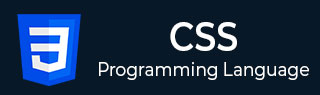
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS grid - grid-template-areas
The CSS property grid-template-areas defines named sections within a grid that outline the arrangement of the cells and identify them with specific names.
These sections aren't specifically linked to individual grid elements but can be accessed via the grid-placement properties grid-row-start, grid-row-end, grid-column-start, grid-column-end and their shorthand forms- grid-row, grid-column, and grid-area.
Possible values
The grid container doesn't contain any named grid regions.
A row is created for each unique string listed, while the cells of each string form individual columns.
If multiple cell tokens in multiple rows have the same name, they're combined into a single-named grid region that spans those specific grid cells.
However, if these cells don't form a rectangular shape, the declaration becomes invalid.
Syntax
grid-template-areas: none | <string> ;
Applies to
Grid containers.
CSS grid-template-areas - Named areas
The following example demonstrates defining named areas using grid-template-areas.
In the example given, the grid-template-areas property defines the layout structure by assigning named areas to certain grid cells.
Each character string in the value of the property represents a row, and individual character strings separated by spaces designate columns.
By assigning names to these areas, the grid layout is organized so that content can be placed in each named section accordingly.
<html> <head> <style> /* Define styles for the grid container */ .grid-container { display: grid; grid-template-columns: repeat(5, 1fr); /* Five columns of equal width */ grid-template-rows: 100px 200px 150px 100px; /* Four rows of different heights */ grid-template-areas: "header header header main extra" "sidebar content content main extra" "sidebar content content main extra" "footer footer footer main extra"; gap: 10px; font-size: 1.5em; height: 600px; /* Set a fixed height for demonstration */ border: 1px solid #ccc; padding: 10px; } /* Define styles for the grid items */ .header { grid-area: header; background-color: #3498db; color: white; display: flex; align-items: center; justify-content: center; } .sidebar { grid-area: sidebar; background-color: #2ecc71; color: white; display: flex; align-items: center; justify-content: center; } .content { grid-area: content; background-color: #e74c3c; color: white; display: flex; align-items: center; justify-content: center; } .footer { grid-area: footer; background-color: #f39c12; color: white; display: flex; align-items: center; justify-content: center; } .main { grid-area: main; background-color: #9b59b6; color: white; display: flex; align-items: center; justify-content: center; } .extra { grid-area: extra; background-color: #34495e; color: white; display: flex; align-items: center; justify-content: center; } </style> </head> <body> <div class="grid-container"> <div class="header">Header</div> <div class="sidebar">Sidebar</div> <div class="content">Content</div> <div class="footer">Footer</div> <div class="main">Main</div> <div class="extra">Extra</div> </div> </body> </html>
CSS grid-template-areas - Web Page Layout
In the following example the grid-template-areas attribute defines named grid areas for every layout segment.
The grid-template-areas allocates the header, article, navigation, advertisement, and footer within the grid, resulting in an organized web page layout.
<html> <head> <style> body { display: grid; grid-template-areas: "header header header" "nav article article" "ads ads footer"; grid-template-rows: 80px 1fr 70px; grid-template-columns: 20% 1fr 15%; grid-gap: 10px; height: 100vh; margin: 0; font-size:20px; } header, footer, article, nav, div { padding: 20px; background: #3498DB; color: #fff; } #pageHeader { grid-area: header; } #pageFooter { grid-area: footer; } #mainArticle { grid-area: article; } #mainNav { grid-area: nav; } #siteAds { grid-area: ads; } </style> </head> <body> <header id="pageHeader">Custom Header</header> <article id="mainArticle">Main Content</article> <nav id="mainNav">Navigation</nav> <div id="siteAds">Advertisement</div> <footer id="pageFooter">Custom Footer</footer> </body> </html>
To Continue Learning Please Login