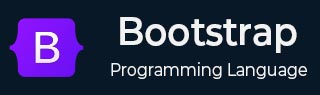
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Checkbox and Radios
This chapter will discuss about checkbox and radios utilities provided by Bootstrap. Checkboxes allow you to select one or more alternatives from a list, whereas radio buttons allow you to choose only one.
Approach
Bootstrap provides wrapper class .form-check for improved layout and behavior of browser default checkboxes and radios elements. It also allows greater customization and cross browser consistency.
.form-check-label class is used to show checkbox labels.
.form-check-input class is used for input type checkbox.
Structurally, the input and label act as siblings.
Bootstrap's custom icons are used to display checked or indeterminate states.
Checkbox
Checkboxes select one or several options from a list.
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Checkbox</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="defaultCheck"> <label class="form-check-label" for="defaultCheck"> item 1 </label> </div> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="flexCheckChecked" checked> <label class="form-check-label" for="flexCheckChecked"> item 2 </label> </div> </body> </html>
Indeterminate
The :indeterminate pseudo class is used to create intermediate state checkboxes.
The indeterminate state is set via JavaScript as there is no equivalent HTML attribute available to specify it.
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Checkbox</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="indeterminateCheckbox" > <label class="form-check-label" for="indeterminateCheckbox"> item 1 </label> </div> <script> var x = document.getElementById("indeterminateCheckbox").indeterminate = true;; </script> </body> </html>
Disabled checkbox
To indicate disabled state use disabled attribute. This makes the associated <label> lighter color indicating disabled state.
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Checkbox</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="disabledIndeterminateCheckbox" disabled> <label class="form-check-label" for="disabledIndeterminateCheckbox"> item 1 </label> </div> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="disabledCheckedCheckbox" checked disabled> <label class="form-check-label" for="disabledCheckedCheckbox"> item 2 </label> </div> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="disabledCheckbox" disabled> <label class="form-check-label" for="disabledCheckbox"> item 3 </label> </div> </body> <script> var x = document.getElementById("disabledIndeterminateCheckbox").indeterminate = true; </script> </html>
Radios
Radio button limits the number of choices to only one in a list.
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Radio</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check"> <input class="form-check-input" type="radio" name="flexRadioDefault" id="defaultRadio"> <label class="form-check-label" for="defaultRadio"> Item 1 </label> </div> <div class="form-check"> <input class="form-check-input" type="radio" name="flexRadioDefault" id="defaultCheckRadio" checked> <label class="form-check-label" for="defaultCheckRadio"> Item 2 </label> </div> </body> </html>
Disabled Radio Button
To indicate disabled state use disabled attribute and the associated <label>'s are styled with a lighter color.
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Form - Radio</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check"> <input class="form-check-input" type="radio" name="flexRadioDisabled" id="disabledRadio" disabled> <label class="form-check-label" for="disabledRadio"> Item 1 </label> </div> <div class="form-check"> <input class="form-check-input" type="radio" name="flexRadioDisabled" id="disabledCheckedRadio" checked disabled> <label class="form-check-label" for="disabledCheckedRadio"> Item 2 </label> </div> </body> </html>
Switches
The switch has the custom checkbox markup, to render the toggle use .form-switch class. Use role="switch" to convey the type of control to assistive technologies.
The switches supports the disabled attribute. The older assistive technologies just announced it as a normal checkbox as a fallback.
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Form - Radio</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check form-switch"> <input class="form-check-input" type="checkbox" role="switch" id="defaultSwitchCheckbox"> <label class="form-check-label" for="defaultSwitchCheckbox">Wi-fi</label> </div> <div class="form-check form-switch"> <input class="form-check-input" type="checkbox" role="switch" id="defaultSwitchCheckedCheckbox" checked> <label class="form-check-label" for="defaultSwitchCheckedCheckbox">Bluetooth</label> </div> <div class="form-check form-switch"> <input class="form-check-input" type="checkbox" role="switch" id="disabledSwitchCheckbox" disabled> <label class="form-check-label" for="disabledSwitchCheckbox">Whatsapp Notification</label> </div> <div class="form-check form-switch"> <input class="form-check-input" type="checkbox" role="switch" id="disabledSwitchCheckedCheckbox" checked disabled> <label class="form-check-label" for="disabledSwitchCheckedCheckbox">Facebook Notification</label> </div> </body> </html>
Default checkbox and radios (stacked)
The radios and checkboxes can be stacked vertically using the .form-check class.
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Checkbox and Radios</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check"> <input class="form-check-input" type="radio" name="Radios" id="defaultStackedRadio" value="option2"> <label class="form-check-label" for="defaultStackedRadio"> English </label> </div> <div class="form-check"> <input class="form-check-input" type="radio" name="Radios" id="disabledStackedRadio" value="option3" disabled> <label class="form-check-label" for="disabledStackedRadio"> Hindi </label> </div> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="defaultCheckbox"> <label class="form-check-label" for="defaultCheckbox"> Marathi </div> <div class="form-check"> <input class="form-check-input" type="checkbox" value="" id="disabledCheckbox" disabled> <label class="form-check-label" for="disabledCheckbox"> Japanes </label> </div> </body> </html>
Inline
To put checkboxes and radios next to each other, use the class .form-check-inline with any .form-check.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Checkbox and Radios</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check form-check-inline"> <input class="form-check-input" type="radio" name="inlineRadioOptions" id="inlineDeafultRadio" value="option1"> <label class="form-check-label" for="inlineDeafultRadio">English</label> </div> <div class="form-check form-check-inline"> <input class="form-check-input" type="radio" name="inlineRadioOptions" id="inlineDisabledRadio" value="option3" disabled> <label class="form-check-label" for="inlineDisabledRadio">Hindi</label> </div> <div class="form-check form-check-inline"> <input class="form-check-input" type="checkbox" id="inlineDeafultCheckbox" value="option1"> <label class="form-check-label" for="inlineDeafultCheckbox">Marathi</label> </div> <div class="form-check form-check-inline"> <input class="form-check-input" type="checkbox" id="inlineDisabledCheckbox" value="option3" disabled> <label class="form-check-label" for="inlineDisabledCheckbox">Japanes</label> </div> </body> </html>
Reverse
Place checkboxes, radios and switches on opposite sides using .form-check-reverse modifier class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Checkbox and Radios</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="form-check form-check-reverse"> <h4>Inline Checkboxes</h4> <input class="form-check-input" type="checkbox" value="" id="deafultReverseCheckbox"> <label class="form-check-label" for="deafultReverseCheckbox"> English </label> </div> <div class="form-check form-check-reverse"> <input class="form-check-input" type="checkbox" value="" id="disabledReverseCheckbox" disabled> <label class="form-check-label" for="disabledReverseCheckbox"> Hindi </label> </div> <div class="form-check form-switch form-check-reverse"> <input class="form-check-input" type="checkbox" id="switchReverseCheckbox"> <label class="form-check-label" for="switchReverseCheckbox">Wifi</label> </div> <div class="form-check form-check-reverse"> <h4>Inline Radios</h4> <input class="form-check-input" type="radio" value="" id="deafultReverseRadio"> <label class="form-check-label" for="deafultReverseRadio"> Marathi </label> </div> <div class="form-check form-check-reverse"> <input class="form-check-input" type="radio" value="" id="disabledReverseRadio" disabled> <label class="form-check-label" for="disabledReverseRadio"> Japanes </label> </div> <div class="form-check form-switch form-check-reverse"> <input class="form-check-input" type="radio" id="switchReverseRadio"> <label class="form-check-label" for="switchReverseRadio">Bluetooth</label> </div> </body> </html>
Without Labels
Skip the wrapping class .form-check for checkboxes and radio that have no label text.
Provide an accessible name for assistive technologies (for instance, using aria-label). For information, refer accessibility section of the Forms overview section.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Form - Checkbox and Radio Buttons</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div> <input class="form-check-input" type="checkbox" id="checkboxNoLabel" value="" aria-label="..."> Item 1 </div> <div> <input class="form-check-input" type="radio" name="radioNoLabel" id="radioNoLabel1" value="" aria-label="..."> Item 2 </div> </body> </html>
Toggle Buttons
Use .btn class on the <label> element rather than .form-check-label to create button-like checkboxes and radio buttons. These toggle buttons may also be placed together in a button group.
Use .btn-check class which indicate that the input is of a button-type checkbox.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Checkbox and Radios</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h5>Checkbox Toggle Buttons</h5> <input type="checkbox" class="btn-check" id="defaultToggleChecbox" autocomplete="off"> <label class="btn btn-primary" for="defaultToggleChecbox">Item 1</label> <input type="checkbox" class="btn-check" id="checkedToggleChecbox" checked autocomplete="off"> <label class="btn btn-secondary" for="checkedToggleChecbox">Item 2</label> <input type="checkbox" class="btn-check" id="disabledToggleChecbox" autocomplete="off" disabled> <label class="btn btn-info" for="disabledToggleChecbox">Item 3</label> <h5>Radios Toggle Buttons</h5> <input type="radio" class="btn-check" id="defaultToggleRadio" autocomplete="off"> <label class="btn btn-primary" for="defaultToggleRadio">Item 1</label> <input type="radio" class="btn-check" id="checkedToggleRadio" checked autocomplete="off"> <label class="btn btn-secondary" for="checkedToggleRadio">Item 2</label> <input type="radio" class="btn-check" id="disabledToggleRadio" autocomplete="off" disabled> <label class="btn btn-info" for="disabledToggleRadio">Item 3</label> </body> </html>
Outlined Styles
Different variants of .btn are supported, including different outline styles as demonstrated in below example.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Checkbox and Radio Buttons</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <input type="checkbox" class="btn-check" id="defualtOutlineCheckbox" autocomplete="off"> <label class="btn btn-outline-primary" for="defualtOutlineCheckbox">Single toggle</label><br><br> <input type="checkbox " class="btn-check" id="checkedOutlineCheckbox" checked autocomplete="off"> <label class="btn btn-secondary" for="checkedOutlineCheckbox">Checked</label><br><br> <input type="radio" class="btn-check" name="options-outlined" id="checkedOutlineRadio" autocomplete="off" checked> <label class="btn btn-info" for="checkedOutlineRadio">Checked success radio</label> <input type="radio" class="btn-check" name="options-outlined" id="defualtOutlineRadio" autocomplete="off"> <label class="btn btn-outline-warning" for="defualtOutlineRadio">Danger radio</label> </body> </html>