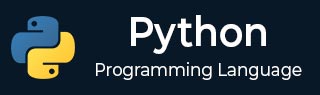
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Translation Methods
maketrans()
The maketrans() method returns a mapping table. It maps each character from astr to a character at same index in bstr. The mapping table returned by this method may be used by translate() method to replace the characters.
Syntax
var.maketrans(astr, bstr, cstr)
Parameters
astr − This can be either dictionary or string. If only one parameter is supplied, this parameter must be a dictionary. If two or more parameters are given, this parameter has to be a string specifying the characters to be replaced.
bstr − This is the string having corresponding mapping character.
cstr − Optional. The string parameter specifies the characters to remove in the string.
Return Value
This method returns a translate table to be used translate() function.
Example
var = 'Explicit is better than implicit.' table = var.maketrans({'i':'I'}) print ("original string:", var) print ("translation table:", table) var1 = var.translate(table) print ("Translated string", var1) var = "Explicit is better than implicit." table = var.maketrans("than", "then") print ("original string:", var) print ("translation table:", table) var2 = var.translate(table) print ("Translated string", var2) var = 'Explicit is better than implicit.' table = var.maketrans("is","as", "s") print ("original string:", var) print ("translation table:", table) var3=var.translate(table) print ("Translated string", var3)
When you run this program, it will produce the following output −
original string: Explicit is better than implicit. translation table: {105: 'I'} Translated string ExplIcIt Is better than ImplIcIt. original string: Explicit is better than implicit. translation table: {116: 116, 104: 104, 97: 101, 110: 110} Translated string Explicit is better then implicit. original string: Explicit is better than implicit. translation table: {105: 97, 115: None} Translated string Explacat a better than amplacat.
translate()
The translate() method returns a string where each character is replaced by its corresponding character in the translation table created by the maketrans() method.
Syntax
var.translate(table)
Parameters
table − A translation table created by the maketrans() method.
Return Value
This method returns a translated copy of the string.
Example
var = 'Explicit is better than implicit.' table = var.maketrans({'i':'I'}) print ("original string:", var) print ("translation table:", table) var1 = var.translate(table) print ("Translated string", var1) var = "Explicit is better than implicit." table = var.maketrans("than", "then") print ("original string:", var) print ("translation table:", table) var2 = var.translate(table) print ("Translated string", var2) var = 'Explicit is better than implicit.' table = var.maketrans("is","as", "s") print ("original string:", var) print ("translation table:", table) var3=var.translate(table) print ("Translated string", var3)
When you run this program, it will produce the following output −
original string: Explicit is better than implicit. translation table: {105: 'I'} Translated string ExplIcIt Is better than ImplIcIt. original string: Explicit is better than implicit. translation table: {116: 116, 104: 104, 97: 101, 110: 110} Translated string Explicit is better then implicit. original string: Explicit is better than implicit. translation table: {105: 97, 115: None} Translated string Explacat a better than amplacat.