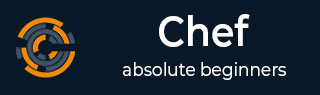
- Chef Tutorial
- Chef - Home
- Chef - Overview
- Chef - Architecture
- Chef - Version Control System Setup
- Chef - Workstation Setup
- Chef - Client Setup
- Chef - Test Kitchen Setup
- Chef - Knife Setup
- Chef - Solo Setup
- Chef - Cookbooks
- Chef - Cookbook Dependencies
- Chef - Roles
- Chef - Environment
- Chef - Chef-Client as Daemon
- Chef - Chef-Shell
- Chef - Testing Cookbooks
- Chef - Foodcritic
- Chef - ChefSpec
- Testing Cookbook with Test Kitchen
- Chef - Nodes
- Chef - Chef-Client Run
- Advanced Chef
- Dynamically Configuring Recipes
- Chef - Templates
- Chef - Plain Ruby with Chef DSL
- Chef - Ruby Gems with Recipes
- Chef - Libraries
- Chef - Definition
- Chef - Environment Variable
- Chef - Data Bags
- Chef - Scripts for Data Bags
- Chef - Cross-Platform Cookbooks
- Chef - Resources
- Lightweight Resource Provider
- Chef - Blueprints
- Chef - Files & Packages
- Chef - Community Cookbooks
- Chef Useful Resources
- Chef - Quick Guide
- Chef - Useful Resources
- Chef - Discussion
Chef - Resources
Chef resource represents a piece of the operating system at its desired state. It is a statement of configuration policy that describes the desired state of a node to which one wants to take the current configuration to using resource providers. It helps in knowing the current status of the target machine using the Ohai mechanism of Chef. It also helps in defining the steps required to perform to get the target machine to that state. The resources are grouped in recipes which describes the working configuration.
In case of Chef, chef::Platform maps the providers and platform versions of each node. At the beginning of every Chef-client run, Chef server collects the details of any machines current state. Later, Chef server uses those values to identify the correct provider.
Resource Syntax
type 'name' do attribute 'value' action :type_of_action end
In the above syntax, ‘type’ is the resource type and ‘name’ is the name that we are going to use. In the ‘do’ and ‘end’ block, we have the attribute of that resource and the action that we need to take for that particular resource.
Every resource that we use in the recipe has its own set of actions, which is defined inside the ‘do’ and ‘end’ block.
Example
type 'name' do attribute 'value' action :type_of_action end
All resources share a common set of functionality, actions, properties, conditional execution, notification, and relevant path of action.
Actions | The :nothing action can be used with any resource or custom resource. |
Properties | The ignore_failure, provider, retries, retry_delay, and supports properties can be used with any resource or custom resources. |
Guards | The not_if and only_if conditional executions can be used to put additional guards around certain resources, so that they are only run when the condition is met. |
Guard Interpreters | Evaluates a string command using a script-based resource: bash, csh, perl, powershell_script, python, or ruby. |
Notifications | The notifies and subscribes notifications can be used with any resource. |
Relative Paths | The #{ENV['HOME']} relative path can be used with any resource. |
Windows File Security | The template, file, remote_file, cookbook_file, directory, and remote_directory resources support the use of inheritance and access control lists (ACLs) within recipes. |
Run in Compile Phase | Sometimes a resource needs to be run before every other resource or after all resources have been added to the resource collection. |
Available Resources
apt_package
Use the apt_package resource to manage packages for the Debian and Ubuntu platforms.
Bash
Use the bash resource to execute scripts using the Bash interpreter. This resource may also use any of the actions and properties that are available to the execute resource. Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
Batch
Use the batch resource to execute a batch script using the cmd.exe interpreter. The batch resource creates and executes a temporary file (similar to how the script resource behaves), rather than running the command inline.
This resource inherits actions (:run and :nothing) and properties (creates, cwd, environment, group, path, timeout, and user) from the execute resource. Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
bff_package
Use the bff_package resource to manage packages for the AIX platform using the installp utility. When a package is installed from a local file, it must be added to the node using the remote_file or cookbook_file resources.
chef_gem
Use the chef_gem resource to install a gem only for the instance of Ruby that is dedicated to the Chef-Client. When a gem is installed from a local file, it must be added to the node using the remote_file or cookbook_file resources.
The chef_gem resource works with all of the same properties and options as the gem_package resource, but does not accept the gem_binary property because it always uses the CurrentGemEnvironment under which the Chef-Client is running. In addition to performing actions similar to the gem_package resource, the chef_gem resource does the above.
cookbook_file
Use the cookbook_file resource to transfer files from a sub-directory of COOKBOOK_NAME/files/ to a specified path located on a host that is running the ChefClient.
The file is selected according to file specificity, which allows different source files to be used based on the hostname, host platform (operating system, distro, or as appropriate), or platform version. Files that are located in the COOKBOOK_NAME/files/default subdirectory may be used on any platform.
Cron
Use the cron resource to manage cron entries for time-based job scheduling. Properties for a schedule will default to * if not provided. The cron resource requires access to a crontab program, typically cron.
Csh
Use the csh resource to execute scripts using the csh interpreter. This resource may also use any of the actions and properties that are available to the execute resource.
Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
Deploy
Use the deploy resource to manage and control deployments. This is a popular resource, but is also complex, having the most properties, multiple providers, the added complexity of callbacks, plus four attributes that support layout modifications from within a recipe.
Directory
Use the directory resource to manage a directory, which is a hierarchy of folders that comprises all of the information stored on a computer. The root directory is the top-level, under which the rest of the directory is organized.
The directory resource uses the name property to specify the path to a location in a directory. Typically, permission to access that location in the directory is required.
dpkg_package
Use the dpkg_package resource to manage packages for the dpkg platform. When a package is installed from a local file, it must be added to the node using the remote_file or cookbook_file resources.
easy_install_package
Use the easy_install_package resource to manage packages for the Python platform.
Env
Use the env resource to manage environment keys in Microsoft Windows. After an environment key is set, Microsoft Windows must be restarted before the environment key is available to the Task Scheduler.
erl_call
Use the erl_call resource to connect to a node located within a distributed Erlang system. Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
Execute
Use the execute resource to execute a single command. Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
File
Use the file resource to manage the files directly on a node.
freebsd_package
Use the freebsd_package resource to manage packages for the FreeBSD platform.
gem_package
Use the gem_package resource to manage gem packages that are only included in recipes. When a package is installed from a local file, it must be added to the node using the remote_file or cookbook_file resources.
Git
Use the git resource to manage source control resources that exist in a git repository. git version 1.6.5 (or higher) is required to use all of the functionality in the git resource.
Group
Use the group resource to manage a local group.
homebrew_package
Use the homebrew_package resource to manage packages for the Mac OS X platform.
http_request
Use the http_request resource to send an HTTP request (GET, PUT, POST, DELETE, HEAD, or OPTIONS) with an arbitrary message. This resource is often useful when custom callbacks are necessary.
Ifconfig
Use the ifconfig resource to manage interfaces.
ips_package
Use the ips_package resource to manage packages (using Image Packaging System (IPS)) on the Solaris 11 platform.
Ksh
Use the ksh resource to execute scripts using the Korn shell (ksh) interpreter. This resource may also use any of the actions and properties that are available to the execute resource.
Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
Link
Use the link resource to create symbolic or hard links.
Log
Use the log resource to create log entries. The log resource behaves like any other resource: built into the resource collection during the compile phase, and then run during the execution phase. (To create a log entry that is not built into the resource collection, use Chef::Log instead of the log resource)
macports_package
Use the macports_package resource to manage packages for the Mac OS X platform.
Mdadm
Use the mdadm resource to manage RAID devices in a Linux environment using the mdadm utility. The mdadm provider will create and assemble an array, but it will not create the config file that is used to persist the array upon reboot.
If the config file is required, it must be done by specifying a template with the correct array layout, and then by using the mount provider to create a file systems table (fstab) entry.
Mount
Use the mount resource to manage a mounted file system.
Ohai
Use the ohai resource to reload the Ohai configuration on a node. This allows recipes that change system attributes (like a recipe that adds a user) to refer to those attributes later on during the chef-client run.
Package
Use the package resource to manage packages. When the package is installed from a local file (such as with RubyGems, dpkg, or RPM Package Manager), the file must be added to the node using the remote_file or cookbook_file resources.
pacman_package
Use the pacman_package resource to manage packages (using pacman) on the Arch Linux platform.
powershell_script
Use the powershell_script resource to execute a script using the Windows PowerShell interpreter, much like how the script and script-based resources—bash, csh, perl, python, and ruby—are used. The powershell_script is specific to the Microsoft Windows platform and the Windows PowerShell interpreter.
Python
Use the python resource to execute scripts using the Python interpreter. This resource may also use any of the actions and properties that are available to the execute resource.
Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
Reboot
Use the reboot resource to reboot a node, a necessary step with some installations on certain platforms. This resource is supported for use on the Microsoft Windows, Mac OS X, and Linux platforms.
registry_key
Use the registry_key resource to create and delete registry keys in Microsoft Windows.
remote_directory
Use the remote_directory resource to incrementally transfer a directory from a cookbook to a node. The directory that is copied from the cookbook should be located under COOKBOOK_NAME/files/default/REMOTE_DIRECTORY.
The remote_directory resource will obey file specificity.
remote_file
Use the remote_file resource to transfer a file from a remote location using file specificity. This resource is similar to the file resource.
Route
Use the route resource to manage the system routing table in a Linux environment.
rpm_package
Use the rpm_package resource to manage packages for the RPM Package Manager platform.
Ruby
Use the ruby resource to execute scripts using the Ruby interpreter. This resource may also use any of the actions and properties that are available to the execute resource.
Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
ruby_block
Use the ruby_block resource to execute Ruby code during a Chef-Client run. Ruby code in the ruby_block resource is evaluated with other resources during convergence, whereas Ruby code outside of a ruby_block resource is evaluated before other resources, as the recipe is compiled.
Script
Use the script resource to execute scripts using a specified interpreter, such as Bash, csh, Perl, Python, or Ruby. This resource may also use any of the actions and properties that are available to the execute resource.
Commands that are executed with this resource are (by their nature) not idempotent, as they are typically unique to the environment in which they are run. Use not_if and only_if to guard this resource for idempotence.
Service
Use the service resource to manage a service.
smart_os_package
Use the smartos_package resource to manage packages for the SmartOS platform.
solaris_package
The solaris_package resource is used to manage packages for the Solaris platform.
Subversion
Use the subversion resource to manage source control resources that exist in a Subversion repository.
Template
Use the template resource to manage the contents of a file using an Embedded Ruby (ERB) template by transferring files from a sub-directory of COOKBOOK_NAME/templates/ to a specified path located on a host that is running the Chef-Client. This resource includes actions and properties from the file resource. Template files managed by the template resource follow the same file specificity rules as the remote_file and file resources.
User
Use the user resource to add users, update existing users, remove users, and to lock/unlock user passwords.
windows_package
Use the windows_package resource to manage Microsoft Installer Package (MSI) packages for the Microsoft Windows platform.
windows_service
Use the windows_service resource to manage a service on the Microsoft Windows platform.
yum_package
Use the yum_package resource to install, upgrade, and remove packages with Yum for the Red Hat and CentOS platforms. The yum_package resource is able to resolve provides data for packages much like Yum can do when it is run from the command line. This allows a variety of options for installing packages, like minimum versions, virtual provides, and library names.