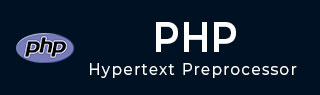
- PHP - Home
- PHP - Roadmap
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP - Operators
- PHP - Arithmetic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
PHP - Class/Object get_class_vars() Function
The PHP Class/Object get_class_vars() function is used to get the default properties (variables) of a class. It gives you access to static properties and default values without the need to create the class. This function is useful for checking class properties.
Syntax
Below is the syntax of the PHP Class/Object get_class_vars() function −
array get_class_vars ( string $class )
Parameters
This function accepts $class parameter which is the name of the class as a string whose default properties you want to access.
Return Value
The get_class_vars() function returns an associative array of default public properties of the class. The resulting array elements are in the form of varname => value.
PHP Version
First introduced in core PHP 4, the get_class_vars() function continues to function easily in PHP 5, PHP 7, and PHP 8.
Example 1
Here is the first example to show you the usage of the PHP Class/Object get_class_vars() function to get the default properties of a simple class.
<?php // Declare Car class here class Car { public $color = 'red'; public $model = 'Sedan'; } $vars = get_class_vars('Car'); print_r($vars); ?>
Output
Here is the outcome of the following code −
Array ( [color] => red [model] => Sedan )
Example 2
This example shows how to get the default variables of a class House that has many data types using the get_class_vars() function.
<?php // Declare House class here class House { public $rooms = 3; public $price = 2500000; public $location = 'Mumbai'; } $vars = get_class_vars('House'); print_r($vars); ?>
Output
This will generate the below output −
Array ( [rooms] => 3 [price] => 2500000 [location] => Mumbai )
Example 3
This example shows that get_class_vars() function only returns public properties and it will not return the private or protected ones.
<?php // Declare Person class here class Person { public $name = 'Amit'; private $age = 33; protected $gender = 'Male'; } $vars = get_class_vars('Person'); print_r($vars); ?>
Output
This will create the below output −
Array ( [name] => Amit )
Example 4
This code creates a class called ExampleClass with many properties, some of which have default values. It uses get_class_vars() to get and print the default values of the class's public properties before they are modified in the constructor.
<?php class ExampleClass { var $property1; // this has no default value... var $property2 = "abc"; var $property3 = 200; private $property4; // constructor function __construct() { // change some properties $this->property1 = "foo"; $this->property2 = "bar"; return true; } } $example_object = new ExampleClass(); $class_vars = get_class_vars(get_class($example_object)); foreach ($class_vars as $name => $value) { echo "$name : $value\n"; } ?>
Output
Following is the output of the above code −
property1 : property2 : abc property3 : 200