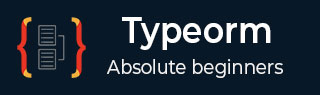
- TypeORM Tutorial
- TypeORM - Home
- TypeORM - Introduction
- TypeORM - Installation
- TypeORM - Creating a Simple Project
- TypeORM - Connection API
- TypeORM - Entity
- TypeORM - Relations
- TypeORM - Working with Repository
- TypeORM - Working with Entity Manager
- TypeORM - Query Builder
- TypeORM - Query Operations
- TypeORM - Transactions
- TypeORM - Indices
- TypeORM - Entity Listener and Logging
- TypeORM with JavaScript
- TypeORM - Working with MongoDB
- TypeORM with Express
- TypeORM - Migrations
- TypeORM - Working with CLI
- TypeORM Useful Resources
- TypeORM - Quick Guide
- TypeORM - Useful Resources
- TypeORM - Discussion
TypeORM - Migrations
Migrations are like version control for your database. It is used to modify and share application’s database schema. This section explains about how migrations works in TypeORM.
Creating new migration
To create a new migration, first we need to setup connection in ormconfig.json. It is defined below −
ormconfig.json
"type": "mysql", "host": "localhost", "port": 8889, "username": "root", "password": "root", "database": "Library", "entities": ["entity/*.js"], "migrationsTableName": "student_migration_table", "migrations": ["migration/*.js"], "cli": { "migrationsDir": "migration" }
Here,
- migrationsTableName − it refers the migration table name.
- migrations − TypeORM loads migrations from given directory.
- cli − indicates migration will create inside the specific directory.
Create Book entity
Let’s create an entity named Book entity inside src/entity/Book.ts as follows −
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm'; @Entity() export class Book { @PrimaryGeneratedColumn() id: number; @Column() title: string; @Column() text: string; }
Execute CLI to create new migration
Now, we can execute new migration using CLI as follows −
Syntax
typeorm migration:create -n <migration-name>
Example
typeorm migration:create -n myMigration
After executing the above command, you could see the below response −
Migration /path/to/project/src/migration/1587101104904-myMigration.ts has been generated successfully.
Now, move inside src/migration/1587101104904-myMigration.ts file looks similar to this.
import {MigrationInterface, QueryRunner} from "typeorm"; export class myMigration1587101104904 implements MigrationInterface { public async up(queryRunner: QueryRunner): Promise<any> { } public async down(queryRunner: QueryRunner): Promise<any> { } }
Here,
We have two methods up and down. up method is used to add changes to the migration and down method is used to revert changes in your migration.
Let us add up method inside myMigration.ts file as specified below −
import {MigrationInterface, QueryRunner} from "typeorm"; export class Book1587131893261 implements MigrationInterface { public async up(queryRunner: QueryRunner): Promise<any> { await queryRunner.query(`ALTER TABLE book ADD COLUMN price int`); } public async down(queryRunner: QueryRunner): Promise<any> { } }
Here,
We have added a new column price inside book table. Now, execute the CLI to add the above changes.
ts-node ./node_modules/typeorm/cli.js migration:run
The above command executes migrations and run them in a sequence. Now, you could see the below changes in your screen −
Output

Now open your mysql server, new column is added. It is shown below −
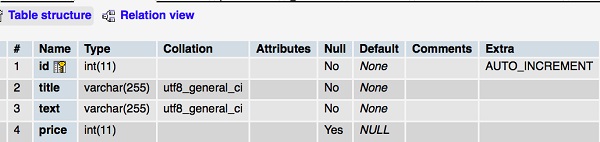
Similarly, We can modify column title datatype to varchar(30) as follows,
import {MigrationInterface, QueryRunner} from "typeorm"; export class Book1587131893261 implements MigrationInterface { public async up(queryRunner: QueryRunner): Promise<any> { await queryRunner.query(`ALTER TABLE book MODIFY COLUMN title varchar(30)`); } public async down(queryRunner: QueryRunner): Promise<any> { } }
Now, execute the same command and you could the below changes −
ts-node ./node_modules/typeorm/cli.js migration:run
Output

Book table is modified as,
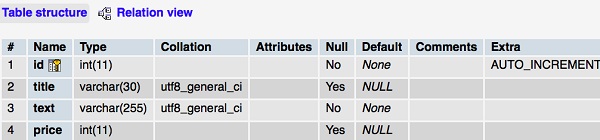
Revert migration
Let’s add the below code inside down method to revert migration −
import {MigrationInterface, QueryRunner} from "typeorm"; export class Book1587131893261 implements MigrationInterface { public async up(queryRunner: QueryRunner): Promise<any> { } public async down(queryRunner: QueryRunner): Promise<any> { await queryRunner.query(`ALTER TABLE book drop column price`); // reverts things made in "up" method } }
Now, execute the below command to revert all the changes −
ts-node ./node_modules/typeorm/cli.js migration:revert
You could see the following response −
Output

Book table is modified as,
Output

As we seen in this chapter, TypeORM makes it easy to write database migration script.