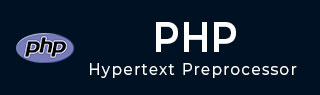
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Passing Functions
To a function in PHP, in addition to scalar types, arrays, and objects, you can also pass a function as one of its arguments. If a function is defined to accept another function as an argument, the passed function will be invoked inside it. PHP’s standard library has certain built-in functions of this type, where one of the arguments to be passed is a function, which may be another built-in function or even a user defined function.
array_map
The array_map() is one of the built-in functions. The first argument to this function is a callback function. There may be one or more arrays as the other arguments. The callback function is applied to all the elements of arrays.
array_map(?callable $callback, array $array, array ...$arrays): array
The array_map() function returns an array. It contains the result of applying the callback function to the corresponding elements of arrays passed as other arguments.
Example
In the following example, we have a square() function that computes the square of a number passed to it. This function in turn is used as an argument for array_map() function, along with another array of numbers. Each number is successively passed to the squares() function. The resultant array is a list of squares.
<?php function square($number) { return $number * $number; } $arr = [1, 2, 3, 4, 5]; $squares = array_map('square', $arr); var_dump($squares); ?>
It will produce the following output −
array(5) { [0]=> int(1) [1]=> int(4) [2]=> int(9) [3]=> int(16) [4]=> int(25) }
call_user_func
Another example of passing a function to another function is call_user_func(). As the name suggests, it calls another user defined callback function, and the other arguments are passed to the callback.
call_user_func(callable $callback, mixed ...$args): mixed
Example
In the example below, the square() function is invoked repeatedly, passing each number in an array.
<?php function square($number) { return $number * $number; } $arr = [1, 2, 3, 4, 5]; foreach($arr as $a) { echo "square of $a:" . call_user_func("square", $a). PHP_EOL; } ?>
It will produce the following output −
square of 1:1 square of 2:4 square of 3:9 square of 4:16 square of 5:25
usort
As another example of passing function, we take a look a usort() function.
usort(array &$array, callable $callback): true
The first parameter is an array. The array is sorted as per the callback function, which is the second parameter.
The callback parameter is a comparison function must return an integer less than, equal to, or greater than zero if the first argument is considered to be respectively less than, equal to, or greater than the second.
Example
Here is an example. First we have a mysort() function. It compares two numbers and returns "-1", "0" or "1" if the first number is less than, equal to or greater than second number.
The first argument to usort() is the mysort() function, and the second one is an array. To begin with, the first two numbers are passed to mysort(). If it returns 1, they are swapped. Next, the second and third numbers are passed and swapped if the comparison returns 1. The same process repeats so that the array elements are arranged in ascending order.
<?php function mysort($a, $b) { if ($a == $b) { return 0; } return ($a < $b) ? -1 : 1; } $a = array(3, 2, 5, 6, 1); usort($a, "mysort"); foreach ($a as $key => $value) { echo "$key: $value\n"; } ?>
It will produce the following output −
0: 1 1: 2 2: 3 3: 5 4: 6
Pass Callback to User-defined Function
Apart from the above built-in functions, you can define your own function that accepts one of the arguments as another function.
In the example below, we have two functions, square() and cube(), that return the square and cube of a given number.
Next, there is myfunction(), whose first argument is used as a variable function and the second argument to myfunction() is passed to it.
Thus, myfunction() internally calls square() or cube() to return either square or cube of a given number.
Example
<?php function myfunction($function, $number) { $result = $function($number); return $result; } function cube($number) { return $number ** 2; } function square($number) { return $number ** 3; } $x = 5; $cube = myfunction('cube', $x); $square = myfunction('square', $x); echo "Square of $x = $square" . PHP_EOL; echo "Cube of $x = $cube" . PHP_EOL; ?>
It will produce the following output −
Square of 5 = 125 Cube of 5 = 25