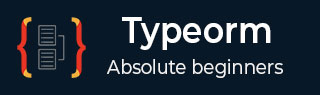
- TypeORM Tutorial
- TypeORM - Home
- TypeORM - Introduction
- TypeORM - Installation
- TypeORM - Creating a Simple Project
- TypeORM - Connection API
- TypeORM - Entity
- TypeORM - Relations
- TypeORM - Working with Repository
- TypeORM - Working with Entity Manager
- TypeORM - Query Builder
- TypeORM - Query Operations
- TypeORM - Transactions
- TypeORM - Indices
- TypeORM - Entity Listener and Logging
- TypeORM with JavaScript
- TypeORM - Working with MongoDB
- TypeORM with Express
- TypeORM - Migrations
- TypeORM - Working with CLI
- TypeORM Useful Resources
- TypeORM - Quick Guide
- TypeORM - Useful Resources
- TypeORM - Discussion
TypeORM - Indices
In general, Indexing is a process to optimize the performance of a database by optimizing the data storage. It is used to quickly locate and access the data in a database. This section explains about how to use index in TypeORM. Indices are classified into different types. Let’s go through one by one in detail.
Column indices
We can create index for particular column using @Index. Consider an example of Customer entity as shown below and index defined for firstName column,
import {Entity, PrimaryGeneratedColumn, Column} from "typeorm"; @Entity() export class Student { @PrimaryGeneratedColumn() id: number; @Index() @Column() firstName: string; @Column() lastName: string; @Column() age: number; @Column() address: string; }
@Index allow to specify name for an index as well −
@Index("Name-idx") @Column() firstName: string;
Unique indices
To specify Unique constraints in your column, use the below property −
{ unique: true }
For example, below is the code to specify unique index for Name column −
@Index({ unique: true }) @Column() firstName: string;
To apply indices for more than one columns, we can directly specify it after @Entity(). The sample code is as follows −
@Entity() @Index(["firstName", "lastName"]) @Index(["firstName", "lastName"], { unique: true })
Spatial indices
Spatial index allows to access spatial objects. MySQL and PostgreSQL supports spacial indices. To enable spatial indices in your column, add the following property −
{ spatial: true }
Spatial type has multiple sub types such as, geometry, point, line string, polygon etc., For example, if you want to add point spatial type in your column, use below code −
@Column("point") @Index({ spatial: true }) point: string;
Disable synchronization
To disable synchronization, use the below option on @Index decorator −
{ synchronize: false }