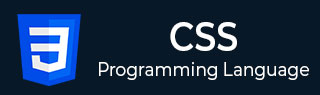
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - grid Property
CSS grid property is a shorthand property used to declare all explicit and implicit grid properties in one declaration.
It's a convenient and concise way to define the grid layout of an element. The grid property is a shorthand for the following individual grid-related properties:
grid-gap (or grid-row-gap and grid-column-gap)
Possible Values
<grid-template> − Works the same as the grid template shorthand.
<grid-template-rows> / [ auto-flow && dense? ] <grid-auto-columns> − Sets grid-template-rows to the specified value (auto-flow or dense). If the auto-flow keyword is to the right of the slash, it sets grid-auto-flow to column. If the dense keyword is specified additionally, the auto-placement algorithm uses a "dense" packing algorithm. If grid-auto-columns is omitted, it is set to auto.
[ auto-flow && dense? ] <grid-auto-rows>? / <grid-template-columns> − Sets grid-auto-columns to the specified value. If the auto-flow keyword is to the left of the slash, it sets grid-auto-flow to row. If the dense keyword is specified additionally, the auto-placement algorithm uses a “dense” packing algorithm. If grid-auto-rows is omitted, it is set to auto.
Applies to
All the HTML elements.
DOM Syntax
object.style.grid = "<grid-template>|<grid-template-rows> / [ auto-flow && dense? ] <grid-auto-columns>| [ auto-flow && dense? ] <grid-auto-rows>? / <grid-template-columns>";
CSS grid - <grid-template>
The following example demonstrates that a grid set as grid: 100px/ 200px, has the first row 100px high and columns 200px wide −
<html> <head> <style> .grid-box { display: grid; grid: 100px / 200px; gap: 10px; } .grid-box > div { background-color: red; border: 3px solid lightgreen; padding: 10px; text-align: center; } </style> </head> <body> <div class="grid-box"> <div>1</div> <div>2</div> <div>3</div> <div>4</div> </div> </body> </html>
CSS grid - minmax() / repeat()
The following example demonstrates that the use of grid: minmax(100px, min-content) / repeat(auto-fill, 50px); property.
minmax(100px, min-content) is used for row sizing. Here, the minmax function defines a minimum size of 100px and a maximum size dependent on the content.
repeat(auto-fill, 50px) is used for column sizing. The repeat function repeats the pattern for grid tracks. It uses auto-fill to create as many columns as possible within the available area, with each column having a fixed size of 50px.
<html> <head> <style> .grid-container { display: grid; grid: minmax(100px, min-content) / repeat(auto-fill, 50px); color: white; text-align: center; width: 300px; } .grid-container > div { background-color: red; border: 2px solid lightgreen; margin: 5px; } </style> </head> <body> <div class="grid-container"> <div>Grid item 1</div> <div>Grid item 2</div> <div>Grid item 3</div> <div>Grid item 4</div> <div>Grid item 5</div> <div>Grid item 6</div> </div> </body> </html>
CSS grid - auto-flow Value
The following example demonstrates that the use of the grid: auto-flow / 200px property sets row size to auto-flow, which automatically sizes the height of the row and set columns width to 200px −
<html> <head> <style> .grid-container { display: grid; grid: auto-flow / 200px; color: white; width: 300px; border: 2px solid lightgreen; } .grid-container > div { background-color: red; border: 2px solid lightgreen; padding: 10px; } </style> </head> <body> <div class="grid-container"> <div>Grid item 1</div> <div>Grid item 2</div> <div>Grid item 3</div> <div>Grid item 4</div> <div>Grid item 5</div> <div>Grid item 6</div> </div> </body> </html>
CSS grid - auto-flow dense Value
The following example demonstrates the use of grid: 100px 200px / auto-flow dense property. It specifies two rows with heights of 100px and 200px.
The columns are set to auto-flow dense, which automatically place items in the grid and dense, to fit as many grid elements as feasible without gaps.
<html> <head> <style> .grid-container { display: grid; grid: 100px 200px / auto-flow dense; color: white; width: 300px; border: 2px solid lightgreen; } .grid-container > div { background-color: red; border: 2px solid lightgreen; padding: 10px; } </style> </head> <body> <div class="grid-container"> <div>Grid item 1</div> <div>Grid item 2</div> <div>Grid item 3</div> <div>Grid item 4</div> <div>Grid item 5</div> <div>Grid item 6</div> </div> </body> </html>
CSS grid - [ auto-flow && dense? ] <grid-auto-rows>? / <grid-template-columns>
The following example demonstrates that the grid: auto-flow 50px / repeat(3, 150px) property sets the rows to auto-flow 50px, which automatically places items in the grid with row height set to 50px.
The repeat(3, 150px) specifies that there should be three columns, each 150px wide.
<html> <head> <style> .grid-container { display: grid; grid: auto-flow 50px / repeat(3, 150px); color: white; width: 300px; border: 2px solid lightgreen; } .grid-container > div { background-color: red; border: 2px solid lightgreen; padding: 10px; } </style> </head> <div class="grid-container"> <div>Grid item 1</div> <div>Grid item 2</div> <div>Grid item 3</div> <div>Grid item 4</div> <div>Grid item 5</div> <div>Grid item 6</div> </div> </body> </html>
CSS grid - Related Properties
Following is the list of CSS properties related to grid:
property | value |
---|---|
display | Define whether an element is a grid container or an inline grid container. |
gap | Defines the gap between rows and columns. |
grid-area | Defines the location and size of the grid item within a grid layout. |
grid-column | Control the placement of a grid item within the grid container in the column direction. |
grid-row | Control the placement of a grid item within the grid container in the row direction. |
grid-template | Specify the grid columns, grid rows and grid areas. |
grid-auto-columns | Determines the size of automatically generated grid column tracks or a pattern of such tracks. |
grid-auto-rows | Determines the size of automatically- generated grid rows tracks or a pattern of such tracks. |
grid-auto-flow | Specifies arrangement of the grid item within the grid. |