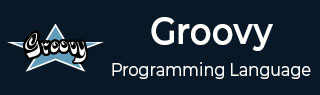
- Groovy Tutorial
- Groovy - Home
- Groovy - Overview
- Groovy - Environment
- Groovy - Basic Syntax
- Groovy - Data Types
- Groovy - Variables
- Groovy - Operators
- Groovy - Loops
- Groovy - Decision Making
- Groovy - Methods
- Groovy - File I/O
- Groovy - Optionals
- Groovy - Numbers
- Groovy - Strings
- Groovy - Ranges
- Groovy - Lists
- Groovy - Maps
- Groovy - Dates & Times
- Groovy - Regular Expressions
- Groovy - Exception Handling
- Groovy - Object Oriented
- Groovy - Generics
- Groovy - Traits
- Groovy - Closures
- Groovy - Annotations
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSLS
- Groovy - Database
- Groovy - Builders
- Groovy - Command Line
- Groovy - Unit Testing
- Groovy - Template Engines
- Groovy - Meta Object Programming
- Groovy Useful Resources
- Groovy - Quick Guide
- Groovy - Useful Resources
- Groovy - Discussion
Groovy - Relational Operators
Relational operators allow of the comparison of objects. Following are the relational operators available in Groovy −
Operator | Description | Example |
---|---|---|
== | Tests the equality between two objects | 2 == 2 will give true |
!= | Tests the difference between two objects | 3 != 2 will give true |
< | Checks to see if the left objects is less than the right operand. | 2 < 3 will give true |
<= | Checks to see if the left objects is less than or equal to the right operand. | 2 <= 3 will give true |
> | Checks to see if the left objects is greater than the right operand. | 3 > 2 will give true |
>= | Checks to see if the left objects is greater than or equal to the right operand. | 3 >= 2 will give true |
The following code snippet shows how the various operators can be used.
class Example { static void main(String[] args) { def x = 5; def y = 10; def z = 8; if(x == y) { println("x is equal to y"); } else println("x is not equal to y"); if(z != y) { println("z is not equal to y"); } else println("z is equal to y"); if(z != y) { println("z is not equal to y"); } else println("z is equal to y"); if(z<y) { println("z is less than y"); } else println("z is greater than y"); if(x<=y) { println("x is less than y"); } else println("x is greater than y"); if(x>y) { println("x is greater than y"); } else println("x is less than y"); if(x>=y) { println("x is greater or equal to y"); } else println("x is less than y"); } }
When we run the above program, we will get the following result. It can be seen that the results are as expected from the description of the operators as shown above.
x is not equal to y z is not equal to y z is not equal to y z is less than y x is less than y x is less than y x is less than y
groovy_operators.htm
Advertisements