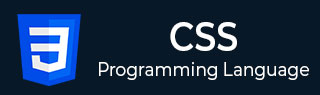
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS RWD Images
Responsive images are important for the web development, as they ensure that the images are appropriately sized based on the device size and resolution. The responsive images improve the page loading speed and also reduce the time to load it.
When an image is uploaded to a website, it has its default width and height; but these dimensions can be changed using CSS. In order to make an image responsive and fluid, you need to provide a new value to its width property, to which the height of the image gets adjusted automatically.
For better adaptability, you should always use relative units for the width property such as percentage, instead of absolute values, such as pixels. Absolute values restrict the image getting responsive.
CSS RWD Images - width Property
To make an image responsive and fluid, the width property of the image is set to a percentage value, along with height property set to auto.
<html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> img { width: 100%; height: auto; } </style> </head> <body> <p>Resize the browser window to see how the image will resize.</p> <img src="images/pink-flower.jpg"> </body> </html>
CSS RWD Images - max-width Property
To make an image responsive and fluid, the max-width property of the image is set to a 100%, which will let the image get scaled down to the extent it is set, but will never scale up more than its original size.
<html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> img { max-width: 100%; height: auto; } </style> </head> <body> <p>Resize the browser window to see how the size of the image changes.</p> <img src="images/pink-flower.jpg"> </body> </html>
CSS RWD Images - Within a grid
The following example demonstrates the use of a responsive image within a grid column. Based on the max-width value of the image, the image gets scaled down as per the screen size.
<html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> * { box-sizing: border-box; } .title { border: 2px solid black; padding: 10px; background-color: blanchedalmond; } .grid-one { width: 60%; float: left; padding: 10px; border: 2px solid black; background-color: darkseagreen; } .grid-two { width: 40%; float: left; padding: 15px; border: 2px solid black; background-color: lightgreen; } ul { list-style-type: none; } li { background-color: aqua; padding: 5px; border: 1px solid black; margin: 5px; } img { max-width: 100%; height: auto; } </style> </head> <body> <div class="title"> <h1>Responsive Web Design</h1> </div> <div class="grid-two"> <ul> <li>Viewport</li> <li>Grid view</li> <li>Media queries</li> <li>Images</li> <li>Videos</li> <li>Frameworks</li> </ul> </div> <div class="grid-one"> <h1>Responsive Images</h1> <p>Responsive images are important for the web development, as they ensure that the images are appropriately sized based on the device size and resolution. The responsive images improve the page loading speed and also reduce the time to load it.</p> <img src="images/scenery2.jpg"> <p>Resize the browser window to see how the content gets responsive to the resizing.</p> </div> </body> </html>
CSS RWD Images - Using Background Images
The background images can also be resized or scaled based on the property background-size: contain. This property will scale the image, and tries to fit the content area. The aspect ratio of the image will be maintained.
<html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> div { width: 100%; height: 400px; background-image: url(images/pink-flower.jpg); background-repeat: no-repeat; background-size: contain; border: 5px solid black; background-color: antiquewhite; } </style> </head> <body> <h1>Responsive Web Design - Images</h1> <div></div> </body> </html>
The background image can also be resized or scaled based on the property background-size: cover. This property will scale the image such that it covers the entire content area. The aspect ratio of the image will be maintained, but some parts of the background image may be clipped.
<html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> div { width: 100%; height: 80%; background-image: url(images/pink-flower.jpg); background-repeat: no-repeat; background-size: cover; border: 5px solid red; background-color: antiquewhite; } </style> </head> <body> <h1>Responsive Web Design - Images</h1> <div></div> </body> </html>
The background image can also be resized or scaled based on the property background-size: 100% 100%. This value will make the image get stretched to cover the entire content area.
<html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> div { width: 100%; height: 80%; background-image: url(images/pink-flower.jpg); background-repeat: no-repeat; background-size: 100% 100%; border: 5px solid red; background-color: antiquewhite; } </style> </head> <body> <h1>Responsive Web Design - Images</h1> <div></div> </body> </html>
CSS RWD Images - Using Media Query
When you need an image to be displayed in different sizes on different devices, you need to use a media query. The example below shows an image whose width is 50% for one kind of screen, but in order to make it full-size for a mobile device a media query will be used.
<html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> /* For width smaller than 400px: */ body { background-repeat: no-repeat; background-image: url(images/orange-flower.jpg); } /* For width 400px and larger: */ @media only screen and (min-width: 700px) { body { background-image: url(images/pink-flower.jpg); background-size: cover; } } </style> </head> <body> <p style="margin-top:360px;color:white;">Resize the browser width and the background image will change at 400px.</p> </body> </html>