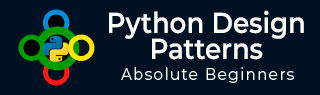
- Python Design Patterns Tutorial
- Python Design Patterns - Home
- Introduction
- Python Design Patterns - Gist
- Model View Controller Pattern
- Python Design Patterns - Singleton
- Python Design Patterns - Factory
- Python Design Patterns - Builder
- Python Design Patterns - Prototype
- Python Design Patterns - Facade
- Python Design Patterns - Command
- Python Design Patterns - Adapter
- Python Design Patterns - Decorator
- Python Design Patterns - Proxy
- Chain of Responsibility Pattern
- Python Design Patterns - Observer
- Python Design Patterns - State
- Python Design Patterns - Strategy
- Python Design Patterns - Template
- Python Design Patterns - Flyweight
- Abstract Factory
- Object Oriented
- Object Oriented Concepts Implementation
- Python Design Patterns - Iterator
- Dictionaries
- Lists Data Structure
- Python Design Patterns - Sets
- Python Design Patterns - Queues
- Strings & Serialization
- Concurrency in Python
- Python Design Patterns - Anti
- Exception Handling
- Python Design Patterns Resources
- Quick Guide
- Python Design Patterns - Resources
- Discussions
Python Design Patterns - Command
Command Pattern adds a level of abstraction between actions and includes an object, which invokes these actions.
In this design pattern, client creates a command object that includes a list of commands to be executed. The command object created implements a specific interface.
Following is the basic architecture of the command pattern −
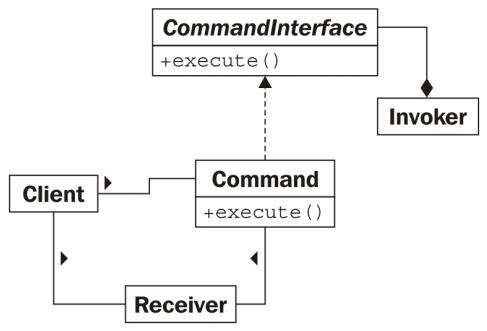
How to implement the command pattern?
We will now see how to implement the design pattern.
def demo(a,b,c): print 'a:',a print 'b:',b print 'c:',c class Command: def __init__(self, cmd, *args): self._cmd=cmd self._args=args def __call__(self, *args): return apply(self._cmd, self._args+args) cmd = Command(dir,__builtins__) print cmd() cmd = Command(demo,1,2) cmd(3)
Output
The above program generates the following output −
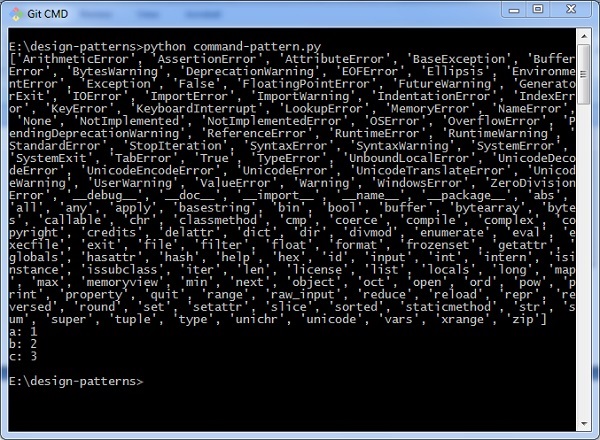
Explanation
The output implements all the commands and keywords listed in Python language. It prints the necessary values of the variables.
Advertisements