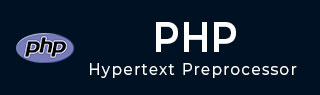
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - File Handling
In PHP, a file is a resource object, from which data can be read or written to in a linear fashion. The term "file handling" refers to a set of functions in PHP that enable read/write operations on disk files with PHP code.
A file object is classified as a stream. Any resource on which linear read/write operations are done is a stream. Other stream-like objects are the TCP sockets, standard input stream, i.e., a system keyboard represented by "php://stdin", the standard output stream represented by "php://stdout", and the error stream "php://stderr".
Note − Tthe constants STDIN, STDOUT, and STDERR stand for the respective standard streams.
Although PHP is regarded as a server-side scripting language for developing web applications, PHP also has a command-line interface to perform console IO operations.
Example
The readline() function in PHP accepts the user input from a standard keyboard, and echo/print statements render the output on the console.
<?php $str = readline("Type something:"); echo $str; ?>
It will produce the following output −
C:\xampp\php>php hello.php Type something: Are you enjoying this PHP tutorial? Are you enjoying this PHP tutorial?
Example
We can obtain the same effect by reading the input from "php://stdin" and outputting it to "php://stdout".
<?php $f = fopen("php://stdin", "r"); echo "Type something: "; $str = fgets($f); $f1 = fopen("php://stdout", "w"); fputs($f1, $str); ?>
Here, the fopen() function is used to open the stdin stream for reading and the stdout stream for writing.
Example
PHP supports a variety of stream protocols for stream related functions such as fopen(), file_exists(), etc. Use php_get_wrappers() function to get a list of all the registered wrappers.
<?php print_r(stream_get_wrappers()); ?>
It will produce the following output −
Array ( [0] => php [1] => file [2] => glob [3] => data [4] => http [5] => ftp [6] => zip [7] => compress.zlib [8] => compress.bzip2 [9] => https [10] => ftps [11] => phar )
The streams are referenced as "scheme://target". For instance, the file stream is "file://xyz.txt".
The input data from the console is stored in the computer's main memory (RAM) until the application is running. Thereafter, the memory contents from RAM are erased.
We would like to store it in such a way that it can be retrieved whenever required in a persistent medium such as a disk file. Hence, instead of the standard streams (keyboard for input and the display device for output), we will use the disk files for reading the data, and destination for storing the data.
In addition to the read and write modes as used in the above example (IO operations with standard streams), the file stream can be opened in various other modes like "r+" and "w+" for simultaneous read/write, "b" for binary mode, etc.
To open a disk file for reading and obtain its reference pointer, use the fopen() function.
$handle = fopen('file://' . __DIR__ . '/data.txt', 'r');
The "file://" scheme is the default. Hence, it can be easily dropped, especially when dealing with local files.
Note − It is always recommended to close the stream that was opened. Use the fclose() function for this purpose.
fclose($handle);
PHP has several built-in functions for performing read/write operations on the file stream. In the subsequent chapters, we shall explore the filesystem functions.