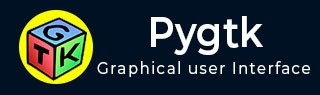
- PyGTK Tutorial
- PyGTK - Home
- PyGTK - Introduction
- PyGTK - Environment
- PyGTK - Hello World
- PyGTK - Important Classes
- PyGTK - Window Class
- PyGTK - Button Class
- PyGTK - Label CLass
- PyGTK - Entry Class
- PyGTK - Signal Handling
- PyGTK - Event Handling
- PyGTK - Containers
- PyGTK - Box Class
- PyGTK - ButtonBox Class
- PyGTK - Alignment Class
- PyGTK - EventBox Class
- PyGTK - Layout Class
- PyGTK - ComboBox Class
- PyGTK - ToggleButton Class
- PyGTK - CheckButton Class
- PyGTK - RadioButton Class
- PyGTK - MenuBar, Menu & MenuItem
- PyGTK - Toolbar Class
- PyGTK - Adjustment Class
- PyGTK - Range Class
- PyGTK - Scale Class
- PyGTK - Scrollbar Class
- PyGTK - Dialog Class
- PyGTK - MessageDialog Class
- PyGTK - AboutDialog Class
- PyGTK - Font Selection Dialog
- PyGTK - Color Selection Dialog
- PyGTK - File Chooser Dialog
- PyGTK - Notebook Class
- PyGTK - Frame Class
- PyGTK - AspectFrame Class
- PyGTK - TreeView Class
- PyGTK - Paned Class
- PyGTK - Statusbar Class
- PyGTK - ProgressBar Class
- PyGTK - Viewport Class
- PyGTK - Scrolledwindow Class
- PyGTK - Arrow Class
- PyGTK - Image Class
- PyGTK - DrawingArea Class
- PyGTK - SpinButton Class
- PyGTK - Calendar Class
- PyGTK - Clipboard Class
- PyGTK - Ruler Class
- PyGTK - Timeout
- PyGTK - Drag and Drop
- PyGTK Useful Resources
- PyGTK - Quick Guide
- PyGTK - Useful Resources
- PyGTK - Discussion
PyGTK - Timeout
The gobject module of the PyGTK API has a useful function to create a timeout function that will be called periodically.
source_id = gobject.timeout_add(interval, function, …)
The second argument is the callback function you wish to have called after every millisecond which is the value of the first argument – interval. Additional arguments may be passed to the callback as function data.
The return value of this function is source_id. Using it, the callback function is stopped from calling.
gobject.source_remove(source_id)
The callback function must return True in order to keep repeating. Therefore, it can be stopped by returning False.
Two buttons and two labels are put on a toplevel window in the following program. One label displays an incrementing number. The btn1 calls on_click which sets the timeout function with an interval of 1000 ms (1 second).
btn1.connect("clicked", self.on_click) def on_click(self, widget): self.source_id = gobject.timeout_add(1000, counter, self)
The timeout function is named as counter(). It increments the number on a label after every 1 second.
def counter(timer): c=timer.count+1 print c timer.count=c timer.lbl.set_label(str(c)) return True
The Callback on the second button removes the timeout function.
btn2.connect("clicked", self.on_stop) def on_stop(self, widget): gobject.source_remove(self.source_id)
Example
The following is the complete code for the Timeout example −
import gtk, gobject def counter(timer): c = timer.count+1 print c timer.count = c timer.lbl.set_label(str(c)) return True class PyApp(gtk.Window): def __init__(self): super(PyApp, self).__init__() self.set_title("Timeout Demo") self.set_size_request(300, 200) self.set_position(gtk.WIN_POS_CENTER) vbox = gtk.VBox(False, 5) hbox = gtk.HBox(True, 3) hb = gtk.HBox() lbl1 = gtk.Label("Counter: ") hb.add(lbl1) self.lbl = gtk.Label("") hb.add(self.lbl) valign = gtk.Alignment(0.5, 0.5, 0, 0) valign.add(hb) vbox.pack_start(valign, True, True, 10) btn1 = gtk.Button("start") btn2 = gtk.Button("stop") self.count = 0 self.source_id = 0 hbox.add(btn1) hbox.add(btn2) halign = gtk.Alignment(0.5, 0.5, 0, 0) halign.add(hbox) vbox.pack_start(halign, False, True, 10) self.add(vbox) btn1.connect("clicked", self.on_click) btn2.connect("clicked", self.on_stop) self.connect("destroy", gtk.main_quit) self.show_all() def on_click(self, widget): self.source_id = gobject.timeout_add(1000, counter, self) def on_stop(self, widget): gobject.source_remove(self.source_id) PyApp() gtk.main()
When executed, the window shows two buttons at the bottom. The number on the label will increment periodically when the Start button is clicked on and it will stop incrementing when the Stop button is clicked on.
Observe the output −
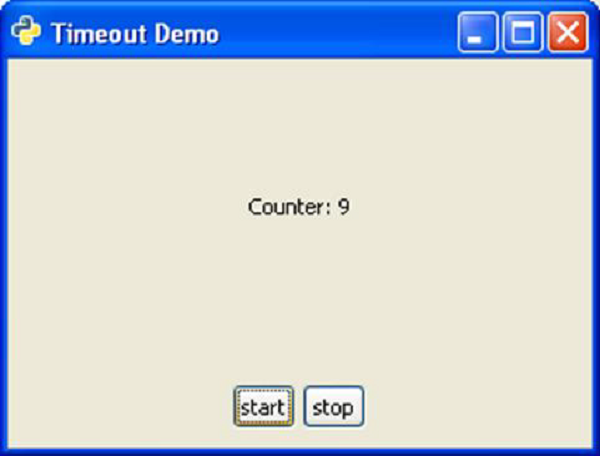