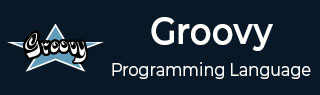
- Groovy - Home
- Groovy - Overview
- Groovy - Environment
- Groovy - Basic Syntax
- Groovy - Data Types
- Groovy - Variables
Groovy Operators
- Groovy - Operators
- Groovy - Arithmetic Operators
- Groovy - Assignment Operators
- Groovy - Relational Operators
- Groovy - Logical Operators
- Groovy - Bitwise Operators
- Groovy Operator Precedence & Associativity
Control Statements
- Groovy - Decision Making
- Groovy - If Else Statement
- Groovy - Switch Statement
- Groovy - Loops
- Groovy - For Loop
- Groovy - For-in Loop
- Groovy - While Loop
- Groovy - Do While Loop
- Groovy - Break Statement
- Groovy - Continue Statement
Groovy File Handling
- Groovy - File I/O
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
Groovy Error & Exceptions
- Groovy - Exception Handling
- Groovy - try-catch Block
- Groovy - try-with-resources
- Groovy - Multi-catch Block
- Groovy - Nested try Block
- Groovy - Finally Block
- Groovy - throw Exception
- Groovy - Exception Propagation
- Groovy - Built-in Exceptions
- Groovy - Custom Exception
Groovy Multithreading
- groovy - Multithreading
- groovy - Thread Life Cycle
- groovy - Creating a Thread
- groovy - Starting a Thread
- groovy - Joining Threads
- groovy - Naming Thread
Groovy Synchronization
- Groovy - Operators
- Groovy - Loops
- Groovy - Methods
- Groovy - Optionals
- Groovy - Numbers
- Groovy - Strings
- Groovy - Ranges
- Groovy - Lists
- Groovy - Maps
- Groovy - Dates & Times
- Groovy - Regular Expressions
- Groovy - Exception Handling
- Groovy - Object Oriented
- Groovy - Generics
- Groovy - Traits
- Groovy - Closures
- Groovy - Annotations
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSLS
- Groovy - Database
- Groovy - Builders
- Groovy - Command Line
- Groovy - Unit Testing
- Groovy - Template Engines
- Groovy - Meta Object Programming
- Groovy Useful Resources
- Groovy - Quick Guide
- Groovy - Useful Resources
- Groovy - Discussion
Groovy - Logical Operators
Logical operators are used to evaluate Boolean expressions. Following are the logical operators available in Groovy −
Operator | Description | Example |
---|---|---|
&& | This is the logical and operator | true && true will give true |
|| | This is the logical or operator | true || true will give true |
! | This is the logical not operator | !false will give true |
Example - Usage of AND operator
In this example, we're creating two variables x and y and using logical operators. We've performed a logical AND operation and printed the result.
Example.groovy
class Example { static void main(String[] args) { boolean x = true; boolean y = false; println("x && y = " + (x && y)); } }
Output
When we run the above program, we will get the following result.
x && y = false
Example - Usage of OR operator
In this example, we're creating two variables x and y and using logical operators. We've performed a logical OR operation and printed the result.
Example.groovy
class Example { static void main(String[] args) { boolean x = true; boolean y = false; println("x || y = " + (x || y)); } }
Output
When we run the above program, we will get the following result.
x || y = true
Example - Usage of Logical NOT operator
In this example, we're creating two variables a and b and using logical operators. We've performed a logical NOT operation and printed the result.
Example.groovy
class Example { static void main(String[] args) { boolean x = true; boolean y = false; println("!(x && y) = " + !(x && y)); } }
Output
When we run the above program, we will get the following result.
!(x && y) = true
Advertisements