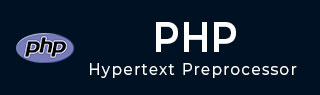
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Encapsulation
PHP implements encapsulation, one of the important principles of OOP with access control keywords: public, private and protected.
Encapsulation refers to the mechanism of keeping the data members or properties of an object away from the reach of the environment outside the class, allowing controlled access only through the methods or functions available in the class.
The following diagram illustrates the principle of encapsulation in object-oriented programming methodology.
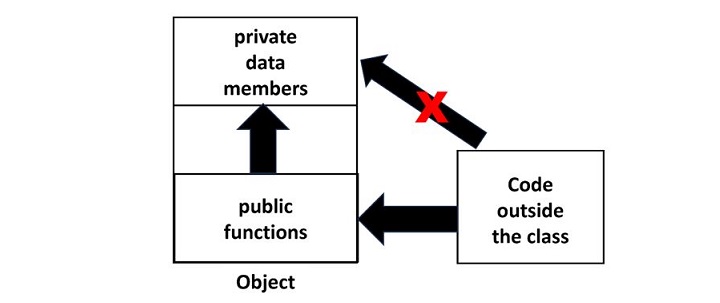
PHP’s keywords list contains the following keywords that determine the accessibility of properties and methods of an object, which is an instance of a class in PHP −
Public − Class members are accessible from anywhere, even from outside the scope of the class, but only with the object reference.
Private − Class members can be accessed within the class itself. It prevents members from outside class access even with the reference of the class instance.
Protected − Members can be accessed within the class and its child class only, nowhere else.
These three keywords "public, private and protected" are often called access modifiers. They are also referred as visibility modes, as they decide upto what extent a certain class member is available.
Public Members
In PHP, the class members (both member variables as well as member functions) are public by default.
Example
In the following program, the member variables title and price of the object are freely accessible outside the class because they are public by default, if not otherwise specified.
<?php class Person { /* Member variables */ var $name; var $age; /*Constructor*/ function __construct(string $param1="Ravi", int $param2=28) { $this->name = $param1; $this->age = $param2; } function getName() { echo "Name: $this->name" . PHP_EOL;; } function getAge() { echo "Age: $this->age" . PHP_EOL;; } } $b1 = new Person(); $b1->getName(); $b1->getAge(); echo "Name : $b1->name Age: $b1->age" . PHP_EOL; ?>
It will produce the following output −
Name: Ravi Age: 28 Name : Ravi Age: 28
Note that the properties all the class members are public by default, you can explicitly declare them as public if desired. As a result, the instance methods getName() and getAge() can be called from outside the class.
Since properties name and age are also public, hence they can also be accessed outside the class, something which is not desired as per the principle of encapsulation.
Private Members
As mentioned above, the principle of encapsulation requires that the member variables should not be accessible directly. Only the methods should have the access to the data members. Hence, we need to make the member variables private and methods public.
Example
Let us change the declaration of name and age properties to private and run the following PHP script −
<?php class Person { /* Member variables */ private $name; private $age; /*Constructor*/ function __construct(string $param1="Ravi", int $param2=28) { $this->name = $param1; $this->age = $param2; } public function getName() { echo "Name: $this->name" . PHP_EOL;; } public function getAge(){ echo "Age: $this->age" . PHP_EOL;; } } $b1 = new Person(); $b1->getName(); $b1->getAge(); echo "Name : $b1->name Age: $b1->age" . PHP_EOL; ?>
It will produce the following output −
Name: Ravi Age: 28 PHP Fatal error: Uncaught Error: Cannot access private property Person::$name in person.php:27
The error message tells the reason that a private property cannot be accessed from a public scope.
Protected Members
The effect of specifying protected access to a class member is effective in case of class inheritance. We know that public members are accessible from anywhere outside the class, and private members are denied access from anywhere outside the class.
The protected keyword grants access to an object of the same class and an object of its inherited class, denying it to any other environment.
Example
Let us inherit the person class and define a student class. We shall change the name property from private to protected. The student class has a new public method getDetails() that prints the values of name and age properties.
Person class
<?php class Person { /* Member variables */ protected $name; private $age; /*Constructor*/ function __construct(string $param1="Ravi", int $param2=28) { $this->name = $param1; $this->age = $param2; } public function getName(){ echo "Name: $this->name" . PHP_EOL;; } public function getAge() { echo "Age: $this->age" . PHP_EOL;; } }
Student class
class student extends Person { public function getDetails() { echo "My Name: $this->name" . PHP_EOL; echo "My age: $this->age" . PHP_EOL; } } $s1 = new student(); $s1->getDetails(); ?>
It will produce the following output −
My Name: Ravi PHP Warning: Undefined property: student::$age in person.php on line 28 My age:
The following table illustrates the rules of accessibility of class members in PHP −
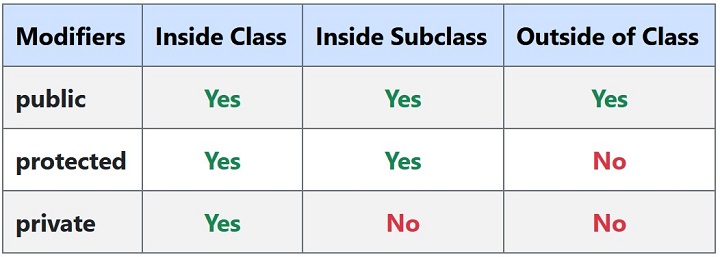