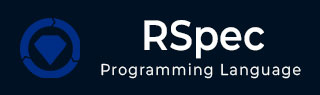
- RSpec Tutorial
- RSpec - Home
- RSpec - Introduction
- RSpec - Basic Syntax
- RSpec - Writing Specs
- RSpec - Matchers
- RSpec - Test Doubles
- RSpec - Stubs
- RSpec - Hooks
- RSpec - Tags
- RSpec - Subjects
- RSpec - Helpers
- RSpec - Metadata
- RSpec - Filtering
- RSpec - Expectations
- RSpec Resources
- RSpec - Quick Guide
- RSpec - Useful Resources
- RSpec - Discussion
RSpec - Expectations
When you learn RSpec, you may read a lot about expectations and it can be a bit confusing at first. There are two main details you should keep in mind when you see the term Expectation −
An Expectation is simply a statement in an it block that uses the expect() method. That’s it. It’s no more complicated than that. When you have code like this: expect(1 + 1).to eq(2), you have an Expectation in your example. You are expecting that the expression 1 + 1 evaluates to 2. The wording is important though since RSpec is a BDD test framework. By calling this statement an Expectation, it is clear that your RSpec code is describing the “behavior” of the code it’s testing. The idea is that you are expressing how the code should behave, in a way that reads like documentation.
The Expectation syntax is relatively new. Before the expect() method was introduced (back in 2012), RSpec used a different syntax that was based on the should() method. The above Expectation is written like this in the old syntax: (1 + 1).should eq(2).
You may encounter the old RSpec syntax for Expectations when working with an older code based or an older version of RSpec. If you use the old syntax with a new version of RSpec, you will see a warning.
For example, with this code −
RSpec.describe "An RSpec file that uses the old syntax" do it 'you should see a warning when you run this Example' do (1 + 1).should eq(2) end end
When you run it, you will get an output that looks like this −
. Deprecation Warnings: Using `should` from rspec-expectations' old `:should` syntax without explicitly enabling the syntax is deprecated. Use the new `:expect` syntax or explicitly enable `:should` with `config.expect_with( :rspec) { |c| c.syntax = :should }` instead. Called from C:/rspec_tutorial/spec/old_expectation.rb:3 :in `block (2 levels) in <top (required)>'. If you need more of the backtrace for any of these deprecations to identify where to make the necessary changes, you can configure `config.raise_errors_for_deprecations!`, and it will turn the deprecation warnings into errors, giving you the full backtrace. 1 deprecation warning total Finished in 0.001 seconds (files took 0.11201 seconds to load) 1 example, 0 failures
Unless you are required to use the old syntax, it is highly recommended that you use expect() instead of should().