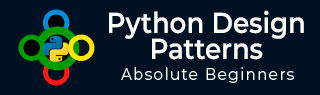
- Python Design Patterns Tutorial
- Python Design Patterns - Home
- Introduction
- Python Design Patterns - Gist
- Model View Controller Pattern
- Python Design Patterns - Singleton
- Python Design Patterns - Factory
- Python Design Patterns - Builder
- Python Design Patterns - Prototype
- Python Design Patterns - Facade
- Python Design Patterns - Command
- Python Design Patterns - Adapter
- Python Design Patterns - Decorator
- Python Design Patterns - Proxy
- Chain of Responsibility Pattern
- Python Design Patterns - Observer
- Python Design Patterns - State
- Python Design Patterns - Strategy
- Python Design Patterns - Template
- Python Design Patterns - Flyweight
- Abstract Factory
- Object Oriented
- Object Oriented Concepts Implementation
- Python Design Patterns - Iterator
- Dictionaries
- Lists Data Structure
- Python Design Patterns - Sets
- Python Design Patterns - Queues
- Strings & Serialization
- Concurrency in Python
- Python Design Patterns - Anti
- Exception Handling
- Python Design Patterns Resources
- Quick Guide
- Python Design Patterns - Resources
- Discussions
Object Oriented Concepts Implementation
In this chapter, we will focus on patterns using object oriented concepts and its implementation in Python. When we design our programs around blocks of statements, which manipulate the data around functions, it is called procedure-oriented programming. In object-oriented programming, there are two main instances called classes and objects.
How to implement classes and object variables?
The implementation of classes and object variables are as follows −
class Robot: population = 0 def __init__(self, name): self.name = name print("(Initializing {})".format(self.name)) Robot.population += 1 def die(self): print("{} is being destroyed!".format(self.name)) Robot.population -= 1 if Robot.population == 0: print("{} was the last one.".format(self.name)) else: print("There are still {:d} robots working.".format( Robot.population)) def say_hi(self): print("Greetings, my masters call me {}.".format(self.name)) @classmethod def how_many(cls): print("We have {:d} robots.".format(cls.population)) droid1 = Robot("R2-D2") droid1.say_hi() Robot.how_many() droid2 = Robot("C-3PO") droid2.say_hi() Robot.how_many() print("\nRobots can do some work here.\n") print("Robots have finished their work. So let's destroy them.") droid1.die() droid2.die() Robot.how_many()
Output
The above program generates the following output −
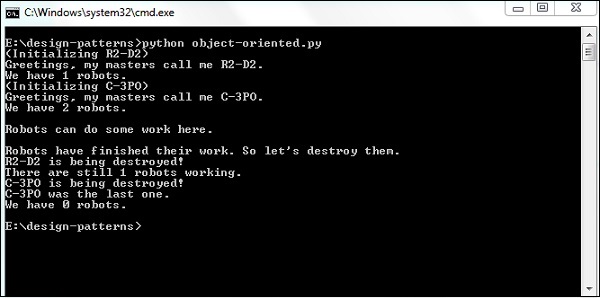
Explanation
This illustration helps to demonstrate the nature of class and object variables.
“population” belongs to the “Robot” class. Hence, it is referred to as a class variable or object.
Here, we refer to the population class variable as Robot.population and not as self.population.
Advertisements