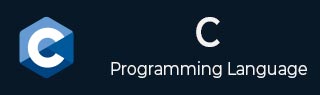
- C Programming Tutorial
- C - Home
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- Loops in C
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Math Functions in C
The standard library of C includes a number of mathematical functions that perform often required computations such as finding trigonometric ratios, calculating log and exponentials, rounding the numbers, etc.
Most of the mathematical functions are defined in the "math.h" header file. In this chapter, let us focus on the library functions in C that are related to mathematical calculations.
Trigonometric Functions
The math.h library defines the function sin(), cos() and tan() – that return the respective trigonometric ratios, sine, cosine and tangent of an angle.
These functions return the respective ratio for a given double type representing the angle expressed in radians. All the functions return a double value.
double sin(double x) double cos(double x) double tan(double x)
For all the above functions, the argument "x" is the angle in radians.
Example
The following example shows how you can use trigonometric functions in C −
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main() { double x, sn, cs, tn, val; x = 45.0; val = PI / 180; sn = sin(x*val); cs = cos(x*val); tn = tan(x*val); printf("sin(%f) : %f\n", x, sn); printf("cos(%f) : %f\n", x, cs); printf("tan(%f) : %f\n", x, tn); return(0); }
Output
When you run this code, it will produce the following output −
sin(45.000000) : 0.707107 cos(45.000000) : 0.707107 tan(45.000000) : 1.000000
Inverse Trigonometric Functions
The math.h library also includes inverse trigonometric functions, also known as arcus functions or anti-trigonometric functions. They are the inverse functions of basic trigonometric functions. For example, asin(x) is equivalent to $\mathrm{sin^{-1}(x)}$. The other inverse functions are acos(), atan() and atan2().
The following asin() function returns the arc sine of "x" in the interval [-pi/2, +pi/2] radians −
double asin(double x)
The following acos() function returns principal arc cosine of "x" in the interval [0, pi] radians −
double acos(double x)
The following atan() function returns the principal arc tangent of "x" in the interval [-pi/2, +pi/2] radians.
double atan(double x)
Example 1
The following example demonstrates how you can use inverse trigonometric functions in a C program −
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main() { double x, asn, acs, atn, val; x = 0.9; val = 180/PI; asn = asin(x); acs = acos(x); atn = atan(x); printf("asin(%f) : %f in radians\n", x, asn); printf("acos(%f) : %f in radians\n", x, acs); printf("atan(%f) : %f in radians\n", x, atn); asn = (asn * 180) / PI; acs = (acs * 180) / PI; atn = (atn * 180) / PI; printf("asin(%f) : %f in degrees\n", x, asn); printf("acos(%f) : %f in degrees\n", x, acs); printf("atan(%f) : %f in degrees\n", x, atn); return(0); }
Output
When you run this code, it will produce the following output −
asin(0.900000) : 1.119770 in radians acos(0.900000) : 0.451027 in radians atan(0.900000) : 0.732815 in radians asin(0.900000) : 64.158067 in degrees acos(0.900000) : 25.841933 in degrees atan(0.900000) : 41.987213 in degrees
The atan2() function returns the arc tangent in radians of "y/x" based on the signs of both values to determine the correct quadrant.
double atan2(double y, double x)
This function returns the principal arc tangent of "y / x" in the interval [-pi, +pi] radians.
Example 2
Take a look at the following example −
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main() { double x, y, ret, val; x = -7.0; y = 7.0; val = 180.0 / PI; ret = atan2 (y,x) * val; printf("The arc tangent of x = %lf, y = %lf ", x, y); printf("is %lf degrees\n", ret); return(0); }
Output
Run the code and check its output −
The arc tangent of x = -7.000000, y = 7.000000 is 135.000000 degrees
Hyperbolic Functions
In Mathematics, hyperbolic functions are similar to trigonometric functions but are defined using the hyperbola rather than the circle. The math.h header file provides sinh(), cosh() and tanh() functions.
double sinh(double x)
This function returns hyperbolic sine of x.
double cosh(double x)
This function returns hyperbolic cosine of x.
double tanh(double x)
This function returns hyperbolic tangent of x.
Example
The following example shows how you can use hyperbolic functions in a C program −
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main() { double x,val, sh, ch, th; x = 45; val = PI/180.0 ; sh = sinh(x*val); ch = cosh(x*val); th = tanh(x*val); printf("The sinh(%f) = %lf\n", x, sh); printf("The cosh(%f) = %lf\n", x, ch); printf("The tanh(%f) = %lf\n", x, th); return(0); }
Output
Run the code and check its output −
The sinh(45.000000) = 0.868671 The cosh(45.000000) = 1.324609 The tanh(45.000000) = 0.655794
Exponentiation and Logarithmic Functions
The "math.h" library includes the following functions related to exponentiation and logarithms −
The exp() Function: It returns the value of e raised to the xth power. (Value of e - Euler’s number – is 2.718 approx)
double exp(double x)
The log() Function: It returns the natural logarithm (base-e logarithm) of "x".
double log(double x)
Note that Logarithmic functions are equivalent to the exponential function’s inverse.
The log10() Function: It returns the common logarithm (base-10 logarithm) of "x".
double log10(double x)
Example
The following example shows how you can use exponentiation and logarithmic functions in a C program −
#include <stdio.h> #include <math.h> #define PI 3.14159265 int main () { double x = 2; double e, ln, ls; e = exp(2); ln = log(e); printf("exp(%f): %f log(%f): %f\n",x, e, e, ln); ln = log(x); printf("log(%f): %f\n",x,ln); ls = log10(x); printf("log10(%f): %f\n",x,ls); return(0); }
Output
When you run this code, it will produce the following output −
exp(2.000000): 7.389056 log(7.389056): 2.000000 log(2.000000): 0.693147 log10(2.000000): 0.301030
The frexp() Function
The "math.h" header file also includes the frexp() function. It breaks a floating-point number into its significand and exponent.
double frexp(double x, int *exponent)
Here, "x" is the floating point value to be computed and "exponent" is the pointer to an int object where the value of the exponent is to be stored.
This function returns the normalized fraction.
Example
Take a look at the following example −
#include <stdio.h> #include <math.h> int main () { double x = 1024, fraction; int e; fraction = frexp(x, &e); printf("x = %.2lf = %.2lf * 2^%d\n", x, fraction, e); return(0); }
Output
Run the code and check its output −
x = 1024.00 = 0.50 * 2^11
The ldexp() Function
The ldexp() function combines a significand and an exponent to form a floating-point number. Its syntax is as follows −
double ldexp(double x, int exponent)
Here, "x" is the floating point value representing the significand and "exponent" is the value of the exponent. This function returns (x * 2 exp)
Example
The following example shows how you can use this ldexp() function in a C program −
#include <stdio.h> #include <math.h> int main () { double x, ret; int n; x = 0.65; n = 3; ret = ldexp(x ,n); printf("%f * 2 %d = %f\n", x, n, ret); return(0); }
Output
Run the code and check its output −
0.650000 * 2^3 = 5.200000
The pow() Function
This function returns x raised to the power of y i.e. xy.
double pow(double x, double y)
The sqrt() Function
returns the square root of x.
double sqrt(double x)
The sqrt(x) function returns a value which is same as pow(x, 0.5)
Example
The following example shows how you can use the pow() and sqrt() functions in a C program −
#include <stdio.h> #include <math.h> int main() { double x = 9, y=2; printf("Square root of %lf is %lf\n", x, sqrt(x)); printf("Square root of %lf is %lf\n", x, pow(x, 0.5) ); printf("%lf raised to power %lf\n", x, pow(x, y)); return(0); }
Output
When you run this code, it will produce the following output −
Square root of 9.000000 is 3.000000 Square root of 9.000000 is 3.000000 9.000000 raised to power 81.000000
Rounding Functions
The math.h library includes ceil(), floor() and round() functions that round off the given floating point number.
The ceil() Function
This returns the smallest integer value greater than or equal to x.
double ceil(double x)
This function returns the smallest integral value not less than x.
The floor() Function
This function returns the largest integer value less than or equal to x.
double floor(double x)
Parameter x : This is the floating point value. This function returns the largest integral value not greater than x.
The round() Function
This function is used to round off the double, float or long double value passed to it as a parameter to the nearest integral value.
double round( double x )
The value returned is the nearest integer represented as floating point
Example
The following example demonstrates how you can use the rounding functions in a C program −
#include <stdio.h> #include <math.h> int main() { float val1, val2, val3, val4; val1 = 1.2; val2 = 1.6; val3 = 2.8; val4 = -2.3; printf ("ceil(%lf) = %.1lf\n", val1, ceil(val1)); printf ("floor(%lf) = %.1lf\n", val2, floor(val2)); printf ("ceil(%lf) = %.1lf\n", val3, ceil(val3)); printf ("floor(%lf) = %.1lf\n", val4, floor(val4)); printf("round(%lf) = %.1lf\n", val1, round(val1)); printf("round(%lf) = %.1lf", val4, round(val4)); return(0); }
Output
When you run this code, it will produce the following output −
ceil(1.200000) = 2.0 floor(1.600000) = 1.0 ceil(2.800000) = 3.0 floor(-2.300000) = -3.0 round(1.200000) = 1.0 round(-2.300000) = -2.0
Modulus Functions
The "math.h" library includes the following functions in this category:
The modf() Function
This function returns the fraction component (part after the decimal), and sets integer to the integer component.
double modf(double x, double *integer)
Here, "x" is the floating point value and "integer" is the pointer to an object where the integral part is to be stored.
This function returns the fractional part of "x" with the same sign.
Example
Take a look at the following example −
#include <stdio.h> #include <math.h> int main () { double x, fractpart, intpart; x = 8.123456; fractpart = modf(x, &intpart); printf("Integral part = %lf\n", intpart); printf("Fraction Part = %lf \n", fractpart); return(0); }
Output
When you run this code, it will produce the following output −
Integral part = 8.000000 Fraction Part = 0.123456
The fmod() Function
This function returns the remainder of x divided by y.
double fmod(double x, double y)
Here, "x" is the numerator and "y" is the denominator. The function returns remainder of "x / y".
Example
Take a look at the following example −
#include <stdio.h> #include <math.h> int main () { float a, b; int c; a = 9.2; b = 3.7; c = 2; printf("Remainder of %f / %d is %lf\n", a, c, fmod(a,c)); printf("Remainder of %f / %f is %lf\n", a, b, fmod(a,b)); return(0); }
Output
When you run this code, it will produce the following output −
Remainder of 9.200000 / 2 is 1.200000 Remainder of 9.200000 / 3.700000 is 1.800000
Note that the modulus operator (%) works only with integer operands.