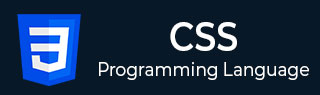
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Align
The term alignment, in context of web design and CSS, refers to the positioning and arrangement of elements or content within a layout, typically with respect to specific guidelines or reference points. Alignment is used to create visually pleasing and organized designs by ensuring that elements are positioned relative to each other or to the layout structure in a consistent and harmonious way.
Alignment can be applied to various types of elements, including text, images, buttons, and more, to create a cohesive and polished design. CSS provides various properties that can be used to align the elements.
There are two main aspects of alignment:
Horizontal alignment: This refers to the positioning of elements along the horizontal axis, which typically runs from left to right. Common horizontal alignment options include:
Left alignment: Elements are aligned to the left side of a container or layout.
Center alignment: Elements are positioned in the center of a container or layout horizontally.
Right alignment: Elements are aligned to the right side of a container or layout.
Vertical alignment: This refers to the positioning of elements along the vertical axis, which typically runs from top to bottom. Common vertical alignment options include:
Top alignment: Elements are aligned to the top of a container or layout.
Middle or Center alignment: Elements are centered vertically within a container or layout.
Bottom alignment: Elements are aligned to the bottom of a container or layout.
CSS Align - position Property
There are several ways to align an element to left. Let us see few ways to achieve this.
With the use of the CSS property position, the alignment of elements can be adjusted.
Here is an example of setting alignment using position:
<html> <head> <style> .right-alignment { position: absolute; right: 0px; width: 100px; border: 3px solid #0d1601; background-color: rgb(244, 244, 135); padding: 10px; } .left-alignment { position: relative; left: 0px; width: 100px; border: 3px solid #0c1401; background-color: blanchedalmond; padding: 10px; } .center-alignment { margin: auto; border: 3px solid black; padding: 5px; background-color: rgb(241, 97, 126); width: 120px; position: relative; } </style> </head> <body> <div class="right-alignment"> <h3>Right align</h3> <strong>Right align with position:absolute</strong> </div> <div class="left-alignment"> <h3>Left align</h3> <strong>Left align with position:relative</strong> </div> <div class="center-alignment"> <h3>Center align</h3> <strong>Vertically & horizontally centered using position:relative and margin:auto.</strong> </div> </body> </html>
Note: Absolute positioned elements are removed from the normal flow, and can overlap elements.
CSS Align - float Property
With the use of the CSS property float, the alignment of elements can be adjusted.
Here is an example of setting alignment using float:
<html> <head> <style> .right-alignment { float: right; width: 100px; border: 3px solid #0d1601; background-color: rgb(244, 244, 135); padding: 10px; } .left-alignment { float: left; left: 0px; width: 100px; border: 3px solid #0c1401; background-color: blanchedalmond; padding: 10px; } </style> </head> <body> <div class="right-alignment"> <h3>Right align</h3> <strong>Right align with float:right</strong> </div> <div class="left-alignment"> <h3>Left align</h3> <strong>Left align with float:left</strong> </div> </body> </html>
CSS Align - text-align Property
To align the text inside an element, use the property text-align.
Here is an example to align the text inside a <div> element:
<html> <head> <style> div { width: 300px; border: 3px solid #0d1601; padding: 10px; margin-bottom: 1cm; } .right-alignment { text-align: right; color:red; } .left-alignment { text-align:left; color:green; } .center-alignment { text-align: center; color:blue; } </style> </head> <body> <div class="right-alignment"> <h3>Right align</h3> <strong>Text right aligned</strong> </div> <div class="left-alignment"> <h3>Left align</h3> <strong>Text left aligned</strong> </div> <div class="center-alignment"> <h3>Center align</h3> <strong>Text center aligned</strong> </div> </body> </html>
CSS Align - padding Property
A piece of text can be centered vertically using padding CSS property.
<html> <head> <style> .center-alignment { padding: 100px 0; border: 3px solid black; margin: 5px; background-color: lightblue; } </style> </head> <body> <div class="center-alignment"> <p>Vertically centerd using padding.</p> </div> </body> </html>
CSS Align - Center Text
Here is an example if you want to center the text vertically as well as horizontally, you need to use text-align:center along with padding:
<html> <head> <style> .center-alignment { padding: 100px 0; text-align: center; border: 3px solid black; margin: 5px; background-color: lightblue; } </style> </head> <body> <div class="center-alignment"> <p>Vertically & horizontally centerd using padding and text-align properties.</p> </div> </body> </html>
CSS Align - justify-content Property
Here is an example if you want to center the text vertically as well as horizontally, using flex and justify-content properties:
<html> <head> <style> .center-alignment { display: flex; justify-content: center; align-items: center; height: 300px; border: 3px solid black; font-size: larger; background-color: lightblue; } </style> </head> <body> <div class="center-alignment"> <p>Vertically & horizontally centered using flex and justify-content.</p> </div> </body> </html>
CSS Align - Related Properties
Following table lists all the properties related to alignment:
Property | Description |
---|---|
align-content | Aligns the content of a flex container along the cross-axis or a grid's block axis. |
align-items | Controls the alignment of items of a flex container along the cross-axis. |
align-self | Controls the alignment of an individual item within a container. |
vertical-align | Determines the vertical alignment of inline, inline-block or a table cell text. |
line-height | Sets the distance between lines of text. |
text-align | Sets the horizontal alignment of inline, inline-block or a table cell text. |
margin | Shorthand for margin values that can modify the alignment. |