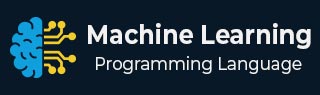
- Machine Learning Basics
- Machine Learning - Home
- Machine Learning - Getting Started
- Machine Learning - Basic Concepts
- Machine Learning - Python Libraries
- Machine Learning - Applications
- Machine Learning - Life Cycle
- Machine Learning - Required Skills
- Machine Learning - Implementation
- Machine Learning - Challenges & Common Issues
- Machine Learning - Limitations
- Machine Learning - Reallife Examples
- Machine Learning - Data Structure
- Machine Learning - Mathematics
- Machine Learning - Artificial Intelligence
- Machine Learning - Neural Networks
- Machine Learning - Deep Learning
- Machine Learning - Getting Datasets
- Machine Learning - Categorical Data
- Machine Learning - Data Loading
- Machine Learning - Data Understanding
- Machine Learning - Data Preparation
- Machine Learning - Models
- Machine Learning - Supervised
- Machine Learning - Unsupervised
- Machine Learning - Semi-supervised
- Machine Learning - Reinforcement
- Machine Learning - Supervised vs. Unsupervised
- Machine Learning Data Visualization
- Machine Learning - Data Visualization
- Machine Learning - Histograms
- Machine Learning - Density Plots
- Machine Learning - Box and Whisker Plots
- Machine Learning - Correlation Matrix Plots
- Machine Learning - Scatter Matrix Plots
- Statistics for Machine Learning
- Machine Learning - Statistics
- Machine Learning - Mean, Median, Mode
- Machine Learning - Standard Deviation
- Machine Learning - Percentiles
- Machine Learning - Data Distribution
- Machine Learning - Skewness and Kurtosis
- Machine Learning - Bias and Variance
- Machine Learning - Hypothesis
- Regression Analysis In ML
- Machine Learning - Regression Analysis
- Machine Learning - Linear Regression
- Machine Learning - Simple Linear Regression
- Machine Learning - Multiple Linear Regression
- Machine Learning - Polynomial Regression
- Classification Algorithms In ML
- Machine Learning - Classification Algorithms
- Machine Learning - Logistic Regression
- Machine Learning - K-Nearest Neighbors (KNN)
- Machine Learning - Naïve Bayes Algorithm
- Machine Learning - Decision Tree Algorithm
- Machine Learning - Support Vector Machine
- Machine Learning - Random Forest
- Machine Learning - Confusion Matrix
- Machine Learning - Stochastic Gradient Descent
- Clustering Algorithms In ML
- Machine Learning - Clustering Algorithms
- Machine Learning - Centroid-Based Clustering
- Machine Learning - K-Means Clustering
- Machine Learning - K-Medoids Clustering
- Machine Learning - Mean-Shift Clustering
- Machine Learning - Hierarchical Clustering
- Machine Learning - Density-Based Clustering
- Machine Learning - DBSCAN Clustering
- Machine Learning - OPTICS Clustering
- Machine Learning - HDBSCAN Clustering
- Machine Learning - BIRCH Clustering
- Machine Learning - Affinity Propagation
- Machine Learning - Distribution-Based Clustering
- Machine Learning - Agglomerative Clustering
- Dimensionality Reduction In ML
- Machine Learning - Dimensionality Reduction
- Machine Learning - Feature Selection
- Machine Learning - Feature Extraction
- Machine Learning - Backward Elimination
- Machine Learning - Forward Feature Construction
- Machine Learning - High Correlation Filter
- Machine Learning - Low Variance Filter
- Machine Learning - Missing Values Ratio
- Machine Learning - Principal Component Analysis
- Machine Learning Miscellaneous
- Machine Learning - Performance Metrics
- Machine Learning - Automatic Workflows
- Machine Learning - Boost Model Performance
- Machine Learning - Gradient Boosting
- Machine Learning - Bootstrap Aggregation (Bagging)
- Machine Learning - Cross Validation
- Machine Learning - AUC-ROC Curve
- Machine Learning - Grid Search
- Machine Learning - Data Scaling
- Machine Learning - Train and Test
- Machine Learning - Association Rules
- Machine Learning - Apriori Algorithm
- Machine Learning - Gaussian Discriminant Analysis
- Machine Learning - Cost Function
- Machine Learning - Bayes Theorem
- Machine Learning - Precision and Recall
- Machine Learning - Adversarial
- Machine Learning - Stacking
- Machine Learning - Epoch
- Machine Learning - Perceptron
- Machine Learning - Regularization
- Machine Learning - Overfitting
- Machine Learning - P-value
- Machine Learning - Entropy
- Machine Learning - MLOps
- Machine Learning - Data Leakage
- Machine Learning - Resources
- Machine Learning - Quick Guide
- Machine Learning - Useful Resources
- Machine Learning - Discussion
Machine Learning - Gaussian Discriminant Analysis
Gaussian Discriminant Analysis (GDA) is a statistical algorithm used in machine learning for classification tasks. It is a generative model that models the distribution of each class using a Gaussian distribution, and it is also known as the Gaussian Naive Bayes classifier.
The basic idea behind GDA is to model the distribution of each class as a multivariate Gaussian distribution. Given a set of training data, the algorithm estimates the mean and covariance matrix of each class's distribution. Once the parameters of the model are estimated, it can be used to predict the probability of a new data point belonging to each class, and the class with the highest probability is chosen as the prediction.
The GDA algorithm makes several assumptions about the data −
The features are continuous and normally distributed.
The covariance matrix of each class is the same.
The features are independent of each other given the class.
Assumption 1 means that GDA is not suitable for data with categorical or discrete features. Assumption 2 means that GDA assumes that the variance of each feature is the same across all classes. If this is not true, the algorithm may not perform well. Assumption 3 means that GDA assumes that the features are independent of each other given the class label. This assumption can be relaxed using a different algorithm called Linear Discriminant Analysis (LDA).
Example
The implementation of GDA in Python is relatively straightforward. Here's an example of how to implement GDA on the Iris dataset using the scikit-learn library −
from sklearn.datasets import load_iris from sklearn.discriminant_analysis import QuadraticDiscriminantAnalysis from sklearn.model_selection import train_test_split # Load the iris dataset iris = load_iris() # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.3, random_state=42) # Train a GDA model gda = QuadraticDiscriminantAnalysis() gda.fit(X_train, y_train) # Make predictions on the testing set y_pred = gda.predict(X_test) # Evaluate the model's accuracy accuracy = (y_pred == y_test).mean() print('Accuracy:', accuracy)
In this example, we first load the Iris dataset using the load_iris function from scikit-learn. We then split the data into training and testing sets using the train_test_split function. We create a QuadraticDiscriminantAnalysis object, which represents the GDA model, and train it on the training data using the fit method. We then make predictions on the testing set using the predict method and evaluate the model's accuracy by comparing the predicted labels to the true labels.
Output
The output of this code will show the model's accuracy on the testing set. For the Iris dataset, the GDA model typically achieves an accuracy of around 97-99%.
Accuracy: 0.9811320754716981
Overall, GDA is a powerful algorithm for classification tasks that can handle a wide range of data types, including continuous and normally distributed data. While it makes several assumptions about the data, it is still a useful and effective algorithm for many real-world applications.