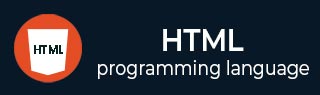
- HTML Tutorial
- HTML - Home
- HTML - Introduction
- HTML - Editors
- HTML - Basic Tags
- HTML - Elements
- HTML - Attributes
- HTML - Headings
- HTML - Paragraphs
- HTML - Fonts
- HTML - Blocks
- HTML - Style Sheet
- HTML - Formatting
- HTML - Quotations
- HTML - Comments
- HTML - Colors
- HTML - Images
- HTML - Image Map
- HTML - Iframes
- HTML - Phrase Elements
- HTML - Meta Tags
- HTML - Classes
- HTML - IDs
- HTML - Backgrounds
- HTML Tables
- HTML - Tables
- HTML - Headers & Caption
- HTML - Table Styling
- HTML - Table Colgroup
- HTML - Nested Tables
- HTML Lists
- HTML - Lists
- HTML - Unordered Lists
- HTML - Ordered Lists
- HTML - Definition Lists
- HTML Links
- HTML - Text Links
- HTML - Image Links
- HTML - Email Links
- HTML Color Names & Values
- HTML - Color Names
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML Forms
- HTML - Forms
- HTML - Form Attributes
- HTML - Form Control
- HTML - Input Attributes
- HTML Media
- HTML - Video Element
- HTML - Audio Element
- HTML - Embed Multimedia
- HTML Header
- HTML - Head Element
- HTML - Adding Favicon
- HTML - Javascript
- HTML Layouts
- HTML - Layouts
- HTML - Layout Elements
- HTML - Layout using CSS
- HTML - Responsiveness
- HTML - Symbols
- HTML - Emojis
- HTML - Style Guide
- HTML Graphics
- HTML - SVG
- HTML - Canvas
- HTML APIs
- HTML - Geolocation API
- HTML - Drag & Drop API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web Storage
- HTML - Server Sent Events
- HTML Miscellaneous
- HTML - MathML
- HTML - Microdata
- HTML - IndexedDB
- HTML - Web Messaging
- HTML - Web CORS
- HTML - Web RTC
- HTML Demo
- HTML - Audio Player
- HTML - Video Player
- HTML - Web slide Desk
- HTML Tools
- HTML - Velocity Draw
- HTML - QR Code
- HTML - Modernizer
- HTML - Validation
- HTML - Color Picker
- HTML References
- HTML - Cheat Sheet
- HTML - Tags Reference
- HTML - Attributes Reference
- HTML - Events Reference
- HTML - Fonts Reference
- HTML - ASCII Codes
- ASCII Table Lookup
- HTML - Color Names
- HTML - Entities
- MIME Media Types
- HTML - URL Encoding
- Language ISO Codes
- HTML - Character Encodings
- HTML - Deprecated Tags
- HTML Resources
- HTML - Quick Guide
- HTML - Useful Resources
- HTML - Color Code Builder
- HTML - Online Editor
HTML - Classes
The class is an important keyword in HTML. It is an attribute that can be applied to one or more elements and is used to style and categorize elements based on common characteristics or purpose. Classes allows multiple elements to share the same styling rules. By assigning the same class to multiple elements, you can apply CSS styles or JavaScript functionality to all of them simultaneously. This promotes consistency in design and layout, making it easier to manage and update a website.
HTML class attribute is defined in the HTML code using the "class" keyword, and the styling is determined in CSS. This separation of content and style is a key principle in web design, facilitating the creation of visually appealing and organized web pages.
Syntax for Class
To create a CSS rule for HTML elements using class attribute in CSS write a (.) followed by the class name mentioned in HTML element, the we can define the CSS prpeties with curly braces in key: value;
format like color: yellow;
.
In this code, we've selected a class named "highlight" that changes the background color, text color, and font weight of the elements it's applied to.
- In HTML:
<element class="highlight">...</element>
- In CSS:
/* CSS using class Attribute Selector */ .highlight { background-color: yellow; color: black; font-weight: bold; }
- In JavaScript:
document.getElementsByClassName('highlight')
Using HTML Class Attribute
HTML classes are essential for styling and formatting web page elements consistently. They allow you to apply the same styles to multiple elements without repeating code, promoting maintainability and a cohesive design. The class attribute can be used on any HTML Elements(Except elements placed in head element). Here's how to use classes effectively with a practical example.
Define a Class for Styling
In the following example, we have create two element one is h1 and other is p, and we set class on them as well "header" & "heightlight" but using the "heightlight" class in internal CSS to style our p element. You can use the "header" class in the similar way to style the h1 element.
<!DOCTYPE html> <html> <head> <style> <!-- CSS class attribute Selector Used --> .highlight { background-color: yellow; color: black; padding: 5px; } </style> </head> <body> <!-- Using class attribute in both Element--> <h1 class="header">Tutorialspoint</h1> <p class="highlight">Simply Easy Learning</p> </body> </html>
Multiple classes
We can apply multiple classes to a single element by separating class names with a space.
In the following example, the <h1> element has two classes applied "heading" and "content." This is achieved using a space to separate the class names within the class attribute.
Multiple classes can be applied to the same element to inherit styling from both classes. In this case, "heading" class provides a large font size and center alignment, while the "content" class provides a specific text color and line-height.
<!DOCTYPE html> <html> <head> <style> .heading { font-size: 24px; color: #333; text-align: center; } .content { font-size: 16px; color: #666; line-height: 1.5; } .button { background-color: #007bff; color: #fff; padding: 10px 20px; border: none; cursor: pointer; } </style> </head> <body> <!-- Defined two Classes in h1 Element --> <h1 class="heading content"> Welcome to Tutorialspoint </h1> <p class="content"> We make Tutorials - Simply Easy Learning </p> <button class="button">Click Me</button> </body> </html>
Same class on Multiple Elements
The most important feature of classes is their reusability. You can apply the same class to multiple elements to maintain a consistent look throughout your website. Here in the following example we create 2 p elements(paragraphs). Both of these paragraphs will have the same highlighting because they share the "highlight" class.
<!DOCTYPE html> <html> <head> <style> .highlight { background-color: yellow; color: black; font-weight: bold; } </style> </head> <body> <p class="highlight"> To create a class, you need to define it within your HTML document or link to an external CSS file that contains class definitions. Classes are defined using the "class" attribute. </p> <p class="highlight"> HTML classes are essential for styling and formatting web page elements consistently. They allow you to apply the same styles to multiple elements without repeating code, promoting maintainability and a cohesive design. </p> </body> </html>
Using class Attribute through JavaScript
HTML classes are versatile and serve various purposes beyond styling.
The classes are frequently used to identify elements for JavaScript functions. For example, you can use a class to target specific elements, like buttons, and make them interactive through JavaScript. In the following code we have create a button which will trigger a function that will change the display property none to block of a p element. You will see a paragraph.
<!DOCTYPE html> <html> <head> <script> function showContent() { var element = document.getElementsByClassName('content')[0]; if (element.style.display === 'none') { element.style.display = 'block'; } else { element.style.display = 'none'; } } </script> <style> .interactive-button { background-color: #007bff; color: #fff; padding: 10px 20px; border: none; cursor: pointer; } </style> </head> <body> <button class="interactive-button" onclick="showContent()">Click Me</button> <p class="content" style="display: none;"> This content can be toggled by clicking the button. </p> </body> </html>
Things to Remember about Class
- More than 1 class can be define on any HTML element.
- Class are used by CSS and JavaScript both to select the element.
- The class is case sensitive so be careful when you are using to select the element.
- Multiple elements can have the same class as well.
- In CSS we use
.className
and in JavaScriptgetElementsByClassName()
method to select the class assigned HTML element.