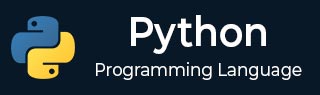
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - List Comprehension
List Comprehension in Python
A list comprehension is a concise way to create lists. It is similar to set builder notation in mathematics. It is used to define a list based on an existing iterable object, such as a list, tuple, or string, and apply an expression to each element in the iterable.
Syntax of Python List Comprehension
The basic syntax of list comprehension is −
new_list = [expression for item in iterable if condition]
Where,
- expression is the operation or transformation to apply to each item in the iterable.
- item is the variable representing each element in the iterable.
- iterable is the sequence of elements to iterate over.
- condition (optional) is an expression that filters elements based on a specified condition.
Example of Python List Comprehension
Suppose we want to convert all the letters in the string "hello world" to their upper-case form. Using list comprehension, we iterate through each character, check if it is a letter, and if so, convert it to uppercase, resulting in a list of uppercase letters −
string = "hello world" uppercase_letters = [char.upper() for char in string if char.isalpha()] print(uppercase_letters)
The result obtained is displayed as follows −
['H', 'E', 'L', 'L', 'O', 'W', 'O', 'R', 'L', 'D']
List Comprehensions and Lambda
In Python, lambda is a keyword used to create anonymous functions. An anonymous function is a function defined without a name. These functions are created using the lambda keyword followed by a comma-separated list of arguments, followed by a colon :, and then the expression to be evaluated.
We can use list comprehension with lambda by applying the lambda function to each element of an iterable within the comprehension, generating a new list.
Example
In the following example, we are using list comprehension with a lambda function to double each element in a given list "original_list". We iterate over each element in the "original_list" and apply the lambda function to double it −
original_list = [1, 2, 3, 4, 5] doubled_list = [(lambda x: x * 2)(x) for x in original_list] print(doubled_list)
Following is the output of the above code −
[2, 4, 6, 8, 10]
Nested Loops in Python List Comprehension
A nested loop in Python is a loop inside another loop, where the inner loop is executed multiple times for each iteration of the outer loop.
We can use nested loops in list comprehension by placing one loop inside another, allowing for concise creation of lists from multiple iterations.
Example
In this example, all combinations of items from two lists in the form of a tuple are added in a third list object −
list1=[1,2,3] list2=[4,5,6] CombLst=[(x,y) for x in list1 for y in list2] print (CombLst)
It will produce the following output −
[(1, 4), (1, 5), (1, 6), (2, 4), (2, 5), (2, 6), (3, 4), (3, 5), (3, 6)]
Conditionals in Python List Comprehension
Conditionals in Python refer to the use of statements like "if", "elif", and "else" to control the flow of a code based on certain conditions. They allow you to execute different blocks of code depending on whether a condition evaluates to "True" or "False".
We can use conditionals in list comprehension by including them after the iterable and before the loop, which filters elements from the iterable based on the specified condition while generating the list.
Example
The following example uses conditionals within a list comprehension to generate a list of even numbers from 1 to 20 −
list1=[x for x in range(1,21) if x%2==0] print (list1)
We get the output as follows −
[2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
List Comprehensions vs For Loop
List comprehensions and for loops are both used for iteration, but they differ in terms of syntax and usage.
List comprehensions are like shortcuts for creating lists in Python. They let you generate a new list by applying an operation to each item in an existing list.
For loop, on the other hand, is a control flow statement used to iterate over elements of an iterable one by one, executing a block of code for each element.
List comprehensions are often preferred for simpler operations, while for loops offer more flexibility for complex tasks.
Example Using For Loop
Suppose we want to separate each letter in a string and put all non-vowel letters in a list object. We can do it by a for loop as shown below −
chars=[] for ch in 'TutorialsPoint': if ch not in 'aeiou': chars.append(ch) print (chars)
The chars list object is displayed as follows −
['T', 't', 'r', 'l', 's', 'P', 'n', 't']
Example Using List Comprehension
We can easily get the same result by a list comprehension technique. A general usage of list comprehension is as follows −
listObj = [x for x in iterable]
Applying this, chars list can be constructed by the following statement −
chars = [ char for char in 'TutorialsPoint' if char not in 'aeiou'] print (chars)
The chars list will be displayed as before −
['T', 't', 'r', 'l', 's', 'P', 'n', 't']
Example
The following example uses list comprehension to build a list of squares of numbers between 1 to 10 −
squares = [x*x for x in range(1,11)] print (squares)
The squares list object is −
[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
Advantages of List Comprehension
Following are the advantages of using list comprehension −
Conciseness − List comprehensions are more concise and readable compared to traditional for loops, allowing you to create lists with less code.
Efficiency − List comprehensions are generally faster and more efficient than for loops because they are optimized internally by Python's interpreter.
Clarity − List comprehensions result in clearer and more expressive code, making it easier to understand the purpose and logic of the operation being performed.
Reduced Chance of Errors − Since list comprehensions are more compact, there is less chance of errors compared to traditional for loops, reducing the likelihood of bugs in your code.