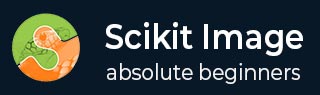
- Scikit Image Tutorial
- Scikit Image - Introduction
- Scikit Image - Image Processing
- Scikit Image - Numpy Images
- Scikit Image - Image datatypes
- Scikit Image - Using Plugins
- Scikit Image - Image Handling
- Scikit Image - Reading Images
- Scikit Image - Writing Images
- Scikit Image - Displaying Images
- Scikit Image - Image Collections
- Scikit Image - Image Stack
- Scikit Image - Multi Images
- Scikit Image - Data Visualization
- Scikit Image - Using Matplotlib
- Scikit Image - Using Plotly
- Scikit Image - Using Mayavi
- Scikit Image - Using Napari
Scikit Image - Using Mayavi
Mayavi is an application and library for interactive scientific data visualization and 3D plotting in Python. It provides a simple and clean scripting interface in Python for 3D visualization. It offers ready-to-use 3D visualization functionality similar to MATLAB or Matplotlib, especially when using the mlab module. This module provides a high-level interface that allows you to easily create various types of 3D plots and visualizations.
Mayavi also offers an object-oriented programming interface, allowing you to have more control and flexibility over your 3D visualizations. And it can work natively and transparently with numpy arrays, which makes it convenient to visualize scientific data stored in NumPy arrays without the need for data conversion or preprocessing.
Scikit Image with Mayavi
To use Mayavi as a plotting engine in your Python scripts, you can use the mlab scripting API, which provides a simple and convenient way to work with Mayavi and generate TVTK datasets using NumPy arrays or other sequences.
Installing Mayavi
To set up Mayavi and run the visualizations generated by the code, you need to install PyQt along with the Mayavi library. PyQt is a dependency that provides the necessary graphical user interface (GUI) functionality for displaying the visualizations created with Mayavi.
pip install mayavi pip install PyQt5
It is recommended to use pip, the Python Package Installer, for installing Python packages from the PyPI. This installs the latest version of Mayavi available on PyPI.
Once the required packages are installed successfully, you can import Mayavi into your Python scripts or interactive sessions using −
from mayavi import mlab
This imports the necessary modules from Mayavi for 3D visualization and scientific data plotting in your Python scripts.
Below are a few basic Python programs that demonstrate how to use the scikit-image along with Mayavi to perform data visualization in image processing tasks effectively.
Example 1
The following example demonstrates how to display an image using Mayavi's mlab.imshow() function.
from mayavi import mlab from skimage import io import numpy as np # Read an image image = np.random.random((10, 10)) # Display the masked image using Mayavi mlab.figure(fgcolor=(0, 0, 0), bgcolor=(1, 1, 1)) mlab.imshow(image) mlab.show()
Output
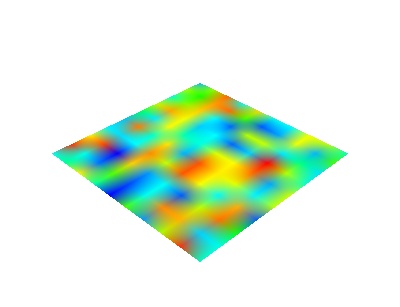
Example 2
Here is another example that demonstrates how to use Mayavi and scikit-image (skimage) together to display a grayscale image using Mayavi's visualization capabilities.
from mayavi import mlab from skimage import io # Read an image image = io.imread('Images/logo-w.png', as_gray=True) # Display the masked image using Mayavi mlab.figure(fgcolor=(0, 0, 0), bgcolor=(1, 1, 1)) mlab.imshow(image) mlab.show()
Output
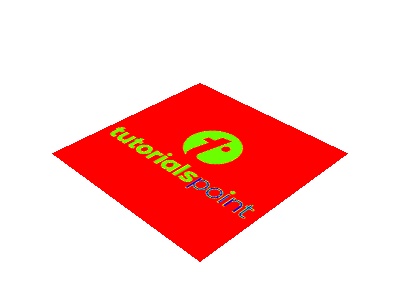