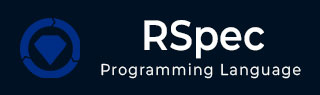
- RSpec Tutorial
- RSpec - Home
- RSpec - Introduction
- RSpec - Basic Syntax
- RSpec - Writing Specs
- RSpec - Matchers
- RSpec - Test Doubles
- RSpec - Stubs
- RSpec - Hooks
- RSpec - Tags
- RSpec - Subjects
- RSpec - Helpers
- RSpec - Metadata
- RSpec - Filtering
- RSpec - Expectations
- RSpec Resources
- RSpec - Quick Guide
- RSpec - Useful Resources
- RSpec - Discussion
RSpec - Hooks
When you are writing unit tests, it is often convenient to run setup and teardown code before and after your tests. Setup code is the code that configures or “sets up” conditions for a test. Teardown code does the cleanup, it makes sure that the environment is in a consistent state for subsequent tests.
Generally speaking, your tests should be independent of each other. When you run an entire suite of tests and one of them fails, you want to have confidence that it failed because the code that it is testing has a bug, not because the previous test left the environment in an inconsistent state.
The most common hooks used in RSpec are before and after hooks. They provide a way to define and run the setup and teardown code we discussed above. Let’s consider this example code −
class SimpleClass attr_accessor :message def initialize() puts "\nCreating a new instance of the SimpleClass class" @message = 'howdy' end def update_message(new_message) @message = new_message end end describe SimpleClass do before(:each) do @simple_class = SimpleClass.new end it 'should have an initial message' do expect(@simple_class).to_not be_nil @simple_class.message = 'Something else. . .' end it 'should be able to change its message' do @simple_class.update_message('a new message') expect(@simple_class.message).to_not be 'howdy' end end
When you run this code, you’ll get the following output −
Creating a new instance of the SimpleClass class . Creating a new instance of the SimpleClass class . Finished in 0.003 seconds (files took 0.11401 seconds to load) 2 examples, 0 failures
Let’s take a closer look at what’s happening. The before(:each) method is where we define the setup code. When you pass the :each argument, you are instructing the before method to run before each example in your Example Group i.e. the two it blocks inside the describe block in the code above.
In the line: @simple_class = SimpleClass.new, we are creating a new instance of the SimpleClass class and assigning it to an instance variable of an object. What object you might be wondering? RSpec creates a special class behind the scenes in the scope of the describe block. This allows you to assign values to instance variables of this class, that you can access within the it blocks in your Examples. This also makes it easy to write cleaner code in our tests. If each test (Example) needs an instance of SimpleClass, we can put that code in the before hook and not have to add it to each example.
Notice that, the line “Creating a new instance of the SimpleClass class” is written to the console twice, this shows that, before hook was called in each of the it blocks.
As we’ve mentioned, RSpec also has an after hook and both the before and after hooks can take: all as an argument. The after hook will run after the specified target. The: all target means that the hook will run before/after all of the Examples. Here is a simple example that illustrates when each hook is called.
describe "Before and after hooks" do before(:each) do puts "Runs before each Example" end after(:each) do puts "Runs after each Example" end before(:all) do puts "Runs before all Examples" end after(:all) do puts "Runs after all Examples" end it 'is the first Example in this spec file' do puts 'Running the first Example' end it 'is the second Example in this spec file' do puts 'Running the second Example' end end
When you run the above code, you will see this output −
Runs before all Examples Runs before each Example Running the first Example Runs after each Example .Runs before each Example Running the second Example Runs after each Example .Runs after all Examples