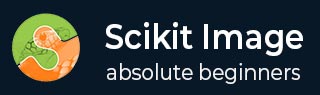
- Scikit Image Tutorial
- Scikit Image - Introduction
- Scikit Image - Image Processing
- Scikit Image - Numpy Images
- Scikit Image - Image datatypes
- Scikit Image - Using Plugins
- Scikit Image - Image Handling
- Scikit Image - Reading Images
- Scikit Image - Writing Images
- Scikit Image - Displaying Images
- Scikit Image - Image Collections
- Scikit Image - Image Stack
- Scikit Image - Multi Images
- Scikit Image - Data Visualization
- Scikit Image - Using Matplotlib
- Scikit Image - Using Plotly
- Scikit Image - Using Mayavi
- Scikit Image - Using Napari
Scikit Image - Multi Images
Multi Image or Multi-frame Image, in general, refers to an image format that can store and represent multiple images or frames within a single file. For instance, animated GIFs and multi-frame TIFF files are examples of multi-image formats.
MultiImage class in Scikit Image
The MultiImage class in the scikit-image io module is used to specifically handle multiframe TIFF images. It provides a convenient way to load and manipulate multi-frame TIFF images.
When working with multi-frame TIFFs using the MultiImage class, it returns a list of image-data arrays, similar to the ImageCollection class. However, there is a difference in how they handle multi-frame images. Multi-Image stores all frames of a multi-frame TIFF image as a single element in the list, with a shape of (N, W, H), where N is the number of frames and W and H are the width and height of each frame.
Following is the syntax of this class −
class skimage.io.MultiImage(filename, conserve_memory=True, dtype=None, **imread_kwargs)
Here are the parameters of the class −
- filename − A string or list of strings specifying the pattern or filenames to load. The path can be absolute or relative.
- conserve_memory (optional) − A boolean value. If set to True, only one image will be kept in memory at a time. If set to False, images will be cached after loading to improve subsequent access speed.
Example 1
The following example demonstrates how to use the MultiImage class to load a multiframe TIFF image and obtain information about the loaded image.
from skimage.io import MultiImage # Load the multi-frame TIFF image multi_image = MultiImage('Images_/Multi_Frame.tif') # Access and display information about the loaded image file print(multi_image) print('Type:',type(multi_image)) print('Length:',len(multi_image)) print('Shape:',multi_image[0].shape)
Output
['Images_/Multi_Frame.tif'] Type: < class 'skimage.io.collection.MultiImage' > Length: 1 Shape: (6, 382, 363, 3)
Example 2
Let's read the same Multi-frame TIFF file, "Multi_Frame.tif" using the ImageCollection class and observe how it treats the multi-frame images compared to the MultiImage class.
from skimage.io import ImageCollection # Load the multi-frame TIFF image ic = ImageCollection('Images_/Multi_Frame.tif') # Access and display information about the loaded image file print(ic) print('Type:',type(ic)) print('Length:',len(ic)) print('Shape:',ic[0].shape)
Output
['Images_/Multi_Frame.tif'] Type: < class 'skimage.io.collection.ImageCollection' > Length: 6 Shape: (382, 363, 3)
When working with an animated GIF image, MultiImage reads only the first frame, whereas the ImageCollection reads all frames by default.
Example 3
Let's look into the following example and observe how the MultiImage class treats the animated GIF image.
from skimage.io import MultiImage # Load an animated GIF image multi_image = MultiImage('Images/dance-cartoon.gif') # display the multi_image object print(multi_image) print('Type:',type(multi_image)) print('Length:',len(multi_image)) for i, frame in enumerate(multi_image): print('Image {} shape:{}'.format(i, frame.shape))
Output
['Images/dance-cartoon.gif'] Type: < class 'skimage.io.collection.MultiImage'> Length: 1 Image 0 shape:(300, 370, 4)
Example 4
Let's read the same GIF file, "dance-cartoon.gif" using the ImageCollection class and observe how it treats the animated GIF image compared to the MultiImage class.
from skimage.io import ImageCollection # Load an animated GIF image ic = ImageCollection('Images/dance-cartoon.gif') # Access and display information about the loaded image file print(ic) print('Type:',type(ic)) print('Length:',len(ic)) for i, frame in enumerate(ic): print('Image {} shape:{}'.format(i, frame.shape))
Input Image
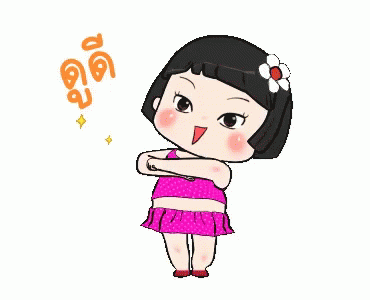
Output
['Images/dance-cartoon.gif'] Type: <class 'skimage.io.collection.ImageCollection'> Length: 12 Image 0 shape:(300, 370, 4) Image 1 shape:(300, 370, 4) Image 2 shape:(300, 370, 4) Image 3 shape:(300, 370, 4) Image 4 shape:(300, 370, 4) Image 5 shape:(300, 370, 4) Image 6 shape:(300, 370, 4) Image 7 shape:(300, 370, 4) Image 8 shape:(300, 370, 4) Image 9 shape:(300, 370, 4) Image 10 shape:(300, 370, 4) Image 11 shape:(300, 370, 4)