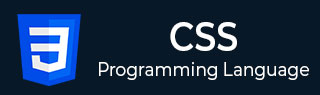
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Flexbox
Flexbox organizes elements within a container along a single dimension, which can be either horizontally or vertically aligned.
What is CSS Flexbox?
CSS flexbox is a layout model in CSS that provides an efficient and flexible way to arrange and distribute space between items within a container. It arranges elements in a single dimension, either horizontally or vertically, within a container.
In this article we will cover all the properties for managing the positioning, alignment, and gaps between elements along both the main and cross axes briefly.
Table of Contents
Elements of Flexbox
- Flexbox Container: The flex container defines the outer element of flexbox, where all the child elements are present. A flexbox container can be defined by setting 'display: flex' or 'display: inline-flex'.
- Flexbox items: Flexbox items are direct children of flexbox container. This items can be arranged vertically and horizontally inside flexbox container based on need.
- Main Axis: Main axis is primary axis along which items arranged in a container. By default this is horizontal axis.
- Cross Axis: >Cross axis is perpendicular axis to primary axis. By default this is vertical axis.
Following Diagram will demonstrate the CSS Flexbox:
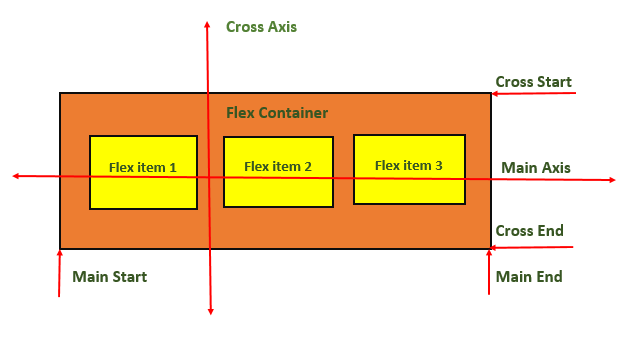
Basic Flexbox Layout
Flexbox is commonly used to create responsive flexbox layout.
Example
<!DOCTYPE html> <html lang="en"> <head> <style> .container { display: flex; flex-direction: row; justify-content: space-between; align-items: center; height: 100vh; } .item { background-color: lightcoral; padding: 20px; margin: 10px; border: 3px solid #ccc; } </style> </head> <body> <div class="container"> <div class="item">Item 1</div> <div class="item">Item 2</div> <div class="item">Item 3</div> </div> </body> </html>
Vertical Flexbox Layout
In CSS, we can also define vertical flexbox by setting `flex-direction: column`.
Example
<!DOCTYPE html> <html lang="en"> <head> <style> .container { display: flex; flex-direction: column; justify-content: center; align-items: center; height: 100vh; } .item { background-color: lightseagreen; padding: 20px; margin: 10px; border: 3px solid #ccc; } </style> </head> <body> <div class="container"> <div class="item">Item 1</div> <div class="item">Item 2</div> <div class="item">Item 3</div> </div> </body> </html>
Flexbox Justify Content Property
The `justify-content` property is commonly used inside flexbox containers to align flexbox items inside container. There are several values possible for this property, for a complete reference of justify-content property visit our tutorial on justify-content property.
Some of the commonly used values of justify-content is noted down below. There are lot more values associated with it.
// Align Item at center of main axis justify-content: center; // Align Item at start of main axis justify-content: flex-start; // Align Item at end of main axis justify-content: flex-end; // Align Item at left side of main axis justify-content: left; // Align Item at right side of main axis justify-content: right;
Flexbox Align Items Property
The `align-items` property in CSS is used to align flex items across cross axis (vertical axis in a row layout, horizontal axis in a column layout) of the container. There are several values associated with this property. To learn more about `align-items` property visit our tutorial on CSS Align Items.
// Align items at start of cross axis of container align-items: flex-start; // Align items at end of cross axis of container align-items: flex-end; // Align items at center of cross axis of container align-items: center; // Align baselines of items (For items with variable sizes) align-items: baseline; // Stretch items along cross axis to fill container align-items: stretch;
Centering a Div using Flexbox
Centering a div element( or any other elements ) is always a challenging and most discussed problem in CSS. With the help of CSS flexbox we can easily center a div element inside a container. We have to use `justify-content` and `align-items` properties to center items, following code shows how this is done.
Example
<!DOCTYPE html> <html lang="en"> <head> <style> .flex-container { display: flex; /* Center horizontally */ justify-content: center; /* Center Vertically */ align-items: center; border: 1px solid #ccc; height: 250px; background-color: grey; } .flex-item { background-color: lightblue; border: 1px solid #333; padding: 5px; height: 70px; width: 70px; } </style> </head> <body> <div class="flex-container"> <div class="flex-item"> This div is in center of container </div> </div> </body> </html>
Flexbox Wrap Property
Flexbox with wrap property allows items within a container to move to next line when there is no enough space in a single line. This helps to maintain a responsive layout.
Following code demonstrates how to use flexbox to create a responsive layout that centers its items and wraps them to fit within the available space. For a complete guidance on flex wrap, visit our tutorial on CSS flex-wrap.
.container { display: flex; flex-wrap: wrap; justify-content: center; align-items: center; } .item { padding: 20px; margin: 10px; /* base size 100px */ flex: 1 1 100px; }
Flexbox Align Self Property
In CSS, we have `align-self` property which can be used to override `align-items` property set on container. This helps to dynamically place each items at special locations inside container.
Following code shows how to use `align-self` property. Here we can see that `align-items: stretch` is not applicable to second and third items, this property is override by `align-self` property of items.
.container { display: flex; flex-direction: row; justify-content: space-around; align-items: stretch; height: 250px; } .item { background-color: lightpink; padding: 20px; margin: 10px; border: 1px solid #ccc; } .item:nth-child(2) { /* Center 2nd item along the cross axis */ align-self: center; } .item:nth-child(3) { /* Align 3rd item at the end of the cross axis */ align-self: flex-end; }
CSS Flexbox Container Properties
Following are all the CSS properties that can be applied to flex container.
Property | Value | Example |
---|---|---|
flex-direction | Sets the flex direction of the flex items. | |
flex-wrap | Sets whether flex items should wrap onto the next line | |
justify-content | Sets the alignment of flex items along the main axis. | |
align-items | Sets the alignment of flex items along the cross axis. | |
align-content | Sets the alignment and spacing of flex lines within the flex container. | |
flex-flow | Sets both the flex-direction and flex-wrap properties. |
CSS Flexbox Items Properties
Following are all the CSS properties that can be applied to flex items.
Property | Value | Example |
---|---|---|
flex-grow | Sets flex item to grow within a flex container. | |
flex-shrink | Sets flex item to shrink in size to fit the available space. | |
flex-basis | Sets the initial size of a flex item. | |
flex | Shorthand property, combines three flex-related properties: flex-grow, flex-shrink, and flex-basis. | |
align-self | Sets the alignment of a specific flex item along the cross-axis. | |
order | Used to specify the order in which flex items appear inside their flex container. |