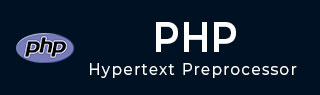
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - File Include
The include statement in PHP is similar to the import statement in Java or Python, and #include directive in C/C++. However, there is a slight difference in the way the include statement works in PHP.
The Java/Python import or #include in C/C++ only loads one or more language constructs such as the functions or classes defined in one file into the current file. In contrast, the include statement in PHP brings in everything in another file into the existing PHP script. It may be a PHP code, a text file, HTML markup, etc.
The "include" Statement in PHP
Here is a typical example of how the include statement works in PHP −
myfile.php
<?php # some PHP code ?>
test.php
<?php include 'myfile.php'; # PHP script in test.php ?>
The include keyword in PHP is very handy, especially when you need to use the same PHP code (function or class) or HTML markup across multiple PHP scripts in a project. A case in point is the creation of a menu that should appear across all pages of a web application.
Suppose you want to create a common menu for your website. Then, create a file "menu.php" with the following content.
<a href="http://www.tutorialspoint.com/index.htm">Home</a> - <a href="http://www.tutorialspoint.com/ebxml">ebXML</a> - <a href="http://www.tutorialspoint.com/ajax">AJAX</a> - <a href="http://www.tutorialspoint.com/perl">PERL</a> <br />
Now create as many pages as you like and include this file to create the header. For example, now your "test.php" file can have the following content −
<html> <body> <?php include("menu.php"); ?> <p>This is an example to show how to include PHP file!</p> </body> </html>
Both the files are assumed to be present in the document root folder of the XAMPP server. Visit http://localhost/test.php URL. It will produce the following output −

When PHP parser encounters the include keyword, it tries to find the specified file in the same directory from which the current script is being executed. If not found, the directories in the "include_path" setting of "php.ini" are searched.
When a file is included, the code it contains inherits the variable scope of the line on which the include occurs. Any variables available at that line in the calling file will be available within the called file, from that point forward. However, all functions and classes defined in the included file have the global scope.
Example
In the following example, we have a "myname.php" script with two variables declared in it. It is included in another script test.php. The variables are loaded in the global scope.
myname.php
<?php $color = 'green'; $fruit = 'apple'; ?>
test.php
<?php include "myname.php"; echo "<h2>$fname $lname</h2>"; ?>
When the browser visits http://localhost/test.php, it shows −
Ravi Teja
However, if the file is included inside a function, the variables are a part of the local scope of the function only.
myname.php
<?php $color = 'green'; $fruit = 'apple'; ?>
test.php
<?php function showname() { include "myname.php"; } echo "<h2>$fname $lname</h2>"; ?>
Now when the browser visits http://localhost/test.php, it shows undefined variable warnings −
Warning: Undefined variable $fname in C:\xampp\htdocs\test.php on line 7 Warning: Undefined variable $lname in C:\xampp\htdocs\test.php on line 7
include_once statement
Just like include, PHP also has the "include_once" keyword. The only difference is that if the code from a file has already been included, it will not be included again, and "include_once" returns true. As the name suggests, the file will be included just once.
"include_once" may be used in cases where the same file might be included and evaluated more than once during a particular execution of a script, so it can help avoid problems such as function redefinitions, variable value reassignments, etc.
PHP – Include vs Require
The require keyword in PHP is quite similar to the include keyword. The difference between the two is that, upon failure require will produce a fatal E_COMPILE_ERROR level error.
In other words, require will halt the script, whereas include only emits a warning (E_WARNING) which allows the script to continue.
require_once keyword
The "require_once" keyword is similar to require with a subtle difference. If you are using "require_once", then PHP will check if the file has already been included, and if so, then the same file it will not be included again.