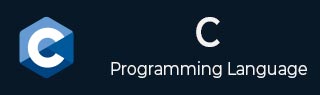
- C Programming Tutorial
- C - Home
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- Loops in C
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Error Handling in C
As such, C programming does not provide direct support for error handling, as there are no keywords in C that can prevent errors or exceptions from abruptly terminating the program. However, a programmer can use other functions for error handling.
You can use errno for efficient error handling in C. Additionally other functions that can be used for error handling include perror, strerror, ferror, and clearererr.
The errno Variable
C is a system programming language. It provides you access at a lower level in the form of return values. Most of the C or even Unix function calls return -1 or NULL in case of any error and set an error code errno. It is set as a global variable and indicates an error occurred during any function call. You can find various error codes defined in <error.h> header file.
So, a C programmer can check the returned values and can take appropriate action depending on the return value. It is a good practice, to set errno to 0 at the time of initializing a program. A value of 0 indicates that there is no error in the program.
The following table shows the errno values and error messages associated with them −
errno value | Error |
---|---|
1 | Operation not permitted |
2 | No such file or directory |
3 | No such process |
4 | Interrupted system call |
5 | I/O error |
6 | No such device or address |
7 | The argument list is too long |
8 | Exec format error |
9 | Bad file number |
10 | No child processes |
11 | Try again |
12 | Out of memory |
13 | Permission denied |
Example
Take a look at the following example −
#include <stdio.h> #include <errno.h> int main() { FILE* fp; // opening a file which does not exist fp = fopen("nosuchfile.txt", "r"); printf("Value of errno: %d\n", errno); return 0; }
Output
It will produce the following output −
Value of errno: 2
The C programming language provides perror() and strerror() functions which can be used to display the text message associated with errno.
The perror() Function
displays the string you pass to it, followed by a colon, a space, and then the textual representation of the current errno value.
void perror(const char *str);
Example
In the above example, the "errno = 2" is associated with the message No such file or directory, which can be printed with perror() function
#include <stdio.h> #include <errno.h> int main(){ FILE* fp; // opening a file which does not exist fp = fopen("nosuchfile.txt", "r"); printf("Value of errno: %d\n", errno); perror("Error message:"); return 0; }
Output
When you run this code, it will produce the following output −
Value of errno: 2 Error message: No such file or directory
The strerror() Function
This returns a pointer to the textual representation of the current errno value.
char *strerror(int errnum);
Let us use this function to display the textual representation of errno=2
Example
Take a look at the following example −
#include <stdio.h> #include <errno.h> int main() { FILE* fp; // opening a file which does not exist fp = fopen("nosuchfile.txt", "r"); printf("Value of errno: %d\n", errno); printf("The error message is : %s\n", strerror(errno)); return 0; }
Output
Value of errno: 2 he error message is : No such file or directory
The ferror() Function
This function is used to check whether an error occurred during a file operation.
int ferror(FILE *stream);
Example
Here, we try to read from a file opened in ‘w’ mode. The ferror() function is used to print the error message
#include <stdio.h> int main(){ FILE *fp; fp = fopen("test.txt","w"); char ch = fgetc(fp); // Trying to read data, from writable file if(ferror(fp)){ printf("File is opened in writing mode! You cannot read data from it!"); } fclose(fp); return(0); }
Output
Run the code and check its output −
File is opened in writing mode! You cannot read data from it!
The clearerr() Function
The clearerr() function is used to clear both end-of-file and error indicators for a file stream.
void clearerr(FILE *stream);
Example
Take a look at the following example −
#include <stdio.h> int main(){ FILE *fp; fp = fopen("test.txt","w"); char ch = fgetc(fp); // Trying to read data, from writable file if(ferror(fp)){ printf("File is opened in writing mode! You cannot read data from it!\n"); } // Clears error-indicators from the file stream // Subsequent ferror() doesn't show error clearerr(fp); if(ferror(fp)){ printf("Error again in reading from file!"); } fclose(fp); return(0); }
Divide by Zero Errors
It is a common problem that at the time of dividing any number, programmers do not check if a divisor is zero and finally it creates a runtime error.
Example 1
The following code fixes this error by checking if the divisor is zero before dividing −
#include <stdio.h> #include <stdlib.h> int main() { int dividend = 20; int divisor = 0; int quotient; if( divisor == 0){ fprintf(stderr, "Division by zero! Exiting...\n"); exit(-1); } quotient = dividend / divisor; fprintf(stderr, "Value of quotient : %d\n", quotient ); exit(0); }
Output
When the above code is compiled and executed, it produces the following result −
Division by zero! Exiting... Program Exit Status
It is a common practice to exit with a value of EXIT_SUCCESS in case of program coming out after a successful operation. Here, EXIT_SUCCESS is a macro and it is defined as 0.
Example 2
If you have an error condition in your program and you are coming out then you should exit with a status EXIT_FAILURE which is defined as "-1". So let's write the above program as follows −
#include <stdio.h> #include <stdlib.h> int main() { int dividend = 20; int divisor = 5; int quotient; if(divisor == 0) { fprintf(stderr, "Division by zero! Exiting...\n"); exit(EXIT_FAILURE); } quotient = dividend / divisor; fprintf(stderr, "Value of quotient: %d\n", quotient ); exit(EXIT_SUCCESS); }
Output
When the above code is compiled and executed, it produces the following result −
Value of quotient: 4