { color: blue; } </style> <script> function displayResult() { document.getElementById("TP").innerHTML = "TutorialsPoint"; } </script> </body> </html>
When we run the above program, an ID attribute will be displayed along with the button labeled as display.
When ID value is taken only in characters, then JavaScript and HTML supports the ID and When Clicked on the display button the below page will open.
To Continue Learning Please Login