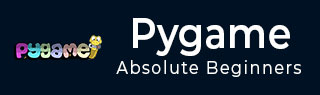
- Pygame Tutorial
- Pygame - Home
- Pygame - Overview
- Pygame - Hello World
- Pygame - Display modes
- Pygame - Locals module
- Pygame - Color object
- Pygame - Event objects
- Pygame - Keyboard events
- Pygame - Mouse events
- Pygame - Drawing shapes
- Pygame - Loading image
- Pygame - Displaying Text in Window
- Pygame - Moving an image
- Pygame - Moving with Numeric pad keys
- Pygame - Moving with mouse
- Pygame - Moving Rectangular objects
- Pygame - Use Text as Buttons
- Pygame - Transforming Images
- Pygame - Sound objects
- Pygame - Mixer channels
- Pygame - Playing music
- Pygame - Playing Movie
- Pygame - Using Camera module
- Pygame - Load cursor
- Pygame - Access CDROM
- Pygame - The Sprite Module
- Pygame - PyOpenGL
- Pygame - Errors and Exception
- Pygame Useful Resources
- Pygame - Quick Guide
- Pygame - Useful Resources
- Pygame - Discussion
Pygame - Using Camera Module
Earlier versions of Pygame upto 1.9.6 contain pygame.camera module. This module contains functionality to capture camera feed on the game window and grab an image from it. The camera devices available to the system are enumerated in a list returned by list_cameras() method.
pygame.camera.list_cameras()
To initialize a camera object, use camera id, resolution and format arguments.
pygame.camera.Camera(device, (width, height), format)
The default format is RGB. Width and height parameters are by default 640x480.
The camera module has following methods defined in Camera class.
pygame.camera.Camera.start() | opens, initializes, and starts capturing |
pygame.camera.Camera.stop() | stops, uninitializes, and closes the camera |
pygame.camera.Camera.get_controls() | gets current values of user controls |
pygame.camera.Camera.set_controls() | changes camera settings if supported by the camera |
pygame.camera.Camera.get_size() | returns the dimensions of the images being recorded |
pygame.camera.Camera.query_image() | checks if a frame is ready |
pygame.camera.Camera.get_image() | captures an image as a Surface |
pygame.camera.Camera.get_raw() | returns an unmodified image as a string |
Example
Following programs captures live feed from computer’s default web camera.
import pygame import pygame.camera pygame.init() gameDisplay = pygame.display.set_mode((640,480)) pygame.camera.init() print (pygame.camera.list_cameras()) cam = pygame.camera.Camera(0) cam.start() while True: img = cam.get_image() gameDisplay.blit(img,(0,0)) pygame.display.update() for event in pygame.event.get() : if event.type == pygame.QUIT : cam.stop() pygame.quit() exit()
Please note that on Windows OS, you may have to install Videocapture module.
pip3 install VideoCapture
Output
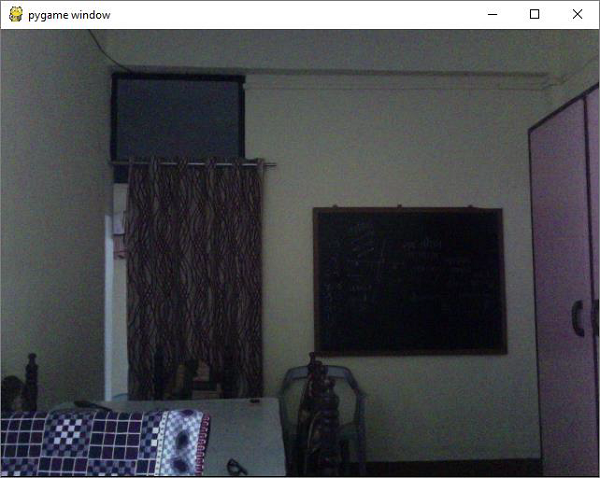
Advertisements