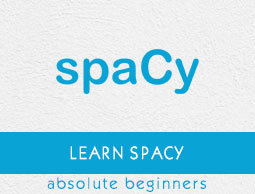
- spaCy Tutorial
- spaCy - Home
- spaCy - Introduction
- spaCy - Getting Started
- spaCy - Models and Languages
- spaCy - Architecture
- spaCy - Command Line Helpers
- spaCy - Top-level Functions
- spaCy - Visualization Function
- spaCy - Utility Functions
- spaCy - Compatibility Functions
- spaCy - Containers
- Doc Class ContextManager and Property
- spaCy - Container Token Class
- spaCy - Token Properties
- spaCy - Container Span Class
- spaCy - Span Class Properties
- spaCy - Container Lexeme Class
- Training Neural Network Model
- Updating Neural Network Model
- spaCy Useful Resources
- spaCy - Quick Guide
- spaCy - Useful Resources
- spaCy - Discussion
spaCy - Top-level Functions
Here, we will be discussing some of the top-level functions used in spaCy. The functions along with the descriptions are listed below −
Sr.No. | Command & Description |
---|---|
1 | spacy.load() To load a model. |
2 | spacy.blank() To create a blank model. |
3 | spacy.info() To provide information about the installation, models and local setup from within spaCy. |
4 | spacy.explain() To give a description. |
5 | spacy.prefer_gpu() To allocate data and perform operations on GPU. |
6 | spacy.require_gpu() To allocate data and perform operations on GPU. |
spacy.load()
As the name implies, this spacy function will load a model via following −
Its shortcut links.
The name of the installed model package.
A Unicode paths.
Path-like object.
spaCy will try to resolve the load argument in the below given order −
If a model is loaded from a shortcut link or package name, spaCy will assume it as a Python package and call the model’s own load() method.
On the other hand, if a model is loaded from a path, spacy will assume it is a data directory and hence initialize the Language class.
Upon using this function, the data will be loaded in via Language.from_disk.
Arguments
The table below explains its arguments −
NAME | TYPE | DESCRIPTION |
---|---|---|
name | unicode / Path | It is the shortcut link, package name or path of the model to load. |
disable | List | It represents the names of pipeline components to disable. |
Example
In the below examples, spacy.load() function loads a model by using shortcut link, package, unicode path and a pathlib path −
Following is the command for spacy.load() function for loading a model by using the shortcut link −
nlp_model = spacy.load("en")
Following is the command for spacy.load() function for loading a model by using package −
nlp_model = spacy.load("en_core_web_sm")
Following is the command for spacy.load() function for loading a model by using the Unicode path −
nlp_model = spacy.load("/path/to/en")
Following is the command for spacy.load() function for loading a model by using the pathlib path −
nlp_model = spacy.load(Path("/path/to/en"))
Following is the command for spacy.load() function for loading a model with all the arguments −
nlp_model = spacy.load("en_core_web_sm", disable=["parser", "tagger"])
spacy.blank()
It is the twin of spacy.blank() function, creates a blank model of a given language class.
Arguments
The table below explains its arguments −
NAME | TYPE | DESCRIPTION |
---|---|---|
name | unicode | It represents the ISO code of the language class to be loaded. |
disable | list | This argument represents the names of pipeline components to be disabled. |
Example
In the below examples, spacy.blank() function is used for creating a blank model of “en” language class.
nlp_model_en = spacy.blank("en")
spacy.info()
Like info command, spacy.info() function provides information about the installation, models, and local setup from within spaCy.
If you want to get the model meta data as a dictionary, you can use the meta-attribute on your nlp object with a loaded model. For example, nlp.meta.
Arguments
The table below explains its arguments −
NAME | TYPE | DESCRIPTION |
---|---|---|
model | unicode | It is the shortcut link, package name or path of a model. |
markdown | bool | This argument will print the information as Markdown. |
Example
An example is given below −
spacy.info() spacy.info("en") spacy.info("de", markdown=True)
spacy.explain()
This function will give us a description for the following −
POS tag
Dependency label
Entity type
Arguments
The table below explains its arguments −
NAME | TYPE | DESCRIPTION |
---|---|---|
term | unicode | It is the term which we want to be explained. |
Example
An example for the use of spacy.explain() function is mentioned below −
import spacy import en_core_web_sm nlp= en_core_web_sm.load() spacy.explain("NORP") doc = nlp("Hello TutorialsPoint") for word in doc: print(word.text, word.tag_, spacy.explain(word.tag_))
Output
Hello UH interjection TutorialsPoint NNP noun, proper singular
spacy.prefer_gpu()
If you have GPU, this function will allocate data and perform operations on GPU. But the data and operations will not be moved to GPU, if they are already available on CPU. It will return a Boolean output whether the GPU was activated or not.
Example
An example for the use of spacy.prefer_gpu() is stated below −
import spacy activated = spacy.prefer_gpu() nlp = spacy.load("en_core_web_sm")
spacy.require_gpu()
This function is introduced in version 2.0.14 and it will also allocate data and perform operations on GPU. It will raise an error if there is no GPU available. The data and operations will not be moved to GPU, if they are already available on CPU.
It is recommended that this function should be called right after importing spacy and before loading any of the model. It will also return a Boolean type output.
Example
An example for the use of spacy.require_gpu() function is as follows −
import spacy spacy.require_gpu() nlp = spacy.load("en_core_web_sm")