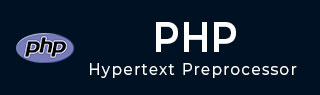
- PHP - Home
- PHP - Roadmap
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP - Operators
- PHP - Arithmetic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
PHP - Class/Object get_class_methods() Function
The PHP Class/Object get_class_methods() function is used to return the method names for a particular class or object in the form of an array. This function is useful for giving a list of all the methods that are available for use with a specific object or class.
Syntax
Below is the syntax of the PHP Class/Object get_class_methods() function −
array get_class_methods( object|string $object_or_class )
Parameters
This function accepts $object_or_class parameter which is the class name or an object instance.
Return Value
The get_class_methods() function returns an array of method names defined for the class specified by class_name. In case of an error, it returns NULL.
PHP Version
First introduced in core PHP 4, the get_class_methods() function continues to function easily in PHP 5, PHP 7, and PHP 8.
Example 1
First we will show you the basic example of the PHP Class/Object get_class_methods() function to get methods from a simple class. The function only returns public functions, so method3 will not be included as it is a private method.
<?php // Declare MyClass here class MyClass { public function method1() {} public function method2() {} private function method3() {} } $methods = get_class_methods('MyClass'); print_r($methods); ?>
Output
Here is the outcome of the following code −
Array ( [0] => method1 [1] => method2 )
Example 2
In the below PHP code we will try to use the get_class_methods() function and get methods from an object instance.
<?php // Declare MyClass here class MyClass { public function signUp() {} public function signIn() {} } $obj = new MyClass(); $methods = get_class_methods($obj); print_r($methods); ?>
Output
This will generate the below output −
Array ( [0] => signUp [1] => signIn )
Example 3
This example demonstrates how get_class_methods() function works and also get methods inherited from a parent class.
<?php // Declare ParentClass here class ParentClass { public function parentMethod() {} } // Declare ChildClass here class ChildClass extends ParentClass { public function childMethod() {} } $methods = get_class_methods('ChildClass'); print_r($methods); ?>
Output
This will create the below output −
Array ( [0] => childMethod [1] => parentMethod )
Example 4
This code creates a class Myclass with a constructor and two methods (function1 and function2). It uses get_class_methods() to get and print the names of all methods in the class.
<?php // Declare Myclass here class Myclass { // constructor function __construct() { return(true); } // Declare function 1 function function1() { return(true); } // Declare function 2 function function2() { return(true); } } $class_methods = get_class_methods(new myclass()); foreach ($class_methods as $method_name) { echo "$method_name\n"; } ?>
Output
Following is the output of the above code −
__construct myfunc1 myfunc2