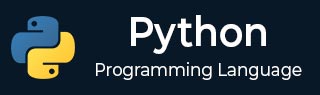
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Python - OS Path Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Classes & Objects
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Advanced Concepts
- Python - Abstract Base Classes
- Python - Custom Exceptions
- Python - Higher Order Functions
- Python - Object Internals
- Python - Memory Management
- Python - Metaclasses
- Python - Metaprogramming with Metaclasses
- Python - Mocking and Stubbing
- Python - Monkey Patching
- Python - Signal Handling
- Python - Type Hints
- Python - Automation Tutorial
- Python - Humanize Package
- Python - Context Managers
- Python - Coroutines
- Python - Descriptors
- Python - Diagnosing and Fixing Memory Leaks
- Python - Immutable Data Structures
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Questions & Answers
- Python - Online Quiz
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Humanize Package
The Humanize Package in Python is a library which is specifically designed to convert numerical values, dates, times and file sizes into formats that are more easily understood by humans.
- This package is essential for creating user-friendly interfaces and readable reports where data interpretation needs to be quick and intuitive.
- The primary goal of the Humanize Package is to bridge the gap between raw data and human understanding.
Although computers and databases excel at processing raw numerical data but these formats can be challenging for humans to quickly grasp. The Humanize Package tackles this issue by converting these data points into more intuitive and user-friendly formats.
Installation of Humanize Package
To install the humanize package in Python we can use pip which is the standard package manager for Python. Following code has to be run in the command line or terminal to install Humanize Package −
pip install humanize
After installation we can verify if humanize is installed correctly by running a Python interpreter and importing the Humanize package by using the below code −
import humanize
Different Utilities in Humanize Package
The Humanize package in Python provides a wide range of utilities that transform data into human-readable formats by enhancing usability and comprehension. Let's explore the different utilities offered by humanize package in detail −
Number Utilities
The Humanize package in Python provides several number of utilities that enhance the readability and comprehension of numerical data. These utilities convert numbers into formats that are more natural and understandable for humans.
Integer Formatting
The Integer Formatting utility converts large integers into strings with commas for improved readability. Following is the example of applying the integer formatting utility −
import humanize print(humanize.intcomma(123456))
Output
123,456
Integer Word Representation
The Integer Word Representation converts large integers into their word representation for easier understanding, especially for very large numbers. Below is the example of it −
import humanize print(humanize.intword(12345678908545944))
Output
12.3 quadrillion
Ordinal Numbers
The Ordinal numbers converts integers into their ordinal form. For example 1 will be given as 1st and 2 as 2nd. Below is the example converting 3 as 3rd −
import humanize print(humanize.ordinal(3))
Output
3rd
AP Numbers
These converts the integers into their corresponding words. Here is the example of it −
import humanize print(humanize.apnumber(9))
Output
nine
Fractional Units
This converts decimal numbers into fractions for more intuitive representation. Following is the example of it −
import humanize print(humanize.fractional(0.5))
Output
1/2
File Size Utilities
As we already know that the humanize package in Python provides several utilities among them File Size Utilities is one which is specifically designed to convert raw byte values into human-readable file sizes.
These utilities help to make file sizes more understandable by converting them into formats that are easier to read and interpret. Here is a detailed overview of the file size utilities available in the humanize package −
File Size Formatting using naturalsize()
The naturalsize() function is the primary utility for converting file sizes into human-readable formats. It automatically selects the appropriate units such as bytes, KB, MB, GB based on the size provided.
Syntax
Following is the syntax of the naturalsize() function of the File Size Utilities of Python Humanize package −
humanize.naturalsize(value,binary,gnu,format)
Parameter
Below are the parameters of the naturalsize() function of the python humanize package −
- value: The file size in bytes.
- binary: A boolean flag to indicate whether to use binary units. The default value is False.
- gnu: A boolean flag to indicate whether to use GNU-style output and the default value is False.
- format: A string to specify the output format. The default value is "%.1f".
Example
Following is the example of using the naturalsize() of humanize package in python −
import humanize # Default usage with decimal units file_size = 123456789 print(f"File size: {humanize.naturalsize(file_size)}") # Using binary units print(f"File size (binary): {humanize.naturalsize(file_size, binary=True)}") # Using GNU-style prefixes print(f"File size (GNU): {humanize.naturalsize(file_size, gnu=True)}") # Custom format print(f"File size (custom format): {humanize.naturalsize(file_size, format='%.2f')}")
Following is the output −
File size: 123.5 MB File size (binary): 117.7 MiB File size (GNU): 117.7M File size (custom format): 123.46 MB
Date Time Utilities
The Humanize package in Python provides several utilities for making dates and times more readable. These utilities transform date-time objects into formats that are easier for humans to understand such as relative times, natural dates and more. Following is the detailed overview of the date and time utilities offered by the humanize package −
Natural Time
The Natural Time converts date-time objects into human-readable relative times such as 2 days ago, 3 hours ago. Following is the example of natural time −
import humanize from datetime import datetime, timedelta past_date = datetime.now() - timedelta(days=2) print(humanize.naturaltime(past_date))
Output
2 days ago
Natural Date
The Natural Date formats specific dates into a readable format like "July 11, 2024". Here is the example −
import humanize from datetime import datetime some_date = datetime(2022, 7, 8) print(humanize.naturaldate(some_date))
Output
Jul 08 2022
Natural Day
The Natural Day provides a human-readable representation of a date by considering today's date for contextual relevance, for example "today", "tomorrow", "yesterday" etc. Below is the example of it −
import humanize from datetime import datetime, timedelta today = datetime.now() tomorrow = today + timedelta(days=1) print(humanize.naturalday(today)) print(humanize.naturalday(tomorrow))
Output
today tomorrow
Precise Delta
The Precise Delta converts time duration into human-readable strings by breaking down into days, hours, minutes and second. Here is the example of it −
import humanize from datetime import timedelta duration = timedelta(days=2, hours=3, minutes=30) print(humanize.precisedelta(duration))
Output
2 days, 3 hours and 30 minutes
Duration Utilities
The humanize package in Python also includes the Duration Utilities for converting duration (time intervals) into human-readable formats. These utilities help to present duration in a way that is understandable and meaningful to users. Here's an overview of the duration utilities provided by humanize package −
Duration Formatting using naturaldelta()
The naturaldelta() function converts time deltas (duration) into human-readable strings that describe the duration in a natural language format such as "2 hours ago", "3 days from now", etc.
Following is the example of using the naturaldelta() function of the Python Humanize Package −
from datetime import timedelta import humanize Using naturaldelta for time durations delta1 = timedelta(days=3, hours=5) print(f"Time duration: {humanize.naturaldelta(delta1)} from now") Example of a future duration (delta2) delta2 = timedelta(hours=5) print(f"Future duration: in {humanize.naturaldelta(delta2)}") Example of a past duration (delta3) delta3 = timedelta(days=1, hours=3) print(f"Past duration: {humanize.naturaldelta(delta3)} ago")
Output
Time duration: 3 days from now Future duration: in 5 hours Past duration: a day ago