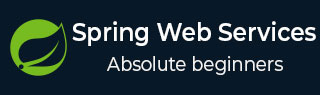
- Spring WS Tutorial
- Spring WS - Home
- Spring WS - Overview
- Spring WS - Environment Setup
- Spring WS - First Application
- Spring WS - Static WSDL
- Spring WS - Writing Server
- Spring WS - Unit Test Server
- Spring WS - Writing Client
- Spring WS - Unit Test Client
- Spring WS Useful Resources
- Spring WS - Quick Guide
- Spring WS - Useful Resources
- Spring WS - Discussion
Spring WS - Writing Server
In this chapter, we will understand how to create a web application server using Spring WS.
Step | Description |
---|---|
1 | Create a project with a name countryService under a package com.tutorialspoint as explained in the Spring WS - First Application chapter. |
2 | Create countries.xsd, domain classes, CountryRepository and CountryEndPoint as explained in the following steps. |
3 | Update spring-ws-servlet.xml under the /WEB-INF sub-folder. |
4 | The final step is to create content for all the source and configuration files and export the application as explained below. |
countries.xsd
<xs:schema xmlns:xs = "http://www.w3.org/2001/XMLSchema" xmlns:tns = "http://tutorialspoint/schemas" targetNamespace = "http://tutorialspoint/schemas" elementFormDefault = "qualified"> <xs:element name = "getCountryRequest"> <xs:complexType> <xs:sequence> <xs:element name = "name" type = "xs:string"/> </xs:sequence> </xs:complexType> </xs:element> <xs:element name = "getCountryResponse"> <xs:complexType> <xs:sequence> <xs:element name = "country" type = "tns:country"/> </xs:sequence> </xs:complexType> </xs:element> <xs:complexType name = "country"> <xs:sequence> <xs:element name = "name" type = "xs:string"/> <xs:element name = "population" type = "xs:int"/> <xs:element name = "capital" type = "xs:string"/> <xs:element name = "currency" type = "tns:currency"/> </xs:sequence> </xs:complexType> <xs:simpleType name = "currency"> <xs:restriction base = "xs:string"> <xs:enumeration value = "GBP"/> <xs:enumeration value = "USD"/> <xs:enumeration value = "INR"/> </xs:restriction> </xs:simpleType> </xs:schema>
Create the Project
Let us open the command console, go the C:\MVN directory and execute the following mvn command.
C:\MVN>mvn archetype:generate -DarchetypeGroupId = org.springframework.ws -DarchetypeArtifactId = spring-ws-archetype -DgroupId = com.tutorialspoint -DartifactId = countryService
Maven will start processing and will create the complete Java Application Project Structure.
[INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building Maven Stub Project (No POM) 1 [INFO] ------------------------------------------------------------------------ [INFO] [INFO] Using property: groupId = com.tutorialspoint [INFO] Using property: artifactId = countryService Define value for property 'version': 1.0-SNAPSHOT: : [INFO] Using property: package = com.tutorialspoint Confirm properties configuration: groupId: com.tutorialspoint artifactId: countryService version: 1.0-SNAPSHOT package: com.tutorialspoint Y: : [INFO] ------------------------------------------------------------------------- --- [INFO] Using following parameters for creating project from Old (1.x) Archetype: spring-ws-archetype:2.0.0-M1 [INFO] ------------------------------------------------------------------------- --- [INFO] Parameter: groupId, Value: com.tutorialspoint [INFO] Parameter: packageName, Value: com.tutorialspoint [INFO] Parameter: package, Value: com.tutorialspoint [INFO] Parameter: artifactId, Value: countryService [INFO] Parameter: basedir, Value: C:\mvn [INFO] Parameter: version, Value: 1.0-SNAPSHOT [INFO] project created from Old (1.x) Archetype in dir: C:\mvn\countryService [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 35.989 s [INFO] Finished at: 2017-01-21T11:18:31+05:30 [INFO] Final Memory: 17M/178M [INFO] ------------------------------------------------------------------------
Now go to C:/MVN directory. We will see a java application project created named countryService (as specified in artifactId). Update the pom.xml.
pom.xml
<?xml version = "1.0" encoding = "UTF-8"?> <project xmlns = "http://maven.apache.org/POM/4.0.0" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint.hr</groupId> <artifactId>countryService</artifactId> <packaging>war</packaging> <version>1.0-SNAPSHOT</version> <name>countryService Spring-WS Application</name> <url>http://www.springframework.org/spring-ws</url> <build> <finalName>countryService</finalName> </build> <dependencies> <dependency> <groupId>org.springframework.ws</groupId> <artifactId>spring-ws-core</artifactId> <version>2.4.0.RELEASE</version> </dependency> <dependency> <groupId>jdom</groupId> <artifactId>jdom</artifactId> <version>1.0</version> </dependency> <dependency> <groupId>jaxen</groupId> <artifactId>jaxen</artifactId> <version>1.1</version> </dependency> <dependency> <groupId>wsdl4j</groupId> <artifactId>wsdl4j</artifactId> <version>1.6.2</version> </dependency> </dependencies> </project>
Create Domain Classes
Copy the countries.xsd in C:\mvn\countryService\src\main\resources folder. Let us open the command console, go the C:\mvn\countryService\src\main\resources directory and execute the following xjc command to generate domain classes using the countries.xsd.
C:\MVN\countryService\src\main\resources>xjc -p com.tutorialspoint countries.xsd
Maven will start processing and will create the domain classes in com.tutorialspoint package.
parsing a schema... compiling a schema... com\tutorialspoint\Country.java com\tutorialspoint\Currency.java com\tutorialspoint\GetCountryRequest.java com\tutorialspoint\GetCountryResponse.java com\tutorialspoint\ObjectFactory.java com\tutorialspoint\package-info.java
Create folder java in C:\mvn\countryService\src\main folder. Copy all the classes in the C:\mvn\countryService\src\main\java folder. Create CountryRepository and CountryEndPoint to represent the country database and country server respectively.
CountryRepository.java
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; import org.springframework.beans.propertyeditors.CurrencyEditor; import org.springframework.stereotype.Component; import org.springframework.util.Assert; @Component public class CountryRepository { private static final List<Country> countries = new ArrayList<Country>(); public CountryRepository(){ initData(); } public void initData() { Country us = new Country(); us.setName("United States"); us.setCapital("Washington"); us.setCurrency(Currency.USD); us.setPopulation(46704314); countries.add(us); Country india = new Country(); india.setName("India"); india.setCapital("New Delhi"); india.setCurrency(Currency.INR); india.setPopulation(138186860); countries.add(india); Country uk = new Country(); uk.setName("United Kingdom"); uk.setCapital("London"); uk.setCurrency(Currency.GBP); uk.setPopulation(63705000); countries.add(uk); } public Country findCountry(String name) { Assert.notNull(name); Country result = null; for (Country country : countries) { if (name.trim().equals(country.getName())) { result = country; } } return result; } }
CountryEndPoint.java
package com.tutorialspoint.ws; import org.jdom.JDOMException; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.ws.server.endpoint.annotation.Endpoint; import org.springframework.ws.server.endpoint.annotation.PayloadRoot; import org.springframework.ws.server.endpoint.annotation.RequestPayload; import org.springframework.ws.server.endpoint.annotation.ResponsePayload; import com.tutorialspoint.Country; import com.tutorialspoint.CountryRepository; import com.tutorialspoint.GetCountryRequest; import com.tutorialspoint.GetCountryResponse; @Endpoint public class CountryEndPoint { private static final String NAMESPACE_URI = "http://tutorialspoint/schemas"; private CountryRepository countryRepository; @Autowired public CountryEndPoint(CountryRepository countryRepository) throws JDOMException { this.countryRepository = countryRepository; } @PayloadRoot(namespace = NAMESPACE_URI, localPart = "getCountryRequest") @ResponsePayload public GetCountryResponse getCountry(@RequestPayload GetCountryRequest request) throws JDOMException { Country country = countryRepository.findCountry(request.getName()); GetCountryResponse response = new GetCountryResponse(); response.setCountry(country); return response; } }
/WEB-INF/spring-ws-servlet.xml
<beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns:context = "http://www.springframework.org/schema/context" xmlns:sws = "http://www.springframework.org/schema/web-services" xsi:schemaLocation = "http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/web-services http://www.springframework.org/schema/web-services/web-services-2.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <context:component-scan base-package = "com.tutorialspoint"/> <sws:annotation-driven/> <sws:dynamic-wsdl id="countries" portTypeName = "CountriesPort" locationUri = "/countryService/" targetNamespace = "http://tutorialspoint.com/definitions"> <sws:xsd location = "/WEB-INF/countries.xsd"/> </sws:dynamic-wsdl> </beans>
/WEB-INF/web.xml
<web-app xmlns = "http://java.sun.com/xml/ns/j2ee" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd" version = "2.4"> <display-name>TutorialsPoint Country Service</display-name> <servlet> <servlet-name>spring-ws</servlet-name> <servlet-class>org.springframework.ws.transport.http.MessageDispatcherServlet </servlet-class> <init-param> <param-name>transformWsdlLocations</param-name> <param-value>true</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>spring-ws</servlet-name> <url-pattern>/*</url-pattern> </servlet-mapping> </web-app>
Build the Project
Let us open the command console. Go the C:\MVN\countryService directory and execute the following mvn command.
C:\MVN\countryService>mvn clean package
Maven will start building the project.
INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building countryService Spring-WS Application 1.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-clean-plugin:2.5:clean (default-clean) @ countryService --- [INFO] Deleting C:\mvn\countryService\target [INFO] [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ countrySer vice --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] Copying 0 resource [INFO] [INFO] --- maven-compiler-plugin:3.1:compile (default-compile) @ countryService --- [INFO] Changes detected - recompiling the module! [WARNING] File encoding has not been set, using platform encoding Cp1252, i.e. build is platform dependent! [INFO] Compiling 4 source files to C:\mvn\countryService\target\classes [INFO] [INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ co untryService --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\mvn\countryService\src\test\resour ces [INFO] [INFO] --- maven-compiler-plugin:3.1:testCompile (default-testCompile) @ country Service --- [INFO] No sources to compile [INFO] [INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ countryService --- [INFO] No tests to run. [INFO] [INFO] --- maven-war-plugin:2.2:war (default-war) @ countryService --- [INFO] Packaging webapp [INFO] Assembling webapp [countryService] in [C:\mvn\countryService\target\count ryService] [INFO] Processing war project [INFO] Copying webapp resources [C:\mvn\countryService\src\main\webapp] [INFO] Webapp assembled in [5137 msecs] [INFO] Building war: C:\mvn\countryService\target\countryService.war [INFO] WEB-INF\web.xml already added, skipping [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 16.484 s [INFO] Finished at: 2017-01-28T09:07:59+05:30 [INFO] Final Memory: 19M/170M [INFO] ------------------------------------------------------------------------
Run the Project
Once we have created the source and configuration files, export the countryService.war file in Tomcat's webapps folder.
Now, start the Tomcat server and ensure if we can access other webpages from the webapps folder using a standard browser. Make a POST request to the URL – http://localhost:8080/countryService/ and by using any SOAP client make the following request.
<x:Envelope xmlns:x = "http://schemas.xmlsoap.org/soap/envelope/" xmlns:tns = "http://tutorialspoint/schemas"> <x:Header/> <x:Body> <tns:getCountryRequest> <tns:name>United States</tns:name> </tns:getCountryRequest> </x:Body> </x:Envelope>
You will see the following result.
<SOAP-ENV:Envelope xmlns:SOAP-ENV = "http://schemas.xmlsoap.org/soap/envelope/"> <SOAP-ENV:Header/> <SOAP-ENV:Body> <ns2:getCountryResponse xmlns:ns2 = "http://tutorialspoint/schemas"> <ns2:country> <ns2:name>United States</ns2:name> <ns2:population>46704314</ns2:population> <ns2:capital>Washington</ns2:capital> <ns2:currency>USD</ns2:currency> </ns2:country> </ns2:getCountryResponse> </SOAP-ENV:Body> </SOAP-ENV:Envelope>