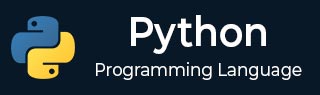
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Arrays
Array is a container which can hold a fix number of items and these items should be of the same type. Most of the data structures make use of arrays to implement their algorithms. Following are the important terms to understand the concept of Array.
- Element− Each item stored in an array is called an element.
- Index − Each location of an element in an array has a numerical index, which is used to identify the element.
Array Representation
Arrays can be declared in various ways in different languages. Below is an illustration.
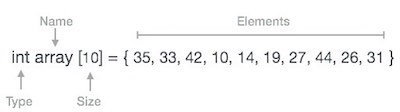
As per the above illustration, following are the important points to be considered.
Index starts with 0.
Array length is 10 which means it can store 10 elements.
Each element can be accessed via its index. For example, we can fetch an element at index 6 as 9.
Basic Operations
Following are the basic operations supported by an array.
Traverse − Print all the array elements one by one.
Insertion − Adds an element at the given index.
Deletion − Deletes an element at the given index.
Search − Searches an element using the given index or by the value.
Update − Updates an element at the given index.
Arrays in Python
Python's standard data types list, tuple and string are sequences. A sequence object is an ordered collection of items. Each item is characterized by incrementing index starting with zero. Moreover, items in a sequence need not be of same type. In other words, a list or tuple may consist of items of different data type.
This feature is different from the concept of an array in C or C++. In C/C++, an array is also an indexed collection of items, but the items must be of similar data type. In C/C++, you have an array of integers or floats, or strings, but you cannot have an array with some elements of integer type and some of different type. A C/C++ array is therefore a homogenous collection of data types.
Python's standard library has array module. The array class in it allows you to construct an array of three basic types, integer, float and Unicode characters.
Syntax
The syntax of creating array is −
import array obj = array.array(typecode[, initializer])
Parameters
typecode − The typecode character used to create the array.
initializer − array initialized from the optional value, which must be a list, a bytes-like object, or iterable over elements of the appropriate type.
Return type
The array() constructor returns an object of array.array class
Example
import array as arr # creating an array with integer type a = arr.array('i', [1, 2, 3]) print (type(a), a) # creating an array with char type a = arr.array('u', 'BAT') print (type(a), a) # creating an array with float type a = arr.array('d', [1.1, 2.2, 3.3]) print (type(a), a)
It will produce the following output −
<class 'array.array'> array('i', [1, 2, 3]) <class 'array.array'> array('u', 'BAT') <class 'array.array'> array('d', [1.1, 2.2, 3.3])
Arrays are sequence types and behave very much like lists, except that the type of objects stored in them is constrained.
Python array type is decided by a single character Typecode argument. The type codes and the intended data type of array is listed below −
typecode | Python data type | Byte size |
---|---|---|
'b' | signed integer | 1 |
'B' | unsigned integer | 1 |
'u' | Unicode character | 2 |
'h' | signed integer | 2 |
'H' | unsigned integer | 2 |
'i' | signed integer | 2 |
'I' | unsigned integer | 2 |
'l' | signed integer | 4 |
'L' | unsigned integer | 4 |
'q' | signed integer | 8 |
'Q' | unsigned integer | 8 |
'f' | floating point | 4 |
'd' | floating point | 8 |
Before looking at various array operations lets create and print an array using python. The below code creates an array named array1.
from array import * array1 = array('i', [10,20,30,40,50]) for x in array1: print(x)
Output
When we compile and execute the above program, it produces the following result −
10 20 30 40 50
Accessing Array Element
We can access each element of an array using the index of the element. The below code shows how
from array import * array1 = array('i', [10,20,30,40,50]) print (array1[0]) print (array1[2])
Output
When we compile and execute the above program, it produces the following result − which shows the element is inserted at index position 1.
10 30
Insertion Operation
Insert operation is to insert one or more data elements into an array. Based on the requirement, a new element can be added at the beginning, end, or any given index of array.
Here, we add a data element at the middle of the array using the python in-built insert() method.
from array import * array1 = array('i', [10,20,30,40,50]) array1.insert(1,60) for x in array1: print(x)
Output
When we compile and execute the above program, it produces the following result which shows the element is inserted at index position 1.
10 60 20 30 40 50
Deletion Operation
Deletion refers to removing an existing element from the array and re-organizing all elements of an array.
Here, we remove a data element at the middle of the array using the python in-built remove() method.
from array import * array1 = array('i', [10,20,30,40,50]) array1.remove(40) for x in array1: print(x)
Output
When we compile and execute the above program, it produces the following result which shows the element is removed form the array.
10 20 30 50
Search Operation
You can perform a search for an array element based on its value or its index. Here, we search a data element using the python in-built index() method.
from array import * array1 = array('i', [10,20,30,40,50]) print (array1.index(40))
Output
When we compile and execute the above program, it produces the following result which shows the index of the element. If the value is not present in the array then the program returns an error.
3
Update Operation
Update operation refers to updating an existing element from the array at a given index. Here, we simply reassign a new value to the desired index we want to update.
from array import * array1 = array('i', [10,20,30,40,50]) array1[2] = 80 for x in array1: print(x)
Output
When we compile and execute the above program, it produces the following result which shows the new value at the index position 2.
10 20 80 40 50